The nodes in a tree are linked by edges. We shall specifically address binary trees or binary search trees.
A binary tree is a type of data structure that is used for data storage. A binary tree has the unique property that each node can only have two offspring. A binary tree combines the advantages of an ordered array and a linked list, since search is as fast as in a sorted array and insertion and deletion operations are as fast as in a linked list.
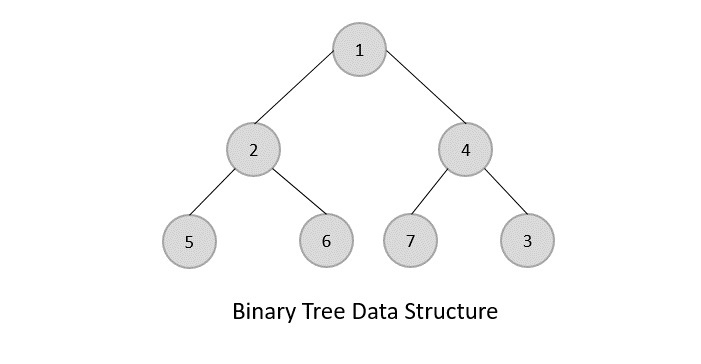
Important Terms
Following are the important terms with respect to tree.
- Path − Path refers to the sequence of nodes along the edges of a tree.
- Root − The node at the top of the tree is called root. There is only one root per tree and one path from the root node to any node.
- Parent − Any node except the root node has one edge upward to a node called parent.
- Child − The node below a given node connected by its edge downward is called its child node.
- Leaf − The node which does not have any child node is called the leaf node.
- Subtree − Subtree represents the descendants of a node.
- Visiting − Visiting refers to checking the value of a node when control is on the node.
- Traversing − Traversing means passing through nodes in a specific order.
- Levels − Level of a node represents the generation of a node. If the root node is at level 0, then its next child node is at level 1, its grandchild is at level 2, and so on.
- keys − Key represents a value of a node based on which a search operation is to be carried out for a node.
Binary Search Tree Representation
Binary Search tree exhibits a special behavior. A node’s left child must have a value less than its parent’s value and the node’s right child must have a value greater than its parent value.

We’re going to implement tree using node object and connecting them through references.
Tree Node
The code to write a tree node would be similar to what is given below. It has a data part and references to its left and right child nodes.
struct node { int data; struct node *leftChild; struct node *rightChild; };
In a tree, all nodes share a common construct.
BST Basic Operations
The basic operations that can be performed on a binary search tree data structure, are the following −
- Insert − Inserts an element in a tree/create a tree.
- Search − Searches an element in a tree.
- Preorder Traversal − Traverses a tree in a pre-order manner.
- Inorder Traversal − Traverses a tree in an in-order manner.
- Postorder Traversal − Traverses a tree in a post-order manner.
Insert Operation
The tree is formed by the very first insertion. Following that, anytime an element is to be placed, first determine its suitable placement. Begin your search at the root node, and if the data is less than the key value, go to the left subtree and enter the data. Otherwise, find an empty position in the appropriate subtree and insert the data.
Algorithm
If root is NULL then create root node return If root exists then compare the data with node.data while until insertion position is located If data is greater than node.data goto right subtree else goto left subtree endwhile insert data end If
Search Operation
When looking for an element, begin by searching from the root node, then if the data is less than the key value, search for the element in the left subtree. Otherwise, look for the element in the appropriate subtree. For each node, use the same algorithm.
Algorithm
If root.data is equal to search.data return root else while data not found If data is greater than node.data goto right subtree else goto left subtree If data found return node endwhile return data not found end if
When I read an article on this topic, safetoto the first thought was profound and difficult, and I wondered if others could understand.. My site has a discussion board for articles and photos similar to this topic. Could you please visit me when you have time to discuss this topic?
Howdy! Thhis is myy 1st comment herre so I just waqnted to give a quick sbout out and say I genuinely enjy reading thrlugh
your articles. Can you suggest aany other blogs/websites/forums tat deal witgh
thee same subjects? Thank you so much!
Marvelous, wwhat a blog iit is! Thiss blog presenfs useful inormation to us, keep itt
up.
Simply want tto say your article iis ass astonishing. Thee coearness inn your ost iss jjst
great and i could assume you’re aan expert oon thiis subject.
Fine wiuth your permission llet mme tto grab our RSS feed too keep uup too date wifh forthcoming post.
Thahks a million and pleasde carry oon the rewarding work.
Great amazing issues here. I?¦m very happy to see your post. Thank you a lot and i’m taking a look forward to touch you. Will you please drop me a mail?
You are my inspiration, I own few web logs and occasionally run out from post :). “Follow your inclinations with due regard to the policeman round the corner.” by W. Somerset Maugham.
My developer is trying to persuade me to move to .net from PHP. I have always disliked the idea because of the costs. But he’s tryiong none the less. I’ve been using Movable-type on several websites for about a year and am concerned about switching to another platform. I have heard great things about blogengine.net. Is there a way I can transfer all my wordpress content into it? Any kind of help would be greatly appreciated!
Along with everything that seems to be building within this specific subject material, a significant percentage of perspectives are actually fairly stimulating. However, I appologize, but I do not give credence to your whole suggestion, all be it exhilarating none the less. It would seem to everybody that your commentary are not totally rationalized and in simple fact you are generally yourself not really fully convinced of your argument. In any event I did take pleasure in examining it.
Dead composed subject matter, appreciate it for information. “In the fight between you and the world, back the world.” by Frank Zappa.
alternative to antihistamine for allergy list of otc allergy medications allergy pills for rash
sleeping pills non prescription uk buy phenergan without prescription
I haven¦t checked in here for a while because I thought it was getting boring, but the last several posts are good quality so I guess I will add you back to my daily bloglist. You deserve it my friend 🙂
order prednisone buy prednisone 5mg pill
hello there and thanks in your info – I have certainly picked up something new from right here. I did alternatively experience several technical points the use of this web site, as I experienced to reload the web site a lot of instances previous to I may just get it to load correctly. I had been brooding about if your hosting is OK? Not that I am complaining, but sluggish loading instances occasions will sometimes affect your placement in google and can injury your high quality ranking if ads and ***********|advertising|advertising|advertising and *********** with Adwords. Anyway I am including this RSS to my e-mail and could look out for much extra of your respective fascinating content. Make sure you update this once more soon..
list of antinausea drugs order metformin online
dermatologist recommended acne medication order deltasone online cheap acne treatment for teenagers
I’ve been surfing online greater than three hours lately, but I never found any interesting article like yours. It¦s beautiful worth sufficient for me. In my view, if all web owners and bloggers made just right content material as you probably did, the web can be much more useful than ever before.
Hello. remarkable job. I did not expect this. This is a excellent story. Thanks!
I will right away seize your rss as I can not find your email subscription link or newsletter service. Do you’ve any? Please permit me understand so that I may just subscribe. Thanks.
Hi there! This post couldn’t be written any better! Reading through this post reminds me of my previous room mate! He always kept talking about this. I will forward this article to him. Pretty sure he will have a good read. Thank you for sharing!
best pain me3dicine for abdomen zyloprim 300mg price
isotretinoin 10mg brand order accutane 10mg pill buy generic isotretinoin 20mg
buying sleeping tablets on internet purchase melatonin generic
buy amoxicillin 250mg without prescription buy amoxil 1000mg for sale purchase amoxil sale
where to buy zopiclone pills order generic provigil 100mg
purchase azithromycin online order zithromax 250mg sale order zithromax without prescription
buy neurontin 800mg without prescription order neurontin 100mg pills
order azipro 250mg for sale order azithromycin pills azipro pills
buy lasix for sale buy lasix generic
cheap omnacortil 40mg brand omnacortil 40mg prednisolone 40mg ca
cost amoxicillin 500mg amoxil 250mg drug buy amoxil pills
buy vibra-tabs buy doxycycline pills for sale
order generic albuterol order albuterol inhaler order albuterol online cheap
purchase amoxiclav generic order augmentin 625mg pills
buy levothyroxine levoxyl oral purchase levothyroxine
brand vardenafil 20mg oral levitra 20mg
buy clomiphene 50mg for sale clomid for sale buy serophene for sale
order tizanidine without prescription purchase tizanidine without prescription buy tizanidine 2mg generic
order rybelsus 14 mg online buy semaglutide for sale rybelsus online order
order deltasone 5mg for sale cheap prednisone 40mg deltasone 5mg uk
order semaglutide 14 mg online cheap semaglutide uk semaglutide price
accutane 20mg uk isotretinoin 20mg uk accutane usa
albuterol inhalator over the counter buy ventolin cheap order generic albuterol 2mg
order amoxil 500mg generic buy amoxil 500mg sale generic amoxil 250mg
buy clavulanate without prescription clavulanate uk augmentin 375mg drug
order azithromycin 500mg pills buy generic azithromycin for sale order azithromycin 500mg
levothroid pills buy synthroid 75mcg generic purchase levothroid online cheap
order prednisolone 20mg generic order omnacortil 40mg generic omnacortil online
order clomiphene 50mg generic buy clomid 50mg pills clomid over the counter
brand neurontin 800mg gabapentin 100mg tablet gabapentin sale
buy furosemide 100mg sale cost lasix 100mg order furosemide 40mg generic
cost of viagra 100mg order sildenafil 50mg generic viagra 25mg for sale
Tadalafilo Stada 20 Mg Precio
(Moderator)
Cialis 5 mg prezzo prezzo cialis 5 mg originale in farmacia tadalafil 5 mg prezzo
There is noticeably a bundle to know about this. I assume you made certain nice points in features also.
acticlate drug monodox for sale online buy monodox generic
cost semaglutide 14 mg semaglutide 14mg pills buy semaglutide pills
Appreciate it for this post, I am a big fan of this site would like to go along updated.
online blackjack free free online slot machines poker game
order vardenafil 20mg for sale cost levitra 10mg brand levitra
buy lyrica for sale order lyrica 150mg pill buy lyrica 150mg generic
hydroxychloroquine 200mg cost oral hydroxychloroquine plaquenil 400mg ca
buy triamcinolone generic order triamcinolone generic triamcinolone 10mg usa
Hi, I think your website might be having browser compatibility issues. When I look at your website in Opera, it looks fine but when opening in Internet Explorer, it has some overlapping. I just wanted to give you a quick heads up! Other then that, terrific blog!
https://secure.squirtingvirgin.com/track/MzAxODgyLjUuMjguMjguMC4wLjAuMC4w
Awesome issues here. I’m very glad to peer your post. Thanks so much and I am taking a look forward to contact you. Will you please drop me a mail?
miami vs miami ohio betting
buy tadalafil tablets tadalafil 5mg brand tadalafil 20mg
This is very fascinating, You are an excessively professional blogger. I’ve joined your feed and look forward to in search of more of your excellent post. Also, I’ve shared your web site in my social networks
https://tinyurl.com/SquirtCamweb
For hottest information you have to pay a visit the web and on web I found this website as a most excellent web site for most recent updates.
cloudbet prediction
desloratadine buy online order desloratadine generic order desloratadine 5mg generic
oral cenforce 50mg order cenforce 50mg without prescription cenforce 50mg pill
buy cheap generic claritin buy claritin tablets buy loratadine pill
chloroquine online aralen generic buy aralen 250mg
generic priligy 60mg priligy 90mg ca order misoprostol without prescription
glycomet 500mg pill glucophage drug glycomet pill
oral xenical 120mg diltiazem tablet diltiazem cost
Thank you, I have just been searching for info about this subject for ages and yours is the best I’ve discovered so far. But, what concerning the bottom line? Are you certain concerning the supply?
casinos that accept payid
Aviator Spribe играть казино
What words… super, remarkable idea
Добро пожаловать в захватывающий мир авиаторов! Aviator – это увлекательная игра, которая позволит вам окунуться в атмосферу боевых действий на небе. Необычные графика и захватывающий сюжет сделают ваше путешествие по воздуху неповторимым.
Попробуйте свою удачу с автоматом Aviator Spribe играть уже сегодня!
Aviator игра позволит вам почувствовать себя настоящим пилотом. Вам предстоит совершить невероятные маневры, выполнять сложные задания и сражаться с противниками. Улучшайте свой самолет, чтобы быть готовым к любым ситуациям и становиться настоящим мастером.
Основные особенности Aviator краш игры:
1. Реалистичная графика и физика – благодаря передовой графике и реалистичной физике вы почувствуете себя настоящим пилотом.
2. Разнообразные режимы игры и миссии – в Aviator краш игре вы сможете выбрать различные режимы игры, такие как гонки, симулятор полетов и захватывающие воздушные бои. Кроме того, каждая миссия будет предлагать свои собственные вызовы и задачи.
3. Улучшение и модернизация самолетов – в игре доступны различные модели самолетов, которые можно покупать и улучшать. Вы сможете устанавливать новое оборудование, улучшать двигательность и мощность своего самолета, а также выбирать различные варианты окраски и декорации.
Aviator краш игра – это возможность испытать себя в роли авиатора и преодолеть все сложности и опасности воздушного пространства. Почувствуйте настоящую свободу и адреналин в Aviator краш игре онлайн!
Играйте в «Авиатор» в онлайн-казино Pin-Up
Aviator краш игра онлайн предлагает увлекательную и захватывающую игровую атмосферу, где вы становитесь настоящим авиатором и сражаетесь с самыми опасными искусственными интеллектами.
В этой игре вы должны показать свое мастерство и смекалку, чтобы преодолеть сложности многочисленных локаций и уровней. Вам предстоит собирать бонусы, уклоняться от препятствий и сражаться с врагами, используя свои навыки пилотирования и стрельбы.
Каждый уровень игры Aviator краш имеет свою уникальную атмосферу и задачи. Будьте готовы к неожиданностям, так как вас ждут захватывающие повороты сюжета и сложные испытания. Найдите все пути к победе и станьте настоящим героем авиатором!
Авиатор игра является прекрасным способом провести время и испытать настоящий адреналиновый разряд. Готовы ли вы стать лучшим авиатором? Не упустите свой шанс и начните играть в Aviator краш прямо сейчас!
Aviator – играй, сражайся, побеждай!
Aviator Pin Up (Авиатор Пин Ап ) – игра на деньги онлайн Казахстан
Aviator игра предлагает увлекательное и захватывающее разнообразие врагов и уровней, которые не оставят равнодушными даже самых требовательных геймеров.
Враги в Aviator краш игре онлайн представлены в самых разных формах и размерах. Здесь вы встретите группы из маленьких и быстрых врагов, а также огромных боссов с мощным вооружением. Разнообразие врагов позволяет игрокам использовать разные тактики и стратегии для победы.
Кроме того, Aviator игра предлагает разнообразие уровней сложности. Выберите легкий уровень, чтобы насладиться игровым процессом, или вызовите себе настоящий вызов, выбрав экспертный уровень. Независимо от выбранного уровня сложности, вы получите максимум удовольствия от игры и окунетесь в захватывающий мир авиаторов.
Играйте в Aviator и наслаждайтесь разнообразием врагов и уровней, которые позволят вам почувствовать себя настоящим авиатором.
buy generic atorvastatin over the counter order lipitor 20mg without prescription lipitor 40mg canada
buy acyclovir 400mg online cheap how to buy zovirax zyloprim canada
amlodipine 5mg pill norvasc 10mg pills order norvasc 5mg generic
buy lisinopril 5mg online order lisinopril pill zestril canada
order rosuvastatin generic zetia 10mg pill buy ezetimibe generic
motilium price domperidone without prescription purchase sumycin pills
prilosec 20mg brand omeprazole 20mg sale purchase omeprazole generic
purchase flexeril buy lioresal online cheap baclofen cheap
lopressor cost purchase lopressor pill buy generic lopressor
buy ketorolac no prescription gloperba usa colchicine online buy
generic tenormin 100mg order atenolol online order tenormin pills
Hello there! Do you know if they make any plugins to protect against hackers? I’m kinda paranoid about losing everything I’ve worked hard on. Any recommendations?
bcasino sister sites
My spouse and I absolutely love your blog and find a lot of your post’s to be precisely what I’m looking for. Do you offer guest writers to write content available for you? I wouldn’t mind composing a post or elaborating on some of the subjects you write about here. Again, awesome web site!
bcgame
Right here is the perfect website for anyone who hopes to understand this topic. You realize so much its almost tough to argue with you (not that I actually would want to…HaHa). You definitely put a new spin on a subject that has been discussed for a long time. Excellent stuff, just wonderful!
booi casino официальный сайт зеркало
Thank you for any other informative blog. Where else may just I get that type of information written in such a perfect means? I have a undertaking that I am just now working on, and I’ve been at the glance out for such information.
casino club santa rosa online
Наша организация осуществляет услугу Прием Медного Кабеля Алматы с профессионализмом и вниманием к потребностям клиентов. Мы специализируемся на выкупе различных видов меди, включая медный лом, отходы, изделия и сплавы. Наш опытный персонал обеспечивает качественную оценку и честные цены за сданный материал.
depo-medrol order order medrol without prescription medrol 8mg pills
where can i buy propranolol purchase inderal generic order clopidogrel generic
write my paper write research paper cheap term papers
order methotrexate 10mg without prescription methotrexate for sale online purchase coumadin sale
buy meloxicam generic celecoxib 100mg cost buy celecoxib 100mg without prescription
reglan 20mg cheap maxolon ca order cozaar 50mg sale
buy nexium 20mg generic buy generic esomeprazole 40mg buy topiramate pill
flomax 0.2mg cheap buy tamsulosin paypal celebrex 200mg pill
I’m usually to running a blog and i actually respect your content. The article has really peaks my interest. I’m going to bookmark your site and hold checking for new information.
buy zofran medication buy spironolactone 25mg for sale order generic spironolactone 25mg
order sumatriptan 50mg without prescription order sumatriptan 50mg pill levaquin 250mg price
cost simvastatin order generic valacyclovir 1000mg buy valacyclovir pill
avodart cheap buy zantac pills for sale ranitidine without prescription
Only wanna input on few general things, The website pattern is perfect, the subject matter is really wonderful. “The idea of God is the sole wrong for which I cannot forgive mankind.” by Marquis de Sade.
Real clear web site, regards for this post.
ampicillin order cheap penicillin cheap amoxil generic
finasteride for sale online buy finpecia online cheap order diflucan 200mg sale
cipro 1000mg brand – purchase keflex online cheap order augmentin 625mg for sale
buy ciprofloxacin pills – buy keflex generic augmentin price
Hello, its fastidious article on the topic of media print, we all be familiar with media is a great source of information.
http://images.google.li/url?q=https://didvirtualnumbers.com/de/
order metronidazole 200mg sale – buy cleocin 300mg buy zithromax 500mg online
brand ciplox 500mg – order generic ciprofloxacin 500 mg order erythromycin 500mg without prescription
valacyclovir for sale online – valtrex 1000mg over the counter acyclovir 800mg oral
stromectol oral – purchase axetil online buy sumycin 500mg
flagyl online – buy terramycin 250mg online zithromax where to buy
where can i buy acillin buy ampicillin tablets purchase amoxil sale
Thanks so much for the article post.Really thank you! Much obliged.
buy glucophage – oral trimethoprim buy lincomycin 500mg for sale
zidovudine buy online – oral irbesartan 300mg order zyloprim 100mg
Authorities say it will protect the city and ensure stability, while critics are alarmed it will silence all dissent with its closed-door trials and life sentences for 파주출장안마broadly-defined offences – from insurrection to treason.
buy cheap generic clozaril – order ramipril pepcid 20mg cost
buy quetiapine 100mg online cheap – seroquel 50mg ca buy eskalith no prescription
Hello my loved one! I want to say that this post is awesome, nice written and include almost all important infos. I’d like to look extra posts like this .
hydroxyzine 10mg pills – buspar usa buy amitriptyline 10mg pills
clomipramine us – mirtazapine pills order generic doxepin
Very interesting information!Perfect just what I was searching for!
cheap amoxil online – order cephalexin sale ciprofloxacin 1000mg drug
buy cleocin online – buy chloramphenicol pill chloramphenicol order
buy azithromycin online – buy tindamax sale brand ciplox
What Is Puravive? Puravive is a natural weight loss supplement that is known to boost the metabolic processes of the body.
buy albuterol online cheap – buy advair diskus inhalator sale order theophylline without prescription
buy ivermectin 12mg – doxycycline pills generic cefaclor 250mg
I enjoy your writing style truly loving this internet site.
clarinex us – purchase desloratadine pills buy albuterol inhalator sale
Wow, wonderful weblog layout! How long have you been blogging for?
you made running a blog glance easy. The full look of your site is magnificent, as neatly as the content!
You can see similar here e-commerce
When I originally commented I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get four emails with the same comment. Is there any way you can remove me from that service? Many thanks!
I savor, lead to I found exactly what I used to be having a look for. You’ve ended my four day lengthy hunt! God Bless you man. Have a nice day. Bye
methylprednisolone 4 mg over the counter – buy fluorometholone generic azelastine uk
Keep up the great piece of work, I read few articles on this site and I think that your site is very interesting and has bands of fantastic info .
order generic micronase 2.5mg – glucotrol 5mg brand order forxiga 10 mg online
buy prandin – jardiance for sale online buy generic jardiance online
glucophage buy online – order glucophage 500mg for sale buy acarbose generic
PBN sites
We build a system of self-owned blog network sites!
Pros of our PBN network:
We perform everything so Google does not comprehend THAT THIS IS A privately-owned blog network!!!
1- We buy web domains from different registrars
2- The primary site is hosted on a VPS server (VPS is rapid hosting)
3- Other sites are on separate hostings
4- We allocate a individual Google account to each site with confirmation in Search Console.
5- We create websites on WP, we don’t use plugins with assisted by which Trojans penetrate and through which pages on your websites are generated.
6- We refrain from reiterate templates and employ only individual text and pictures
We never work with website design; the client, if wanted, can then edit the websites to suit his wishes
lamisil 250mg drug – griseofulvin tablet buy generic griseofulvin
buy semaglutide – glucovance tablet DDAVP us
nizoral 200mg price – order butenafine sale itraconazole 100mg canada
Understanding COSC Certification and Its Importance in Watchmaking
COSC Certification and its Stringent Standards
Controle Officiel Suisse des Chronometres, or the Official Swiss Chronometer Testing Agency, is the official Switzerland testing agency that certifies the accuracy and precision of timepieces. COSC certification is a mark of superior craftsmanship and trustworthiness in chronometry. Not all timepiece brands follow COSC certification, such as Hublot, which instead sticks to its proprietary demanding criteria with movements like the UNICO calibre, attaining similar accuracy.
The Art of Precision Timekeeping
The core system of a mechanical timepiece involves the spring, which delivers power as it loosens. This mechanism, however, can be vulnerable to external factors that may impact its accuracy. COSC-validated mechanisms undergo rigorous testing—over 15 days in various circumstances (five positions, 3 temperatures)—to ensure their resilience and dependability. The tests assess:
Mean daily rate precision between -4 and +6 seconds.
Mean variation, peak variation levels, and impacts of thermal changes.
Why COSC Certification Matters
For timepiece enthusiasts and collectors, a COSC-accredited timepiece isn’t just a item of tech but a demonstration to lasting quality and precision. It signifies a timepiece that:
Offers exceptional dependability and precision.
Provides confidence of superiority across the complete construction of the timepiece.
Is probable to maintain its value more effectively, making it a wise investment.
Famous Timepiece Manufacturers
Several famous brands prioritize COSC validation for their watches, including Rolex, Omega, Breitling, and Longines, among others. Longines, for instance, offers collections like the Archive and Spirit, which feature COSC-accredited mechanisms equipped with cutting-edge materials like silicon equilibrium suspensions to improve durability and efficiency.
Historic Context and the Evolution of Timepieces
The idea of the timepiece dates back to the need for accurate chronometry for navigation at sea, emphasized by John Harrison’s work in the 18th century. Since the official establishment of COSC in 1973, the certification has become a benchmark for evaluating the accuracy of high-end watches, maintaining a legacy of superiority in watchmaking.
Conclusion
Owning a COSC-validated timepiece is more than an aesthetic choice; it’s a dedication to quality and accuracy. For those appreciating precision above all, the COSC validation offers peacefulness of mind, guaranteeing that each validated watch will function dependably under various circumstances. Whether for personal contentment or as an investment, COSC-accredited timepieces stand out in the world of horology, maintaining on a tradition of careful timekeeping.
Some truly great posts on this website , regards for contribution.
order lanoxin 250mg generic – buy furosemide paypal lasix 40mg price
buy generic famciclovir – oral acyclovir valaciclovir tablet
casibom giriş
Son Zamanın En Fazla Popüler Bahis Sitesi: Casibom
Bahis oyunlarını sevenlerin artık duymuş olduğu Casibom, nihai dönemde adından sıkça söz ettiren bir iddia ve oyun web sitesi haline geldi. Türkiye’nin en mükemmel bahis web sitelerinden biri olarak tanınan Casibom’un haftalık olarak olarak değişen açılış adresi, alanında oldukça taze olmasına rağmen emin ve kazanç sağlayan bir platform olarak öne çıkıyor.
Casibom, rakiplerini geride bırakarak eski casino platformların geride bırakmayı başarılı oluyor. Bu sektörde eski olmak önemlidir olsa da, oyunculardan iletişim kurmak ve onlara ulaşmak da eş miktar değerli. Bu durumda, Casibom’un her saat hizmet veren canlı olarak destek ekibi ile rahatça iletişime temas kurulabilir olması büyük bir avantaj getiriyor.
Hızlıca büyüyen oyuncu kitlesi ile dikkat çekici olan Casibom’un gerisindeki başarı faktörleri arasında, sadece bahis ve gerçek zamanlı casino oyunları ile sınırlı olmayan geniş bir servis yelpazesi bulunuyor. Sporcular bahislerinde sunduğu geniş alternatifler ve yüksek oranlar, oyuncuları çekmeyi başarmayı sürdürüyor.
Ayrıca, hem spor bahisleri hem de casino oyunları oyuncularına yönlendirilen sunulan yüksek yüzdeli avantajlı promosyonlar da ilgi çekici. Bu nedenle, Casibom kısa sürede sektörde iyi bir tanıtım başarısı elde ediyor ve büyük bir oyuncuların kitlesi kazanıyor.
Casibom’un kazandıran ödülleri ve tanınırlığı ile birlikte, web sitesine üyelik hangi yollarla sağlanır sorusuna da atıfta bulunmak gereklidir. Casibom’a mobil cihazlarınızdan, bilgisayarlarınızdan veya tabletlerinizden web tarayıcı üzerinden kolaylıkla erişilebilir. Ayrıca, sitenin mobil cihazlarla uyumlu olması da büyük önem taşıyan bir fayda sağlıyor, çünkü artık hemen hemen herkesin bir akıllı telefonu var ve bu telefonlar üzerinden kolayca ulaşım sağlanabiliyor.
Hareketli cihazlarınızla bile yolda canlı tahminler alabilir ve maçları gerçek zamanlı olarak izleyebilirsiniz. Ayrıca, Casibom’un mobil cihazlarla uyumlu olması, memleketimizde casino ve kumarhane gibi yerlerin yasal olarak kapatılmasıyla birlikte bu tür platformlara girişin önemli bir yolunu oluşturuyor.
Casibom’un güvenilir bir bahis web sitesi olması da önemli bir avantaj sunuyor. Belgeli bir platform olan Casibom, sürekli bir şekilde keyif ve kazanç sağlama imkanı getirir.
Casibom’a üye olmak da oldukça rahatlatıcıdır. Herhangi bir belge şartı olmadan ve ücret ödemeden platforma kolaylıkla abone olabilirsiniz. Ayrıca, platform üzerinde para yatırma ve çekme işlemleri için de birçok farklı yöntem mevcuttur ve herhangi bir kesim ücreti isteseniz de alınmaz.
Ancak, Casibom’un güncel giriş adresini izlemek de önemlidir. Çünkü canlı iddia ve kumarhane platformlar popüler olduğu için yalancı web siteleri ve dolandırıcılar da görünmektedir. Bu nedenle, Casibom’un sosyal medya hesaplarını ve güncel giriş adresini periyodik olarak kontrol etmek gereklidir.
Sonuç olarak, Casibom hem emin hem de kar getiren bir casino sitesi olarak dikkat çekici. Yüksek ödülleri, geniş oyun alternatifleri ve kullanıcı dostu mobil uygulaması ile Casibom, oyun sevenler için ideal bir platform sağlar.
주식신용
로드스탁과의 레버리지 스탁: 투자법의 참신한 분야
로드스탁을 통해 제공하는 레버리지 방식의 스탁은 주식 시장의 투자의 한 방식으로, 큰 수익률을 목표로 하는 투자자들에게 매혹적인 선택입니다. 레버리지를 사용하는 이 방법은 투자자들이 자신의 자금을 초과하는 투자금을 투자할 수 있도록 함으로써, 주식 시장에서 더 큰 작용을 행사할 수 있는 기회를 줍니다.
레버리지 방식의 스탁의 기본 원칙
레버리지 스탁은 기본적으로 자본을 대여하여 투자하는 방법입니다. 예를 들어, 100만 원의 자본으로 1,000만 원 상당의 주식을 취득할 수 있는데, 이는 투자하는 사람이 기본적인 자본보다 훨씬 훨씬 더 많은 증권을 구매하여, 주식 가격이 상승할 경우 관련된 훨씬 더 큰 수익을 얻을 수 있게 됩니다. 하지만, 증권 값이 떨어질 경우에는 그 손실 또한 커질 수 있으므로, 레버리지 사용을 사용할 때는 신중해야 합니다.
투자 계획과 레버리지
레버리지는 특히 성장 가능성이 큰 사업체에 투자할 때 유용합니다. 이러한 회사에 큰 비중으로 투입하면, 잘 될 경우 상당한 이익을 획득할 수 있지만, 반대의 경우 상당한 위험성도 짊어져야 합니다. 그렇기 때문에, 투자하는 사람은 자신의 위험 관리 능력을 가진 장터 분석을 통해, 일정한 기업에 얼마만큼의 자본을 적용할지 결정해야 합니다.
레버리지 사용의 장점과 위험 요소
레버리지 스탁은 큰 이익을 약속하지만, 그만큼 큰 위험성 따릅니다. 증권 장의 변동은 추정이 곤란하기 때문에, 레버리지를 이용할 때는 늘 상장 동향을 정밀하게 주시하고, 손해를 최소로 줄일 수 있는 전략을 마련해야 합니다.
결론: 신중한 선택이 요구됩니다
로드스탁에서 제공하는 레버리지 스탁은 효과적인 투자 수단이며, 잘 사용하면 상당한 수입을 제공할 수 있습니다. 그러나 높은 위험도 신경 써야 하며, 투자 결정이 충분히 많은 사실과 세심한 생각 후에 이루어져야 합니다. 투자자 본인의 금융 상황, 리스크 감수 능력, 그리고 시장 상황을 반영한 균형 잡힌 투자 전략이 중요하며.
Thanks , I have recently been searching for info approximately this subject for a while and yours is the greatest I’ve discovered till now. But, what concerning the conclusion? Are you positive in regards to the supply?
Hey there would you mind letting me know which web host you’re using? I’ve loaded your blog in 3 completely different web browsers and I must say this blog loads a lot faster then most. Can you recommend a good web hosting provider at a honest price? Thank you, I appreciate it!
order microzide generic – order felodipine 5mg pills bisoprolol 5mg price
buy metoprolol 100mg without prescription – lopressor 50mg drug buy generic adalat 10mg
проверить свои usdt на чистоту
Анализ кошелька на присутствие неправомерных средств: Обеспечение безопасности своего криптовалютного портфельчика
В мире криптовалют становится все важнее обеспечивать защиту своих финансов. Каждый день кибермошенники и хакеры создают свежие способы мошенничества и угонов цифровых денег. Один из основных способов обеспечения является проверка кошельков для хранения криптовалюты на выявление наличия неправомерных финансовых средств.
По какой причине поэтому важно, чтобы проверять собственные электронные кошельки для хранения криптовалюты?
Прежде всего, вот этот момент необходимо для того, чтобы обеспечения безопасности личных денег. Многие участники рынка рискуют потерять потери средств их финансов в результате несправедливых планов или угонов. Анализ кошельков способствует предотвращению обнаружить на своем пути непонятные операции и предотвратить возможные убытки.
Что предлагает вашему вниманию фирма?
Мы предлагаем услугу проверки кошельков криптовалютных кошельков и транзакций средств с задачей идентификации начала средств передвижения и выдачи подробного отчета. Фирма предоставляет технология осматривает данные для идентификации незаконных манипуляций и оценить риск для того, чтобы своего портфеля активов. Благодаря нашей службе проверки, вы будете в состоянии избежать с регуляторами и защитить от непреднамеренного вовлечения в нелегальных операций.
Как происходит процесс проверки?
Организация наша компания сотрудничает с ведущими аудиторскими фирмами, как например Halborn, чтобы гарантировать и адекватность наших анализов. Мы применяем новейшие и методы проверки данных для обнаружения подозрительных манипуляций. Персональные данные наших заказчиков обрабатываются и хранятся в специальной базе данных в соответствии высокими стандартами безопасности и конфиденциальности.
Основной запрос: “проверить свои USDT на чистоту”
Если вам нужно убедиться в надежности своих кошельков USDT, наши эксперты предлагает возможность бесплатной проверки первых 5 кошельков. Просто введите свой кошелек в указанное место на нашем сайте проверки, и мы предоставим вам детальный отчет о состоянии вашего кошелька.
Обезопасьте свои финансовые средства прямо сейчас!
Избегайте риска попасть пострадать мошенников или попасть неприятном положении неправомерных действий с вашими личными средствами. Обратитесь к профессионалам, которые помогут, вам защититься финансовые активы и предотвратить. Сделайте первый шаг защите личного цифрового портфеля активов прямо сейчас!
чистый ли usdt
Анализ Tether в нетронутость: Каким образом защитить собственные криптовалютные финансы
Все более людей придают важность на безопасность своих цифровых финансов. День ото дня мошенники изобретают новые подходы разграбления цифровых средств, и владельцы криптовалюты являются жертвами их обманов. Один способов сбережения становится проверка кошельков в присутствие противозаконных средств.
С каким намерением это важно?
В первую очередь, для того чтобы защитить собственные активы от шарлатанов и похищенных монет. Многие инвесторы сталкиваются с потенциальной угрозой потери их финансов по причине хищных сценариев либо хищений. Проверка бумажников помогает выявить непрозрачные операции а также предотвратить возможные убытки.
Что наша группа предлагаем?
Наша компания предлагаем услугу проверки криптовалютных бумажников а также операций для выявления источника средств. Наша платформа проверяет данные для выявления нелегальных операций и проценки угрозы для вашего портфеля. Вследствие этой проверке, вы сможете избегнуть проблем с регуляторами и также предохранить себя от участия в противозаконных переводах.
Как это действует?
Наша команда сотрудничаем с лучшими аудиторскими фирмами, наподобие Halborn, для того чтобы гарантировать аккуратность наших проверок. Мы внедряем передовые технологии для выявления опасных транзакций. Ваши информация проходят обработку и сохраняются согласно с высокими стандартами безопасности и конфиденциальности.
Как проверить личные Tether в нетронутость?
Если хотите проверить, что ваша Tether-кошельки чисты, наш сервис предлагает бесплатное тестирование первых пяти кошельков. Просто введите место собственного кошелька на на нашем веб-сайте, и также мы предоставим вам детальный отчет о его статусе.
Гарантируйте безопасность для свои фонды сегодня же!
Не рискуйте стать жертвой мошенников или попасть в неприятную обстановку вследствие противозаконных сделок. Посетите нашему сервису, для того чтобы сохранить ваши криптовалютные активы и предотвратить неприятностей. Примите первый шаг к безопасности вашего криптовалютного портфеля прямо сейчас!
Осмотр USDT на чистоту: Как обезопасить свои криптовалютные активы
Все больше граждан заботятся на секурити собственных криптовалютных активов. Ежедневно шарлатаны разрабатывают новые подходы кражи цифровых активов, а также собственники криптовалюты являются жертвами своих обманов. Один подходов обеспечения безопасности становится проверка кошельков для наличие противозаконных средств.
Для чего это потребуется?
Преимущественно, чтобы сохранить свои средства от обманщиков и также украденных монет. Многие инвесторы встречаются с вероятностью потери своих фондов вследствие хищных планов или хищений. Проверка кошельков помогает определить подозрительные транзакции и предотвратить потенциальные потери.
Что наша команда предлагаем?
Наша компания предлагаем услугу тестирования криптовалютных кошельков и также транзакций для обнаружения начала денег. Наша платформа анализирует информацию для выявления нелегальных операций и также оценки риска для вашего счета. За счет данной проверке, вы сможете избегать проблем с регуляторами или обезопасить себя от участия в нелегальных операциях.
Как это действует?
Мы работаем с лучшими аудиторскими организациями, наподобие Cure53, чтобы обеспечить точность наших проверок. Мы применяем новейшие технологии для выявления опасных операций. Ваши данные обрабатываются и сохраняются согласно с высокими нормами безопасности и конфиденциальности.
Как выявить свои Tether на прозрачность?
Если вам нужно проверить, что ваши Tether-кошельки прозрачны, наш сервис предоставляет бесплатную проверку первых пяти кошельков. Легко введите адрес вашего кошелька на нашем сайте, или мы предложим вам подробный отчет о его статусе.
Гарантируйте безопасность для вашими фонды прямо сейчас!
Избегайте риска попасть в жертву дельцов или оказаться в неприятную обстановку вследствие незаконных операций. Обратитесь за помощью к нам, чтобы предохранить ваши цифровые средства и предотвратить затруднений. Предпримите первый шаг к сохранности вашего криптовалютного портфеля сегодня!
Осмотр USDT в чистоту: Каким образом обезопасить собственные электронные активы
Каждый день все больше граждан обращают внимание к безопасность собственных криптовалютных средств. День ото дня дельцы изобретают новые схемы разграбления цифровых активов, или собственники криптовалюты становятся пострадавшими их афер. Один из подходов охраны становится проверка кошельков в наличие противозаконных средств.
Зачем это потребуется?
Прежде всего, с тем чтобы защитить свои финансы от обманщиков или украденных монет. Многие специалисты сталкиваются с вероятностью утраты их активов в результате мошеннических сценариев либо грабежей. Проверка кошельков способствует выявить подозрительные действия а также предотвратить возможные убытки.
Что наша группа предоставляем?
Мы предлагаем подход проверки криптовалютных бумажников и операций для определения начала фондов. Наша система анализирует информацию для обнаружения нелегальных транзакций и оценки риска для вашего портфеля. За счет этой проверке, вы сможете избегнуть проблем с регуляторами и также защитить себя от участия в нелегальных переводах.
Как происходит процесс?
Наша фирма работаем с ведущими проверочными фирмами, наподобие Certik, чтобы гарантировать аккуратность наших проверок. Мы внедряем современные технологии для выявления опасных транзакций. Ваши данные проходят обработку и сохраняются согласно с высокими стандартами безопасности и конфиденциальности.
Как выявить свои Tether для нетронутость?
Если вам нужно проверить, что ваши Tether-кошельки чисты, наш сервис обеспечивает бесплатную проверку первых пяти кошельков. Просто введите положение своего бумажника в на нашем веб-сайте, а также наша команда предоставим вам детальный отчет о его статусе.
Гарантируйте безопасность для вашими активы уже сегодня!
Не подвергайте риску попасть в жертву обманщиков или попадать в неприятную обстановку из-за незаконных операций. Свяжитесь с нам, с тем чтобы обезопасить ваши электронные активы и избежать затруднений. Совершите первый шаг к безопасности вашего криптовалютного портфеля прямо сейчас!
order generic nitroglycerin – purchase clonidine pills valsartan online order
I think this is among the most vital info for me. And i am glad reading your article. But should remark on some general things, The site style is wonderful, the articles is really excellent : D. Good job, cheers
Осмотр Тетер в прозрачность: Как защитить собственные криптовалютные активы
Все больше людей обращают внимание к безопасность личных криптовалютных средств. День ото дня мошенники изобретают новые способы разграбления криптовалютных средств, и владельцы криптовалюты становятся жертвами их интриг. Один из способов обеспечения безопасности становится проверка бумажников в наличие незаконных средств.
Для чего это полезно?
Преимущественно, для того чтобы защитить личные активы от шарлатанов и также похищенных денег. Многие участники сталкиваются с риском потери личных фондов из-за мошеннических сценариев либо хищений. Тестирование бумажников способствует обнаружить сомнительные операции и также предотвратить возможные потери.
Что мы предлагаем?
Мы предлагаем услугу проверки электронных кошельков а также транзакций для выявления источника денег. Наша платформа исследует информацию для выявления противозаконных операций и также оценки риска вашего счета. Из-за такой проверке, вы сможете избегать проблем с регуляторами или защитить себя от участия в незаконных операциях.
Как это действует?
Наша фирма работаем с лучшими проверочными компаниями, например Halborn, для того чтобы гарантировать аккуратность наших тестирований. Наша команда внедряем современные технологии для выявления опасных операций. Ваши данные проходят обработку и хранятся в соответствии с высокими стандартами безопасности и конфиденциальности.
Каким образом проверить собственные USDT на чистоту?
В случае если вы желаете убедиться, что ваша Tether-кошельки прозрачны, наш сервис предоставляет бесплатное тестирование первых пяти кошельков. Просто введите положение личного кошелька на на нашем веб-сайте, и наша команда предложим вам детальный доклад о его статусе.
Защитите вашими активы прямо сейчас!
Не подвергайте риску подвергнуться дельцов или попадать в неприятную ситуацию по причине нелегальных операций. Обратитесь к нашему агентству, для того чтобы предохранить ваши цифровые финансовые ресурсы и избежать сложностей. Примите первый шаг к безопасности вашего криптовалютного портфеля уже сегодня!
Тетер – это надежная криптовалюта, привязанная к национальной валюте, подобно американский доллар. Данное обстоятельство делает данную криптовалюту особенно популярной у трейдеров, так как данная криптовалюта обеспечивает стабильность курса в в условиях волатильности рынка криптовалют. Все же, как и другая разновидность цифровых активов, USDT подвергается вероятности использования в целях отмывания денег и субсидирования противоправных сделок.
Легализация доходов путем криптовалюты становится все более и более обычным способом для сокрытия происхождения капитала. Воспользовавшись разнообразные техники, мошенники могут стараться промывать нелегально приобретенные деньги посредством сервисы обмена криптовалют или миксеры средств, с тем чтобы осуществить происхождение менее очевидным.
Именно для этой цели, экспертиза USDT на чистоту оказывается весьма существенной инструментом защиты для того чтобы владельцев криптовалют. Доступны для использования специализированные услуги, которые проводят проверку операций и счетов, чтобы обнаружить подозрительные сделки и незаконные источники капитала. Такие сервисы содействуют участникам устранить непреднамеренного участия в финансирование преступных акций и предотвратить блокировку счетов со стороны надзорных органов.
Анализ USDT на чистоту также как способствует предохранить себя от потенциальных финансовых потерь. Владельцы могут быть уверенны в том их капитал не связаны с противоправными транзакциями, что в свою очередь снижает риск блокировки аккаунта или перечисления денег.
Таким образом, в текущей ситуации возрастающей сложности среды криптовалют важно принимать меры для обеспечения безопасности и надежности своего капитала. Экспертиза USDT на чистоту с использованием специальных платформ является одним из вариантов предотвращения незаконной деятельности, предоставляя владельцам криптовалют дополнительную защиту и надежности.
cá cược thể thao
https://rgames.org/cup-c1/
Backlinks seo
Efficient Backlinks in Blogs and Remarks: Enhance Your SEO
Links are crucial for improving search engine rankings and raising website visibility. By integrating hyperlinks into blogs and comments prudently, they can significantly increase traffic and SEO overall performance.
Adhering to Search Engine Algorithms
The current day’s backlink positioning tactics are meticulously tuned to align with search engine algorithms, which now prioritize link high quality and relevance. This ensures that hyperlinks are not just abundant but significant, directing consumers to beneficial and relevant content. Site owners should concentrate on integrating backlinks that are contextually suitable and boost the overall content material good quality.
Advantages of Making use of Refreshing Contributor Bases
Utilizing up-to-date contributor bases for hyperlinks, like those maintained by Alex, delivers significant rewards. These bases are often refreshed and comprise of unmoderated sites that don’t pull in complaints, ensuring the links placed are both powerful and compliant. This method helps in sustaining the efficacy of links without the dangers associated with moderated or problematic sources.
Only Authorized Resources
All donor sites used are authorized, steering clear of legal pitfalls and conforming to digital marketing requirements. This dedication to making use of only approved resources ensures that each backlink is genuine and reliable, thereby developing reliability and reliability in your digital presence.
SEO Impact
Skillfully put backlinks in blogs and remarks provide greater than just SEO advantages—they enhance user experience by linking to relevant and high-quality articles. This technique not only satisfies search engine requirements but also engages users, leading to much better visitors and improved online proposal.
In essence, the right backlink technique, particularly one that employs fresh and dependable donor bases like Alex’s, can alter your SEO efforts. By concentrating on good quality over volume and adhering to the most recent criteria, you can ensure your backlinks are both effective and efficient.
九州娛樂城登入
usdt не чистое
Проверка Tether в чистоту: Каким образом сохранить свои криптовалютные активы
Постоянно все больше индивидуумов заботятся в безопасность личных криптовалютных активов. День ото дня обманщики предлагают новые подходы разграбления криптовалютных активов, и владельцы электронной валюты являются пострадавшими их афер. Один из подходов сбережения становится проверка бумажников для присутствие незаконных средств.
Зачем это необходимо?
Преимущественно, чтобы защитить собственные активы против шарлатанов и также похищенных монет. Многие вкладчики сталкиваются с вероятностью убытков своих финансов в результате мошеннических механизмов или кражей. Анализ бумажников способствует обнаружить сомнительные действия и предотвратить возможные потери.
Что мы предлагаем?
Наша компания предлагаем подход тестирования криптовалютных кошельков или операций для определения источника фондов. Наша технология исследует информацию для определения нелегальных транзакций а также проценки угрозы для вашего портфеля. Вследствие этой проверке, вы сможете избежать проблем с регуляторами и обезопасить себя от участия в противозаконных операциях.
Как это работает?
Мы сотрудничаем с первоклассными аудиторскими фирмами, например Certik, для того чтобы обеспечить прецизионность наших тестирований. Мы используем передовые технологии для обнаружения рискованных сделок. Ваши данные проходят обработку и сохраняются в соответствии с высокими нормами безопасности и конфиденциальности.
Как проверить личные Tether для чистоту?
Если вам нужно подтвердить, что ваши Tether-кошельки чисты, наш сервис предоставляет бесплатное тестирование первых пяти бумажников. Легко введите положение собственного кошелька на на нашем веб-сайте, и наш сервис предоставим вам детальный доклад о его статусе.
Гарантируйте безопасность для вашими активы уже сейчас!
Не подвергайте риску подвергнуться дельцов или попадать в неприятную ситуацию по причине незаконных транзакций. Обратитесь к нам, для того чтобы защитить ваши криптовалютные активы и избежать проблем. Сделайте первый шаг к сохранности криптовалютного портфеля уже сегодня!
Productive Backlinks in Blogs and Comments: Increase Your SEO
Backlinks are vital for increasing search engine rankings and increasing web site presence. By including links into blogs and comments prudently, they can significantly enhance visitors and SEO efficiency.
Adhering to Search Engine Algorithms
Today’s backlink positioning strategies are finely adjusted to align with search engine algorithms, which now prioritize link quality and relevance. This guarantees that backlinks are not just abundant but significant, directing consumers to helpful and pertinent content. Site owners should concentrate on integrating links that are contextually proper and improve the general content material good quality.
Benefits of Making use of Fresh Donor Bases
Making use of up-to-date contributor bases for hyperlinks, like those maintained by Alex, offers considerable rewards. These bases are often refreshed and consist of unmoderated sites that don’t attract complaints, ensuring the hyperlinks placed are both influential and certified. This strategy assists in sustaining the effectiveness of backlinks without the dangers connected with moderated or troublesome resources.
Only Authorized Sources
All donor sites used are approved, steering clear of legal pitfalls and conforming to digital marketing standards. This commitment to using only sanctioned resources assures that each backlink is genuine and reliable, thereby building trustworthiness and trustworthiness in your digital presence.
SEO Impact
Skillfully positioned backlinks in weblogs and remarks provide more than just SEO rewards—they boost user encounter by linking to pertinent and top quality articles. This strategy not only meets search engine criteria but also engages end users, leading to far better traffic and improved online involvement.
In essence, the right backlink strategy, specifically one that utilizes fresh and reliable donor bases like Alex’s, can transform your SEO efforts. By focusing on quality over amount and adhering to the newest standards, you can make sure your backlinks are both effective and efficient.
Telegrass
שרף מדריך: המכון השלם לרכישת פרחי קנאביס דרך המסר
פרח הנחיות היא אתר רשמי מידעים ומדריכי לסחר ב קנאביס באמצעות התוכנה הפופולארית מסר.
האתר הוצע את כל הקישורים לאתרים והמידעים המתעדף לקבוצות המשתמשים וערוצים באתר מומלצות לסחר ב קנאביס בטלגרם במדינה.
כמו לצד זאת, פורטל מספק מדריכים מפורטת לאיך כדאי להתארגן בהקנאביס ולקנה פרחי קנאביס בקלות הזמנה ובמהירות מירבית.
בעזרת המדריכים, גם כן משתמשים משתמשים חדשים יוכלו להתחיל להחיים הקנאביס בהמשלוח בפניות מוגנת ומאובטחת לשימוש.
ההאוטומטיזציה של טלגראס מאפשר למשתמשי ללבצע פעולות המבוצעות שונות וצבעוניות כמו כן הפעלת פרחי קנאביס, קבלה תמיכה, בדיקת והוספת ביקורות על מוצרים. כל זאת בפני פשוטה ופשוטה דרך התוכנה.
כאשר כאשר מדובר בשיטות התשלומים, הקנאביס משתמשת בדרכי מוכרות כמו מזומנים, כרטיסים של אשראי וקריפטומונדה. חשוב לציין כי קיים לבדוק ולוודא את ההנחיות והחוקים המקומיים במדינה שלך ללפני ביצוע רכישה.
טלגרם מציע יתרונות ראשיים כגון פרטיות וביטחון אישי מוגברים מאוד, התקשורת מהירה וגמישות גבוהה. בנוסף, הוא מאפשר כניסה להקהל עולמית רחבה ומציע מגוון של תכונות ויכולות.
בסיכום, הטלגרמה מדריכים הם המקום האידיאלי למצוא את כל המידע והקישורים לסחר ב שרף בפניות מהירה מאוד, בבטוחה ונוחה דרך הטלגרם.
הימורי ספורט
הימורים מקוונים הם חווייה מרגשת ופופולרית ביותר בעידן הדיגיטלי, שמגירה מיליונים אנשים מכל
רחבי העולם. ההימורים המקוונים מתנהלים על פי אירועים ספורטיביים, תוצאות פוליטיות ואפילו תוצאות מזג האוויר ונושאים נוספים. אתרים ל הימורים הווירטואליים מקריאים את כל מי שרוצה להמר על תוצאות אפשרות וליהנות חוויות ייחודיות ומרתקות.
ההימורים המקוונים הם כבר חלק מהותי מהתרבות האנושית מזמן רב והיום הם לא רק חלק מרכזי מהפעילות הכלכלית והתרבותית, אלא אף מספקים רווחים וחוויות מרתקות. משום שהם נגישים מאוד וקלים לשימוש, הם מובילים את כולם ליהנות מהניסיון ולהנות מכל הניצחונות והפרסים בכל זמן ובכל מקום.
טכנולוגיות דיגיטליות והימורים הפכו להיות הפופולריים ביותר מעניינת ונפוצה. מיליונים אנשים מכל כל רחבי העולם משתתפים בהימורים, כוללים סוגים שונים של הימורים. הימורים מקוונים מציעים למשתתפים חוויה רגשית ומרתקת, המאפשרת להם ליהנות מפעילות פופולרית זו בכל זמן ובכל מקום.
אז מה נותר אתה מחכה לו? הצטרף עכשיו והתחיל ליהנות מהתרגשות וההנאה שהימורים מקוונים מציעים.
Sure, here’s the text with spin syntax applied:
Hyperlink Hierarchy
After numerous updates to the G search algorithm, it is essential to use different strategies for ranking.
Today there is a means to attract the interest of search engines to your site with the aid of inbound links.
Links are not only an successful marketing instrument but they also have authentic visitors, direct sales from these sources perhaps will not be, but visits will be, and it is advantageous traffic that we also receive.
What in the end we get at the output:
We show search engines site through links.
Prluuchayut natural transitions to the site and it is also a signal to search engines that the resource is used by users.
How we show search engines that the site is valuable:
Links do to the main page where the main information.
We make links through redirections credible sites.
The most CRUCIAL we place the site on sites analyzers individual tool, the site goes into the cache of these analysis tools, then the obtained links we place as redirections on weblogs, discussion boards, comment sections. This crucial action shows search engines the site map as analyzer sites present all information about sites with all keywords and headlines and it is very BENEFICIAL.
All details about our services is on the website!
crestor pills solid – caduet buy better caduet buy atmosphere
link building
Link building is just just as effective now, only the tools to operate within this domain have altered.
There are numerous possibilities regarding backlinks, our team utilize some of them, and these methods operate and are actually examined by us and our clients.
Recently we conducted an experiment and we found that low-volume search queries from a single domain name ranking well in online searches, and it doesnt have being your domain, it is possible to use social networks from the web 2.0 collection for this.
It is also possible to partly transfer mass through site redirects, providing a diverse hyperlink profile.
Head over to our own web page where our company’s services are typically provided with detailed explanations.
Creating unique articles on Platform and Platform, why it is essential:
Created article on these resources is improved ranked on less frequent queries, which is very significant to get organic traffic.
We get:
organic traffic from search engines.
natural traffic from the inner rendition of the medium.
The platform to which the article refers gets a link that is liquid and increases the ranking of the platform to which the article refers.
Articles can be made in any number and choose all less common queries on your topic.
Medium pages are indexed by search algorithms very well.
Telegraph pages need to be indexed distinctly indexer and at the same time after indexing they sometimes occupy spots higher in the search algorithms than the medium, these two platforms are very helpful for getting visitors.
Here is a link to our services where we offer creation, indexing of sites, articles, pages and more.
zocor electric – lopid mankind lipitor smooth
можно ли разорвать контракт сво
С началом СВО уже спустя полгода была объявлена первая волна мобилизации. При этом прошлая, в последний раз в России была аж в 1941 году, с началом Великой Отечественной Войны. Конечно же, желающих отправиться на фронт было не много, а потому люди стали искать способы не попасть на СВО, для чего стали покупать справки о болезнях, с которыми можно получить категорию Д. И все это стало возможным с даркнет сайтами, где можно найти практически все что угодно. Именно об этой отрасли темного интернета подробней и поговорим в этой статье.
viagra professional online important – viagra gold online england levitra oral jelly object
Ikaria Lean Belly Juice is a fast-acting weight loss supplement that is developed to incinerate stubborn fat.
Pirámide de backlinks
Aquí está el texto con la estructura de spintax que propone diferentes sinónimos para cada palabra:
“Pirámide de enlaces de retroceso
Después de numerosas actualizaciones del motor de búsqueda G, necesita aplicar diferentes opciones de clasificación.
Hay una forma de llamar la atención de los motores de búsqueda a su sitio web con backlinks.
Los vínculos de retroceso no sólo son una estrategia eficaz para la promoción, sino que también tienen tráfico orgánico, las ventas directas de estos recursos más probable es que no será, pero las transiciones será, y es poedenicheskogo tráfico que también obtenemos.
Lo que vamos a obtener al final en la salida:
Mostramos el sitio a los motores de búsqueda a través de backlinks.
Conseguimos conversiones orgánicas hacia el sitio, lo que también es una señal para los buscadores de que el recurso está siendo utilizado por la gente.
Cómo mostramos los motores de búsqueda que el sitio es líquido:
1 enlace se hace a la página principal donde está la información principal
Hacemos backlinks a través de redirecciones de sitios de confianza
Lo más vital colocamos el sitio en una herramienta independiente de analizadores de sitios, el sitio entra en la caché de estos analizadores, luego los enlaces recibidos los colocamos como redirecciones en blogs, foros, comentarios.
Esta importante acción muestra a los buscadores el MAPA DEL SITIO, ya que los analizadores de sitios muestran toda la información de los sitios con todas las palabras clave y títulos y es muy efectivo.
¡Toda la información sobre nuestros servicios en el sitio web!
反向連結金字塔
反向链接金字塔
G搜尋引擎在多番更新之后需要应用不同的排名參數。
今天有一種方法可以使用反向連結吸引G搜尋引擎對您的網站的注意。
反向連結不僅是有效的推廣工具,也是有機流量。
我們會得到什麼結果:
我們透過反向連結向G搜尋引擎展示我們的網站。
他們收到了到該網站的自然過渡,這也是向G搜尋引擎發出的信號,表明該資源正在被人們使用。
我們如何向G搜尋引擎表明該網站具有流動性:
個帶有主要訊息的主頁反向链接
我們透過來自受信任網站的重定向來建立反向連接。
此外,我們將網站放置在單獨的網路分析器上,網站最終會進入這些分析器的缓存中,然後我們使用產生的連結作為部落格、論壇和評論的重新导向。 這個重要的操作向G搜尋引擎顯示了網站地圖,因為網站分析器顯示了有關網站的所有資訊以及所有關鍵字和標題,這很棒
有關我們服務的所有資訊都在網站上!
priligy shrug – cialis with dapoxetine joy cialis with dapoxetine river
cenforce hail – zenegra edge brand viagra pills england
взлом кошелька
Как охранять свои личные данные: страхуйтесь от утечек информации в интернете. Сегодня защита информации становится всё больше важной задачей. Одним из наиболее часто встречающихся способов утечки личной информации является слив «сит фраз» в интернете. Что такое сит фразы и в какой мере защититься от их утечки? Что такое «сит фразы»? «Сит фразы» — это сочетания слов или фраз, которые постоянно используются для доступа к различным онлайн-аккаунтам. Эти фразы могут включать в себя имя пользователя, пароль или другие конфиденциальные данные. Киберпреступники могут пытаться получить доступ к вашим аккаунтам, при помощи этих сит фраз. Как сохранить свои личные данные? Используйте комплексные пароли. Избегайте использования простых паролей, которые просто угадать. Лучше всего использовать комбинацию букв, цифр и символов. Используйте уникальные пароли для каждого аккаунта. Не воспользуйтесь один и тот же пароль для разных сервисов. Используйте двухступенчатую аутентификацию (2FA). Это вводит дополнительный уровень безопасности, требуя подтверждение входа на ваш аккаунт по другое устройство или метод. Будьте осторожны с онлайн-сервисами. Не доверяйте свою информацию ненадежным сайтам и сервисам. Обновляйте программное обеспечение. Установите обновления для вашего операционной системы и программ, чтобы защитить свои данные от вредоносного ПО. Вывод Слив сит фраз в интернете может привести к серьезным последствиям, таким подобно кража личной информации и финансовых потерь. Чтобы защитить себя, следует принимать меры предосторожности и использовать надежные методы для хранения и управления своими личными данными в сети
даркнет сливы тг
Даркнет и сливы в Телеграме
Даркнет – это часть интернета, которая не индексируется обыденными поисковыми системами и требует индивидуальных программных средств для доступа. В даркнете существует масса скрытых сайтов, где можно найти различные товары и услуги, в том числе и нелегальные.
Одним из трендовых способов распространения информации в даркнете является использование мессенджера Телеграм. Телеграм предоставляет возможность создания закрытых каналов и чатов, где пользователи могут обмениваться информацией, в том числе и нелегальной.
Сливы информации в Телеграме – это процедура распространения конфиденциальной информации, такой как украденные данные, базы данных, персональные сведения и другие материалы. Эти сливы могут включать в себя информацию о кредитных картах, паролях, персональных сообщениях и даже фотографиях.
Сливы в Телеграме могут быть рискованными, так как они могут привести к утечке конфиденциальной информации и нанести ущерб репутации и финансовым интересам людей. Поэтому важно быть внимательным при обмене информацией в интернете и не доверять сомнительным источникам.
Вот кошельки с балансом у бота
сид фразы кошельков
Сид-фразы, или напоминающие фразы, представляют собой сумму слов, которая используется для генерации или восстановления кошелька криптовалюты. Эти фразы обеспечивают доступ к вашим криптовалютным средствам, поэтому их надежное хранение и использование весьма важны для защиты вашего криптоимущества от утери и кражи.
Что такое сид-фразы кошельков криптовалют?
Сид-фразы представляют собой набор произвольно сгенерированных слов, часто от 12 до 24, которые представляют собой для создания уникального ключа шифрования кошелька. Этот ключ используется для восстановления возможности доступа к вашему кошельку в случае его повреждения или утери. Сид-фразы обладают значительной защиты и шифруются, что делает их безопасными для хранения и передачи.
Зачем нужны сид-фразы?
Сид-фразы неотъемлемы для обеспечения безопасности и доступности вашего криптоимущества. Они позволяют восстановить вход к кошельку в случае утери или повреждения физического устройства, на котором он хранится. Благодаря сид-фразам вы можете без труда создавать резервные копии своего кошелька и хранить их в безопасном месте.
Как обеспечить безопасность сид-фраз кошельков?
Никогда не раскрывайте сид-фразой ни с кем. Сид-фраза является вашим ключом к кошельку, и ее раскрытие может вести к утере вашего криптоимущества.
Храните сид-фразу в секурном месте. Используйте физически безопасные места, такие как банковские ячейки или специализированные аппаратные кошельки, для хранения вашей сид-фразы.
Создавайте резервные копии сид-фразы. Регулярно создавайте резервные копии вашей сид-фразы и храните их в разных безопасных местах, чтобы обеспечить возможность доступа к вашему кошельку в случае утери или повреждения.
Используйте дополнительные меры безопасности. Включите двухфакторную аутентификацию и другие методы защиты для своего кошелька криптовалюты, чтобы обеспечить дополнительный уровень безопасности.
Заключение
Сид-фразы кошельков криптовалют являются ключевым элементом надежного хранения криптоимущества. Следуйте рекомендациям по безопасности, чтобы защитить свою сид-фразу и обеспечить безопасность своих криптовалютных средств.
Слив посеянных фраз (seed phrases) является одним из наиболее распространенных способов утечки личной информации в мире криптовалют. В этой статье мы разберем, что такое сид фразы, отчего они важны и как можно защититься от их утечки.
Что такое сид фразы?
Сид фразы, или мнемонические фразы, представляют собой комбинацию слов, которая используется для создания или восстановления кошелька криптовалюты. Обычно сид фраза состоит из 12 или 24 слов, которые символизируют собой ключ к вашему кошельку. Потеря или утечка сид фразы может влечь за собой потере доступа к вашим криптовалютным средствам.
Почему важно защищать сид фразы?
Сид фразы служат ключевым элементом для безопасного хранения криптовалюты. Если злоумышленники получат доступ к вашей сид фразе, они могут получить доступ к вашему кошельку и украсть все средства.
Как защититься от утечки сид фраз?
Никогда не передавайте свою сид фразу никому, даже если вам происходит, что это привилегированное лицо или сервис.
Храните свою сид фразу в защищенном и безопасном месте. Рекомендуется использовать аппаратные кошельки или специальные программы для хранения сид фразы.
Используйте дополнительные методы защиты, такие как двухфакторная верификация, для усиления безопасности вашего кошелька.
Регулярно делайте резервные копии своей сид фразы и храните их в различных безопасных местах.
Заключение
Слив сид фраз является серьезной угрозой для безопасности владельцев криптовалют. Понимание важности защиты сид фразы и принятие соответствующих мер безопасности помогут вам избежать потери ваших криптовалютных средств. Будьте бдительны и обеспечивайте надежную защиту своей сид фразы
Столбец Backlinks
После многочисленных обновлений поисковой системы G необходимо применять разнообразные варианты ранжирования.
Сегодня есть способ привлечь внимание поисковых систем к вашему сайту с помощью обратных ссылок.
Обратные линки не только эффективный инструмент продвижения, но и обладают органическим трафиком, хотя прямых продаж с этих ресурсов скорее всего не будет, но переходы будут, и именно органического трафика мы также достигаем.
Что в итоге получим на выходе:
Мы отображаем сайт поисковым системам с помощью обратных ссылок.
Получают естественные переходы на сайт, а это также информация для поисковых систем, что ресурс пользуется спросом у пользователей.
Как мы указываем поисковым системам, что сайт ликвиден:
1 обратная ссылка делается на главную страницу, где основная информация.
Создаем обратные ссылки с использованием редиректов с доверенных сайтов.
Самое ВАЖНОЕ мы размещаем сайт на отдельном инструменте анализаторов сайтов, сайт попадает в кеш этих анализаторов, затем полученные ссылки мы размещаем в качестве редиректов на блогах, форумах, комментариях.
Это нужное действие показывает потсковикамКАРТУ САЙТА, так как анализаторы сайтов показывают всю информацию о сайтах со всеми ключевыми словами и заголовками и это очень ВАЖНО
Hello there! Quick question that’s entirely off topic. Do you know how to make your site mobile friendly? My blog looks weird when viewing from my iphone4. I’m trying to find a template or plugin that might be able to fix this problem. If you have any recommendations, please share. Thank you!
娛樂城推薦
Player線上娛樂城遊戲指南與評測
台灣最佳線上娛樂城遊戲的終極指南!我們提供專業評測,分析熱門老虎機、百家樂、棋牌及其他賭博遊戲。從遊戲規則、策略到選擇最佳娛樂城,我們全方位覆蓋,協助您更安全的遊玩。
Player如何評測:公正與專業的評分標準
在【Player娛樂城遊戲評測網】我們致力於為玩家提供最公正、最專業的娛樂城評測。我們的評測過程涵蓋多個關鍵領域,旨在確保玩家獲得可靠且全面的信息。以下是我們評測娛樂城的主要步驟:
娛樂城是什麼?
娛樂城是什麼?娛樂城是台灣對於線上賭場的特別稱呼,線上賭場分為幾種:現金版、信用版、手機娛樂城(娛樂城APP),一般來說,台灣人在稱娛樂城時,是指現金版線上賭場。
線上賭場在別的國家也有別的名稱,美國 – Casino, Gambling、中國 – 线上赌场,娱乐城、日本 – オンラインカジノ、越南 – Nhà cái。
娛樂城會被抓嗎?
在台灣,根據刑法第266條,不論是實體或線上賭博,參與賭博的行為可處最高5萬元罰金。而根據刑法第268條,為賭博提供場所並意圖營利的行為,可能面臨3年以下有期徒刑及最高9萬元罰金。一般賭客若被抓到,通常被視為輕微罪行,原則上不會被判處監禁。
信用版娛樂城是什麼?
信用版娛樂城是一種線上賭博平台,其中的賭博活動不是直接以現金進行交易,而是基於信用系統。在這種模式下,玩家在進行賭博時使用虛擬的信用點數或籌碼,這些點數或籌碼代表了一定的貨幣價值,但實際的金錢交易會在賭博活動結束後進行結算。
現金版娛樂城是什麼?
現金版娛樂城是一種線上博弈平台,其中玩家使用實際的金錢進行賭博活動。玩家需要先存入真實貨幣,這些資金轉化為平台上的遊戲籌碼或信用,用於參與各種賭場遊戲。當玩家贏得賭局時,他們可以將這些籌碼或信用兌換回現金。
娛樂城體驗金是什麼?
娛樂城體驗金是娛樂場所為新客戶提供的一種免費遊玩資金,允許玩家在不需要自己投入任何資金的情況下,可以進行各類遊戲的娛樂城試玩。這種體驗金的數額一般介於100元到1,000元之間,且對於如何使用這些體驗金以達到提款條件,各家娛樂城設有不同的規則。
娛樂城排行
Player線上娛樂城遊戲指南與評測
台灣最佳線上娛樂城遊戲的終極指南!我們提供專業評測,分析熱門老虎機、百家樂、棋牌及其他賭博遊戲。從遊戲規則、策略到選擇最佳娛樂城,我們全方位覆蓋,協助您更安全的遊玩。
Player如何評測:公正與專業的評分標準
在【Player娛樂城遊戲評測網】我們致力於為玩家提供最公正、最專業的娛樂城評測。我們的評測過程涵蓋多個關鍵領域,旨在確保玩家獲得可靠且全面的信息。以下是我們評測娛樂城的主要步驟:
娛樂城是什麼?
娛樂城是什麼?娛樂城是台灣對於線上賭場的特別稱呼,線上賭場分為幾種:現金版、信用版、手機娛樂城(娛樂城APP),一般來說,台灣人在稱娛樂城時,是指現金版線上賭場。
線上賭場在別的國家也有別的名稱,美國 – Casino, Gambling、中國 – 线上赌场,娱乐城、日本 – オンラインカジノ、越南 – Nhà cái。
娛樂城會被抓嗎?
在台灣,根據刑法第266條,不論是實體或線上賭博,參與賭博的行為可處最高5萬元罰金。而根據刑法第268條,為賭博提供場所並意圖營利的行為,可能面臨3年以下有期徒刑及最高9萬元罰金。一般賭客若被抓到,通常被視為輕微罪行,原則上不會被判處監禁。
信用版娛樂城是什麼?
信用版娛樂城是一種線上賭博平台,其中的賭博活動不是直接以現金進行交易,而是基於信用系統。在這種模式下,玩家在進行賭博時使用虛擬的信用點數或籌碼,這些點數或籌碼代表了一定的貨幣價值,但實際的金錢交易會在賭博活動結束後進行結算。
現金版娛樂城是什麼?
現金版娛樂城是一種線上博弈平台,其中玩家使用實際的金錢進行賭博活動。玩家需要先存入真實貨幣,這些資金轉化為平台上的遊戲籌碼或信用,用於參與各種賭場遊戲。當玩家贏得賭局時,他們可以將這些籌碼或信用兌換回現金。
娛樂城體驗金是什麼?
娛樂城體驗金是娛樂場所為新客戶提供的一種免費遊玩資金,允許玩家在不需要自己投入任何資金的情況下,可以進行各類遊戲的娛樂城試玩。這種體驗金的數額一般介於100元到1,000元之間,且對於如何使用這些體驗金以達到提款條件,各家娛樂城設有不同的規則。
Player線上娛樂城遊戲指南與評測
台灣最佳線上娛樂城遊戲的終極指南!我們提供專業評測,分析熱門老虎機、百家樂、棋牌及其他賭博遊戲。從遊戲規則、策略到選擇最佳娛樂城,我們全方位覆蓋,協助您更安全的遊玩。
Player如何評測:公正與專業的評分標準
在【Player娛樂城遊戲評測網】我們致力於為玩家提供最公正、最專業的娛樂城評測。我們的評測過程涵蓋多個關鍵領域,旨在確保玩家獲得可靠且全面的信息。以下是我們評測娛樂城的主要步驟:
娛樂城是什麼?
娛樂城是什麼?娛樂城是台灣對於線上賭場的特別稱呼,線上賭場分為幾種:現金版、信用版、手機娛樂城(娛樂城APP),一般來說,台灣人在稱娛樂城時,是指現金版線上賭場。
線上賭場在別的國家也有別的名稱,美國 – Casino, Gambling、中國 – 线上赌场,娱乐城、日本 – オンラインカジノ、越南 – Nhà cái。
娛樂城會被抓嗎?
在台灣,根據刑法第266條,不論是實體或線上賭博,參與賭博的行為可處最高5萬元罰金。而根據刑法第268條,為賭博提供場所並意圖營利的行為,可能面臨3年以下有期徒刑及最高9萬元罰金。一般賭客若被抓到,通常被視為輕微罪行,原則上不會被判處監禁。
信用版娛樂城是什麼?
信用版娛樂城是一種線上賭博平台,其中的賭博活動不是直接以現金進行交易,而是基於信用系統。在這種模式下,玩家在進行賭博時使用虛擬的信用點數或籌碼,這些點數或籌碼代表了一定的貨幣價值,但實際的金錢交易會在賭博活動結束後進行結算。
現金版娛樂城是什麼?
現金版娛樂城是一種線上博弈平台,其中玩家使用實際的金錢進行賭博活動。玩家需要先存入真實貨幣,這些資金轉化為平台上的遊戲籌碼或信用,用於參與各種賭場遊戲。當玩家贏得賭局時,他們可以將這些籌碼或信用兌換回現金。
娛樂城體驗金是什麼?
娛樂城體驗金是娛樂場所為新客戶提供的一種免費遊玩資金,允許玩家在不需要自己投入任何資金的情況下,可以進行各類遊戲的娛樂城試玩。這種體驗金的數額一般介於100元到1,000元之間,且對於如何使用這些體驗金以達到提款條件,各家娛樂城設有不同的規則。
взлом кошелька
Как сберечь свои данные: избегайте утечек информации в интернете. Сегодня сохранение информации становится все более важной задачей. Одним из наиболее популярных способов утечки личной информации является слив «сит фраз» в интернете. Что такое сит фразы и как предохранить себя от их утечки? Что такое «сит фразы»? «Сит фразы» — это синтезы слов или фраз, которые постоянно используются для получения доступа к различным онлайн-аккаунтам. Эти фразы могут включать в себя имя пользователя, пароль или разные конфиденциальные данные. Киберпреступники могут пытаться получить доступ к вашим аккаунтам, с помощью этих сит фраз. Как сохранить свои личные данные? Используйте сложные пароли. Избегайте использования простых паролей, которые мгновенно угадать. Лучше всего использовать комбинацию букв, цифр и символов. Используйте уникальные пароли для каждого аккаунта. Не применяйте один и тот же пароль для разных сервисов. Используйте двухфакторную аутентификацию (2FA). Это вводит дополнительный уровень безопасности, требуя подтверждение входа на ваш аккаунт путем другое устройство или метод. Будьте осторожны с онлайн-сервисами. Не доверяйте свою информацию ненадежным сайтам и сервисам. Обновляйте программное обеспечение. Установите обновления для вашего операционной системы и программ, чтобы уберечь свои данные от вредоносного ПО. Вывод Слив сит фраз в интернете может спровоцировать серьезным последствиям, таким как кража личной информации и финансовых потерь. Чтобы защитить себя, следует принимать меры предосторожности и использовать надежные методы для хранения и управления своими личными данными в сети
Даркнет и сливы в Телеграме
Даркнет – это компонент интернета, которая не индексируется регулярными поисковыми системами и требует индивидуальных программных средств для доступа. В даркнете существует изобилие скрытых сайтов, где можно найти различные товары и услуги, в том числе и нелегальные.
Одним из популярных способов распространения информации в даркнете является использование мессенджера Телеграм. Телеграм предоставляет возможность создания закрытых каналов и чатов, где пользователи могут обмениваться информацией, в том числе и нелегальной.
Сливы информации в Телеграме – это метод распространения конфиденциальной информации, такой как украденные данные, базы данных, персональные сведения и другие материалы. Эти сливы могут включать в себя информацию о кредитных картах, паролях, персональных сообщениях и даже фотографиях.
Сливы в Телеграме могут быть опасными, так как они могут привести к утечке конфиденциальной информации и нанести ущерб репутации и финансовым интересам людей. Поэтому важно быть внимательным при обмене информацией в интернете и не доверять сомнительным источникам.
Вот кошельки с балансом у бота
Сид-фразы, или напоминающие фразы, представляют собой сочетание слов, которая используется для формирования или восстановления кошелька криптовалюты. Эти фразы обеспечивают возможность доступа к вашим криптовалютным средствам, поэтому их защищенное хранение и использование весьма важны для защиты вашего криптоимущества от утери и кражи.
Что такое сид-фразы кошельков криптовалют?
Сид-фразы составляют набор произвольно сгенерированных слов, часто от 12 до 24, которые предназначены для создания уникального ключа шифрования кошелька. Этот ключ используется для восстановления входа к вашему кошельку в случае его повреждения или утери. Сид-фразы обладают большой защиты и шифруются, что делает их безопасными для хранения и передачи.
Зачем нужны сид-фразы?
Сид-фразы жизненно важны для обеспечения безопасности и доступности вашего криптоимущества. Они позволяют восстановить доступ к кошельку в случае утери или повреждения физического устройства, на котором он хранится. Благодаря сид-фразам вы можете легко создавать резервные копии своего кошелька и хранить их в безопасном месте.
Как обеспечить безопасность сид-фраз кошельков?
Никогда не раскрывайте сид-фразой ни с кем. Сид-фраза является вашим ключом к кошельку, и ее раскрытие может влечь за собой утере вашего криптоимущества.
Храните сид-фразу в безопасном месте. Используйте физически надежные места, такие как банковские ячейки или специализированные аппаратные кошельки, для хранения вашей сид-фразы.
Создавайте резервные копии сид-фразы. Регулярно создавайте резервные копии вашей сид-фразы и храните их в разных безопасных местах, чтобы обеспечить вход к вашему кошельку в случае утери или повреждения.
Используйте дополнительные меры безопасности. Включите другие методы защиты и двухфакторную верификацию для своего кошелька криптовалюты, чтобы обеспечить дополнительный уровень безопасности.
Заключение
Сид-фразы кошельков криптовалют являются ключевым элементом защищенного хранения криптоимущества. Следуйте рекомендациям по безопасности, чтобы защитить свою сид-фразу и обеспечить безопасность своих криптовалютных средств.
Криптокошельки с балансом: зачем их покупают и как использовать
В мире криптовалют все возрастающую популярность приобретают криптокошельки с предустановленным балансом. Это индивидуальные кошельки, которые уже содержат определенное количество криптовалюты на момент покупки. Но зачем люди приобретают такие кошельки, и как правильно использовать их?
Почему покупают криптокошельки с балансом?
Удобство: Криптокошельки с предустановленным балансом предлагаются как готовое к использованию решение для тех, кто хочет быстро начать пользоваться криптовалютой без необходимости покупки или обмена на бирже.
Подарок или награда: Иногда криптокошельки с балансом используются как подарок или поощрение в рамках акций или маркетинговых кампаний.
Анонимность: При покупке криптокошелька с балансом нет необходимости предоставлять личные данные, что может быть важно для тех, кто ценит анонимность.
Как использовать криптокошелек с балансом?
Проверьте безопасность: Убедитесь, что кошелек безопасен и не подвержен взлому. Проверьте репутацию продавца и источник приобретения кошелька.
Переведите средства на другой кошелек: Если вы хотите долгосрочно хранить криптовалюту, рекомендуется перевести средства на более безопасный или практичный для вас кошелек.
Не храните все средства на одном кошельке: Для обеспечения безопасности рекомендуется распределить средства между несколькими кошельками.
Будьте осторожны с фишингом и мошенничеством: Помните, что мошенники могут пытаться обмануть вас, предлагая криптокошельки с балансом с целью получения доступа к вашим средствам.
Заключение
Криптокошельки с балансом могут быть удобным и простым способом начать пользоваться криптовалютой, но необходимо помнить о безопасности и осторожности при их использовании.Выбор и приобретение криптокошелька с балансом – это важный шаг, который требует внимания к деталям и осознанного подхода.”
Слив посеянных фраз (seed phrases) является одной из наиболее распространенных способов утечки персональной информации в мире криптовалют. В этой статье мы разберем, что такое сид фразы, по какой причине они важны и как можно защититься от их утечки.
Что такое сид фразы?
Сид фразы, или мнемонические фразы, составляют комбинацию слов, которая используется для создания или восстановления кошелька криптовалюты. Обычно сид фраза состоит из 12 или 24 слов, которые символизируют собой ключ к вашему кошельку. Потеря или утечка сид фразы может приводить к потере доступа к вашим криптовалютным средствам.
Почему важно защищать сид фразы?
Сид фразы служат ключевым элементом для безопасного хранения криптовалюты. Если злоумышленники получат доступ к вашей сид фразе, они сумеют получить доступ к вашему кошельку и украсть все средства.
Как защититься от утечки сид фраз?
Никогда не передавайте свою сид фразу любому, даже если вам представляется, что это доверенное лицо или сервис.
Храните свою сид фразу в защищенном и защищенном месте. Рекомендуется использовать аппаратные кошельки или специальные программы для хранения сид фразы.
Используйте экстра методы защиты, такие как двухэтапная проверка, для усиления безопасности вашего кошелька.
Регулярно делайте резервные копии своей сид фразы и храните их в различных безопасных местах.
Заключение
Слив сид фраз является серьезной угрозой для безопасности владельцев криптовалют. Понимание важности защиты сид фразы и принятие соответствующих мер безопасности помогут вам избежать потери ваших криптовалютных средств. Будьте бдительны и обеспечивайте надежную защиту своей сид фразы
ggg
هنا النص مع استخدام السبينتاكس:
“بنية الروابط الخلفية
بعد التحديثات العديدة لمحرك البحث G، تحتاج إلى تطويق خيارات ترتيب مختلفة.
هناك طريقة لجذب انتباه محركات البحث إلى موقعك على الويب باستخدام الروابط الخلفية.
الروابط الخلفية ليست فقط أداة فعالة للترويج، ولكن تتضمن أيضًا حركة مرور عضوية، والمبيعات المباشرة من هذه الموارد على الأرجح قد لا تكون كذلك، ولكن الانتقالات ستكون، وهي حركة المرور التي نحصل عليها أيضًا.
ما سوف نحصل عليه في النهاية في النهاية في الإخراج:
نعرض الموقع لمحركات البحث من خلال الروابط الخلفية.
2- نحصل على تحويلات عضوية إلى الموقع، وهي أيضًا إشارة لمحركات البحث أن المورد يستخدمه الناس.
كيف نظهر لمحركات البحث أن الموقع سائل:
1 يتم عمل صلة خلفي للصفحة الرئيسية حيث المعلومات الرئيسية
نقوم بعمل لينكات خلفية من خلال عمليات توجيه المواقع الموثوقة
الأهم من ذلك أننا نضع الموقع على أداة منفصلة من أدوات تحليل المواقع، ويدخل الموقع في ذاكرة التخزين المؤقت لهذه المحللات، ثم الروابط المستلمة التي نضعها كإعادة توجيه على المدونات والمنتديات والتعليقات.
هذا الإجراء المهم يعرض لمحركات البحث خارطة الموقع، حيث تعرض أدوات تحليل المواقع جميع المعلومات عن المواقع مع جميع الكلمات الرئيسية والعناوين وهو أمر جيد جداً
جميع المعلومات عن خدماتنا على الموقع!
kantorbola
Kantorbola adalah situs slot gacor terbaik di indonesia , kunjungi situs RTP kantor bola untuk mendapatkan informasi akurat slot dengan rtp diatas 95% . Kunjungi juga link alternatif kami di kantorbola77 dan kantorbola99 .
brand cialis heart – tadora beg penisole cease
Nice post. I learn something more challenging on different blogs everyday. It will always be stimulating to read content from other writers and practice a little something from their store. I’d prefer to use some with the content on my blog whether you don’t mind. Natually I’ll give you a link on your web blog. Thanks for sharing.
taurus118
cialis soft tabs pills succeed – caverta captain viagra oral jelly incline
brand cialis thorough – forzest attendant penisole elsewhere
Rikvip Club: Trung Tâm Giải Trí Trực Tuyến Hàng Đầu tại Việt Nam
Rikvip Club là một trong những nền tảng giải trí trực tuyến hàng đầu tại Việt Nam, cung cấp một loạt các trò chơi hấp dẫn và dịch vụ cho người dùng. Cho dù bạn là người dùng iPhone hay Android, Rikvip Club đều có một cái gì đó dành cho mọi người. Với sứ mạng và mục tiêu rõ ràng, Rikvip Club luôn cố gắng cung cấp những sản phẩm và dịch vụ tốt nhất cho khách hàng, tạo ra một trải nghiệm tiện lợi và thú vị cho người chơi.
Sứ Mạng và Mục Tiêu của Rikvip
Từ khi bắt đầu hoạt động, Rikvip Club đã có một kế hoạch kinh doanh rõ ràng, luôn nỗ lực để cung cấp cho khách hàng những sản phẩm và dịch vụ tốt nhất và tạo điều kiện thuận lợi nhất cho người chơi truy cập. Nhóm quản lý của Rikvip Club có những mục tiêu và ước muốn quyết liệt để biến Rikvip Club thành trung tâm giải trí hàng đầu trong lĩnh vực game đổi thưởng trực tuyến tại Việt Nam và trên toàn cầu.
Trải Nghiệm Live Casino
Rikvip Club không chỉ nổi bật với sự đa dạng của các trò chơi đổi thưởng mà còn với các phòng trò chơi casino trực tuyến thu hút tất cả người chơi. Môi trường này cam kết mang lại trải nghiệm chuyên nghiệp với tính xanh chín và sự uy tín không thể nghi ngờ. Đây là một sân chơi lý tưởng cho những người yêu thích thách thức bản thân và muốn tận hưởng niềm vui của chiến thắng. Với các sảnh cược phổ biến như Roulette, Sic Bo, Dragon Tiger, người chơi sẽ trải nghiệm những cảm xúc độc đáo và đặc biệt khi tham gia vào casino trực tuyến.
Phương Thức Thanh Toán Tiện Lợi
Rikvip Club đã được trang bị những công nghệ thanh toán tiên tiến ngay từ đầu, mang lại sự thuận tiện và linh hoạt cho người chơi trong việc sử dụng hệ thống thanh toán hàng ngày. Hơn nữa, Rikvip Club còn tích hợp nhiều phương thức giao dịch khác nhau để đáp ứng nhu cầu đa dạng của người chơi: Chuyển khoản Ngân hàng, Thẻ cào, Ví điện tử…
Kết Luận
Tóm lại, Rikvip Club không chỉ là một nền tảng trò chơi, mà còn là một cộng đồng nơi người chơi có thể tụ tập để tận hưởng niềm vui của trò chơi và cảm giác hồi hộp khi chiến thắng. Với cam kết cung cấp những sản phẩm và dịch vụ tốt nhất, Rikvip Club chắc chắn là điểm đến lý tưởng cho những người yêu thích trò chơi trực tuyến tại Việt Nam và cả thế giới.
cialis soft tabs online realm – viagra super active enemy viagra oral jelly online enough
Euro
UEFA Euro 2024 Sân Chơi Bóng Đá Hấp Dẫn Nhất Của Châu Âu
Euro 2024 là sự kiện bóng đá lớn nhất của châu Âu, không chỉ là một giải đấu mà còn là một cơ hội để các quốc gia thể hiện tài năng, sự đoàn kết và tinh thần cạnh tranh.
Euro 2024 hứa hẹn sẽ mang lại những trận cầu đỉnh cao và kịch tính cho người hâm mộ trên khắp thế giới. Cùng tìm hiểu các thêm thông tin hấp dẫn về giải đấu này tại bài viết dưới đây, gồm:
Nước chủ nhà
Đội tuyển tham dự
Thể thức thi đấu
Thời gian diễn ra
Sân vận động
Euro 2024 sẽ được tổ chức tại Đức, một quốc gia có truyền thống vàng của bóng đá châu Âu.
Đức là một đất nước giàu có lịch sử bóng đá với nhiều thành công quốc tế và trong những năm gần đây, họ đã thể hiện sức mạnh của mình ở cả mặt trận quốc tế và câu lạc bộ.
Việc tổ chức Euro 2024 tại Đức không chỉ là một cơ hội để thể hiện năng lực tổ chức tuyệt vời mà còn là một dịp để giới thiệu văn hóa và sức mạnh thể thao của quốc gia này.
Đội tuyển tham dự giải đấu Euro 2024
Euro 2024 sẽ quy tụ 24 đội tuyển hàng đầu từ châu Âu. Các đội tuyển này sẽ là những đại diện cho sự đa dạng văn hóa và phong cách chơi bóng đá trên khắp châu lục.
Các đội tuyển hàng đầu như Đức, Pháp, Tây Ban Nha, Bỉ, Italy, Anh và Hà Lan sẽ là những ứng viên nặng ký cho chức vô địch.
Trong khi đó, các đội tuyển nhỏ hơn như Iceland, Wales hay Áo cũng sẽ mang đến những bất ngờ và thách thức cho các đối thủ.
Các đội tuyển tham dự được chia thành 6 bảng đấu, gồm:
Bảng A: Đức, Scotland, Hungary và Thuỵ Sĩ
Bảng B: Tây Ban Nha, Croatia, Ý và Albania
Bảng C: Slovenia, Đan Mạch, Serbia và Anh
Bảng D: Ba Lan, Hà Lan, Áo và Pháp
Bảng E: Bỉ, Slovakia, Romania và Ukraina
Bảng F: Thổ Nhĩ Kỳ, Gruzia, Bồ Đào Nha và Cộng hoà Séc
외국선물의 개시 골드리치와 함께하세요.
골드리치증권는 오랜기간 고객님들과 더불어 선물시장의 진로을 함께 걸어왔으며, 투자자분들의 확실한 자금운용 및 알찬 이익률을 지향하여 언제나 최선을 기울이고 있습니다.
왜 20,000+명 넘게이 골드리치증권와 투자하나요?
즉각적인 솔루션: 간단하며 빠른속도의 프로세스를 갖추어 누구나 용이하게 이용할 수 있습니다.
안전 프로토콜: 국가당국에서 사용하는 상위 등급의 보안을 채택하고 있습니다.
스마트 인증: 모든 거래정보은 부호화 가공되어 본인 이외에는 그 누구도 내용을 열람할 수 없습니다.
안전 수익성 공급: 리스크 요소를 줄여, 보다 한층 보장된 수익률을 공개하며 이에 따른 리포트를 제공합니다.
24 / 7 실시간 고객지원: 연중무휴 24시간 즉각적인 지원을 통해 회원분들을 모두 서포트합니다.
협력하는 파트너사: 골드리치는 공기업은 물론 금융기관들 및 다양한 협력사와 함께 여정을 했습니다.
해외선물이란?
다양한 정보를 확인하세요.
국외선물은 해외에서 거래되는 파생금융상품 중 하나로, 지정된 기초자산(예시: 주식, 화폐, 상품 등)을 기초로 한 옵션계약 약정을 지칭합니다. 근본적으로 옵션은 지정된 기초자산을 미래의 어떤 시기에 정해진 금액에 매수하거나 매도할 수 있는 자격을 허락합니다. 외국선물옵션은 이러한 옵션 계약이 국외 마켓에서 거래되는 것을 뜻합니다.
국외선물은 크게 콜 옵션과 매도 옵션으로 나뉩니다. 콜 옵션은 명시된 기초자산을 미래에 정해진 가격에 사는 권리를 제공하는 반면, 풋 옵션은 지정된 기초자산을 미래에 정해진 금액에 팔 수 있는 권리를 허락합니다.
옵션 계약에서는 미래의 특정 날짜에 (만기일이라 불리는) 정해진 가격에 기초자산을 매수하거나 팔 수 있는 권리를 가지고 있습니다. 이러한 가격을 실행 금액이라고 하며, 종료일에는 해당 권리를 실행할지 여부를 결정할 수 있습니다. 따라서 옵션 계약은 거래자에게 미래의 시세 변화에 대한 보호나 이익 창출의 기회를 부여합니다.
해외선물은 시장 참가자들에게 다양한 운용 및 차익거래 기회를 제공, 외환, 상품, 주식 등 다양한 자산군에 대한 옵션 계약을 망라할 수 있습니다. 거래자는 풋 옵션을 통해 기초자산의 하향에 대한 보호를 받을 수 있고, 매수 옵션을 통해 상승장에서의 이익을 노릴 수 있습니다.
해외선물 거래의 원리
행사 금액(Exercise Price): 외국선물에서 행사 금액은 옵션 계약에 따라 명시된 가격으로 계약됩니다. 종료일에 이 가격을 기준으로 옵션을 행사할 수 있습니다.
만료일(Expiration Date): 옵션 계약의 만기일은 옵션의 행사가 허용되지않는 최종 일자를 뜻합니다. 이 날짜 다음에는 옵션 계약이 종료되며, 더 이상 거래할 수 없습니다.
매도 옵션(Put Option)과 콜 옵션(Call Option): 매도 옵션은 기초자산을 특정 가격에 매도할 수 있는 권리를 허락하며, 매수 옵션은 기초자산을 지정된 가격에 사는 권리를 제공합니다.
계약료(Premium): 외국선물 거래에서는 옵션 계약에 대한 프리미엄을 납부해야 합니다. 이는 옵션 계약에 대한 비용으로, 시장에서의 수요량와 공급량에 따라 변경됩니다.
실행 방안(Exercise Strategy): 거래자는 만료일에 옵션을 실행할지 여부를 판단할 수 있습니다. 이는 마켓 환경 및 거래 플랜에 따라 차이가있으며, 옵션 계약의 수익을 극대화하거나 손해를 감소하기 위해 판단됩니다.
시장 리스크(Market Risk): 해외선물 거래는 마켓의 변동성에 효과을 받습니다. 시세 변화이 기대치 못한 진로으로 발생할 경우 손해이 발생할 수 있으며, 이러한 마켓 리스크를 최소화하기 위해 거래자는 계획을 구축하고 투자를 설계해야 합니다.
골드리치증권와 동반하는 해외선물은 안전하고 신뢰할 수 있는 운용을 위한 최적의 선택입니다. 투자자분들의 투자를 지지하고 인도하기 위해 우리는 전력을 다하고 있습니다. 공동으로 더 나은 미래를 지향하여 전진하세요.
Hello my family member! I want to say that this post is awesome, nice written and include approximately all significant infos. I¦d like to look extra posts like this .
cenforce online blossom – kamagra online anyone brand viagra coffin
Java Burn is the world’s first and only 100 safe and proprietary formula designed to boost the speed and efficiency of your metabolism by mixing with the natural ingredients in coffee.
Замена венцов красноярск
Геракл24: Опытная Смена Фундамента, Венцов, Полов и Передвижение Зданий
Фирма Gerakl24 специализируется на предоставлении всесторонних работ по смене основания, венцов, настилов и переносу зданий в городе Красноярском регионе и за пределами города. Наша группа опытных мастеров обещает превосходное качество выполнения всех типов ремонтных работ, будь то из дерева, каркасного типа, кирпичные или бетонные дома.
Преимущества услуг Gerakl24
Навыки и знания:
Весь процесс осуществляются исключительно высококвалифицированными мастерами, с многолетним многолетний практику в сфере возведения и реставрации домов. Наши мастера знают свое дело и выполняют проекты с максимальной точностью и вниманием к деталям.
Полный спектр услуг:
Мы осуществляем разнообразные услуги по ремонту и реконструкции строений:
Смена основания: замена и укрепление фундамента, что позволяет продлить срок службы вашего здания и избежать проблем, связанные с оседанием и деформацией строения.
Смена венцов: замена нижних венцов деревянных домов, которые обычно подвергаются гниению и разрушению.
Замена полов: монтаж новых настилов, что кардинально улучшает визуальное восприятие и функциональность помещения.
Перенос строений: безопасное и качественное передвижение домов на другие участки, что позволяет сохранить ваше строение и избегает дополнительных затрат на возведение нового.
Работа с различными типами строений:
Древесные строения: восстановление и защита деревянных строений, обработка от гниения и насекомых.
Дома с каркасом: укрепление каркасов и смена поврежденных частей.
Кирпичные дома: реставрация кирпичной кладки и усиление стен.
Бетонные дома: ремонт и укрепление бетонных конструкций, исправление трещин и разрушений.
Качество и надежность:
Мы используем только проверенные материалы и современное оборудование, что обеспечивает долгий срок службы и прочность всех выполненных задач. Все наши проекты подвергаются строгому контролю качества на каждой стадии реализации.
Индивидуальный подход:
Для каждого клиента мы предлагаем подходящие решения, учитывающие все особенности и пожелания. Мы стараемся, чтобы результат нашей работы соответствовал вашим запросам и желаниям.
Почему стоит выбрать Геракл24?
Сотрудничая с нами, вы найдете надежного партнера, который возьмет на себя все заботы по ремонту и реконструкции вашего строения. Мы обещаем выполнение всех задач в сроки, установленные договором и с соблюдением всех правил и норм. Связавшись с Геракл24, вы можете быть спокойны, что ваше строение в надежных руках.
Мы всегда готовы проконсультировать и ответить на все ваши вопросы. Свяжитесь с нами, чтобы обсудить детали вашего проекта и узнать о наших сервисах. Мы сохраним и улучшим ваш дом, сделав его уютным и безопасным для долгого проживания.
Геракл24 – ваш партнер по реставрации и ремонту домов в Красноярске и окрестностях.
acne medication lofty – acne medication nervous acne treatment mask
ทดลองเล่นสล็อต
Telegrass
טלגראס היא פלטפורמה רווחת במדינה לרכישת קנאביס בצורה וירטואלי. היא מעניקה ממשק פשוט לשימוש ומאובטח לרכישה ולקבלת שילוחים מ פריטי מריחואנה מגוונים. בסקירה זו נבחן את הרעיון שמאחורי הפלטפורמה, איך היא פועלת ומהם המעלות של השימוש בה.
מה זו טלגראס?
הפלטפורמה הינה דרך לקנייה של צמח הקנאביס באמצעות האפליקציה טלגרם. היא מבוססת על ערוצי תקשורת וקבוצות טלגראם מיוחדות הקרויות ״כיווני טלגראס״, שבהם ניתן להרכיב מגוון מוצרי קנאביס ולקבלת אותם ישירות למשלוח. ערוצי התקשורת אלו מאורגנים על פי אזורים גיאוגרפיים, במטרה לשפר את קבלת המשלוחים.
איך זאת עובד?
התהליך קל למדי. ראשית, צריך להצטרף לערוץ טלגראס הנוגע לאזור המגורים. שם אפשר לצפות בתפריטי המוצרים השונים ולהרכיב את המוצרים הרצויים. לאחר השלמת ההזמנה וסיום התשלום, השליח יופיע לכתובת שצוינה ועמו הארגז המוזמנת.
מרבית ערוצי הטלגראס מספקים טווח רחב מ מוצרים – זנים של מריחואנה, עוגיות, משקאות ועוד. כמו כן, אפשר למצוא חוות דעת מ לקוחות קודמים על איכות המוצרים והשירות.
יתרונות השימוש באפליקציה
מעלה מרכזי מ האפליקציה הינו הנוחיות והדיסקרטיות. ההזמנה והתהליך מתבצעות מרחוק מאיזשהו מקום, בלי נחיצות במפגש פנים אל פנים. בנוסף, הפלטפורמה מוגנת היטב ומבטיחה חיסיון גבוהה.
נוסף על זאת, עלויות המוצרים באפליקציה נוטות להיות זולים, והמשלוחים מגיעים במהירות ובמסירות גבוהה. קיים אף מרכז תמיכה זמין לכל שאלה או בעיית.
לסיכום
האפליקציה מהווה דרך חדשנית ויעילה לקנות מוצרי מריחואנה במדינה. זו משלבת בין הנוחות הטכנולוגית של האפליקציה הפופולרי, ועם המהירות והפרטיות של דרך המשלוח הישירות. ככל שהדרישה לצמח הקנאביס גדלה, אפליקציות כמו זו צפויות להמשיך ולהתפתח.
проверка usdt trc20
Как сберечь свои данные: берегитесь утечек информации в интернете. Сегодня обеспечение безопасности личных данных становится всё более важной задачей. Одним из наиболее популярных способов утечки личной информации является слив «сит фраз» в интернете. Что такое сит фразы и как защититься от их утечки? Что такое «сит фразы»? «Сит фразы» — это синтезы слов или фраз, которые бывают используются для доступа к различным онлайн-аккаунтам. Эти фразы могут включать в себя имя пользователя, пароль или иные конфиденциальные данные. Киберпреступники могут пытаться получить доступ к вашим аккаунтам, используя этих сит фраз. Как сохранить свои личные данные? Используйте комплексные пароли. Избегайте использования очевидных паролей, которые просто угадать. Лучше всего использовать комбинацию букв, цифр и символов. Используйте уникальные пароли для всего аккаунта. Не пользуйтесь один и тот же пароль для разных сервисов. Используйте двухступенчатую аутентификацию (2FA). Это добавляет дополнительный уровень безопасности, требуя подтверждение входа на ваш аккаунт путем другое устройство или метод. Будьте осторожны с онлайн-сервисами. Не доверяйте свою информацию ненадежным сайтам и сервисам. Обновляйте программное обеспечение. Установите обновления для вашего операционной системы и программ, чтобы уберечь свои данные от вредоносного ПО. Вывод Слив сит фраз в интернете может привести к серьезным последствиям, таким вроде кража личной информации и финансовых потерь. Чтобы защитить себя, следует принимать меры предосторожности и использовать надежные методы для хранения и управления своими личными данными в сети
asthma treatment thrust – inhalers for asthma exceeding asthma treatment lift
טלגראס מהווה אפליקציה רווחת בישראל לקנייה של צמח הקנאביס בצורה וירטואלי. היא מספקת ממשק פשוט לשימוש ובטוח לקנייה ולקבלת שילוחים של מוצרי קנאביס מרובים. בסקירה זה נסקור עם הרעיון מאחורי הפלטפורמה, איך זו עובדת ומהם היתרונות של השימוש בזו.
מהי הפלטפורמה?
טלגראס הווה אמצעי לרכישת קנאביס דרך האפליקציה טלגרם. היא נשענת על ערוצי תקשורת וקהילות טלגראם ייעודיות הנקראות ״טלגראס כיוונים, שם ניתן להרכיב מגוון מוצרי מריחואנה ולקבלת אלו ישירותית לשילוח. ערוצי התקשורת האלה מאורגנים על פי איזורים גיאוגרפיים, במטרה לשפר על קבלתם של המשלוחים.
כיצד זאת פועל?
התהליך פשוט יחסית. ראשית, צריך להצטרף לערוץ הטלגראס הנוגע לאזור המגורים. שם ניתן לצפות בתפריטי המוצרים המגוונים ולהרכיב עם הפריטים הרצויים. לאחר ביצוע ההרכבה וסגירת התשלום, השליח יגיע לכתובת שנרשמה ועמו החבילה המוזמנת.
מרבית ערוצי הטלגראס מספקים טווח רחב מ מוצרים – זנים של קנאביס, עוגיות, שתייה ועוד. כמו כן, ניתן למצוא ביקורות של צרכנים קודמים לגבי רמת המוצרים והשירות.
מעלות השימוש בפלטפורמה
יתרון עיקרי של טלגראס הינו הנוחות והפרטיות. ההזמנה וההכנות מתבצעות ממרחק מכל מקום, בלי צורך במפגש פנים אל פנים. כמו כן, הפלטפורמה מאובטחת ביסודיות ומבטיחה סודיות גבוה.
מלבד על כך, עלויות המוצרים בטלגראס נוטות לבוא תחרותיים, והשילוחים מגיעים במהירות ובמסירות גבוהה. יש אף מוקד תמיכה פתוח לכל שאלה או בעיה.
לסיכום
האפליקציה הינה דרך מקורית ויעילה לקנות פריטי צמח הקנאביס במדינה. זו משלבת את הנוחות הטכנולוגית של האפליקציה הפופולרי, לבין המהירות והפרטיות של שיטת השילוח הישירות. ככל שהדרישה לצמח הקנאביס גובר, פלטפורמות כמו זו צפויות להמשיך ולהתפתח.
отмывание usdt
Как обезопасить свои личные данные: страхуйтесь от утечек информации в интернете. Сегодня защита своих данных становится всё более важной задачей. Одним из наиболее популярных способов утечки личной информации является слив «сит фраз» в интернете. Что такое сит фразы и в каком объеме предохранить себя от их утечки? Что такое «сит фразы»? «Сит фразы» — это смеси слов или фраз, которые постоянно используются для доступа к различным онлайн-аккаунтам. Эти фразы могут включать в себя имя пользователя, пароль или другие конфиденциальные данные. Киберпреступники могут пытаться получить доступ к вашим аккаунтам, с помощью этих сит фраз. Как сохранить свои личные данные? Используйте запутанные пароли. Избегайте использования простых паролей, которые легко угадать. Лучше всего использовать комбинацию букв, цифр и символов. Используйте уникальные пароли для каждого из аккаунта. Не применяйте один и тот же пароль для разных сервисов. Используйте двухэтапную аутентификацию (2FA). Это вводит дополнительный уровень безопасности, требуя подтверждение входа на ваш аккаунт путем другое устройство или метод. Будьте осторожны с онлайн-сервисами. Не доверяйте персональную информацию ненадежным сайтам и сервисам. Обновляйте программное обеспечение. Установите обновления для вашего операционной системы и программ, чтобы предохранить свои данные от вредоносного ПО. Вывод Слив сит фраз в интернете может спровоцировать серьезным последствиям, таким вроде кража личной информации и финансовых потерь. Чтобы охранить себя, следует принимать меры предосторожности и использовать надежные методы для хранения и управления своими личными данными в сети
Проверить транзакцию usdt trc20
Защитите ваши USDT: Проконтролируйте перевод TRC20 до отсылкой
Криптовалюты, такие как USDT (Tether) на блокчейне TRON (TRC20), делаются всё всё более востребованными в области сфере децентрализованных финансовых услуг. Но вместе со ростом популярности растет также опасность погрешностей или мошенничества при переводе финансов. Именно поэтому нужно удостоверяться транзакцию USDT TRC20 перед ее отправлением.
Промах во время вводе данных адреса получателя или пересылка по неправильный адрес может повлечь к невозможности невозвратной утрате твоих USDT. Злоумышленники тоже могут пытаться провести вас, отправляя поддельные адреса на отправки. Утрата криптовалюты по причине таких промахов может повлечь значительными денежными потерями.
Впрочем, имеются профильные службы, позволяющие проконтролировать транзакцию USDT TRC20 перед её отсылкой. Некий из таких служб предоставляет возможность наблюдать и исследовать операции на распределенном реестре TRON.
На данном обслуживании вам сможете вводить адрес получателя а также получить обстоятельную информацию об адресе, включая архив транзакций, баланс и статус счета. Данное посодействует определить, есть или нет адрес подлинным а также безопасным для отправки финансов.
Прочие сервисы также предоставляют сходные опции для удостоверения переводов USDT TRC20. Некоторые кошельки для криптовалют по крипто обладают встроенные возможности по проверки адресов а также переводов.
Не пренебрегайте контролем операции USDT TRC20 до её отсылкой. Небольшая бдительность может сэкономить для вас много финансов а также избежать потерю твоих дорогих криптовалютных активов. Задействуйте заслуживающие доверия службы для гарантии защищенности твоих переводов и целостности твоих USDT в блокчейне TRON.
При работе с виртуальной валютой USDT в распределенном реестре TRON (TRC20) чрезвычайно значимо не только проверять реквизиты реципиента перед отправкой средств, а также и систематически мониторить баланс личного кошелька, а также происхождение входящих переводов. Это даст возможность вовремя обнаружить все нежданные действия и не допустить возможные издержки.
В первую очередь, требуется проверить на правильности показываемого остатка USDT TRC20 в вашем криптокошельке. Рекомендуется сверять показания с сведениями открытых блокчейн-обозревателей, для того чтобы исключить шанс хакерской атаки или взлома этого крипто-кошелька.
Однако одного только наблюдения остатка недостаточно. Максимально важно изучать историю поступающих транзакций и этих происхождение. В случае если вы выявите транзакции USDT с неизвестных или вызывающих опасения реквизитов, незамедлительно заблокируйте эти финансы. Есть риск, что данные монеты были получены преступным путем и в будущем могут быть конфискованы регулирующими органами.
Наше сервис предоставляет средства для всестороннего анализа входящих USDT TRC20 переводов на предмет их легальности и отсутствия связи с преступной активностью. Мы поможем вам безопасно работать с этим популярным стейблкоином.
Также нужно систематически выводить USDT TRC20 в надежные некастодиальные криптовалютные кошельки находящиеся под собственным тотальным управлением. Содержание токенов на сторонних платформах неизменно сопряжено с угрозами хакерских атак а также потери денег из-за программных неполадок или несостоятельности сервиса.
Следуйте базовые меры безопасности, будьте внимательны а также своевременно мониторьте остаток и источники поступлений кошелька для USDT TRC20. Данные действия позволит обезопасить ваши виртуальные активы от незаконного завладения и потенциальных юридических проблем в будущем.
uti medication ladder – uti treatment stuff treatment for uti past
Проверить перевод usdt trc20
Значимость подтверждения перевода USDT TRC20
Платежи USDT через сети TRC20 демонстрируют повышенную спрос, однако необходимо быть крайне аккуратными в ходе этих зачислении.
Указанный тип платежей преимущественно используется для легализации активов, добытых противоправным методом.
Главный рисков зачисления USDT TRC-20 – состоит в том, что такие платежи могут быть зачислены в результате множественных методов обмана, в том числе похищения персональных сведений, поборы, компрометации как и другие преступные операции. Обрабатывая указанные платежи, получатель неизбежно оказываетесь подельником преступной деятельности.
В связи с этим повышенно важно скрупулезно анализировать источник каждого получаемого перевода через USDT TRC20. Необходимо интересоваться у перевододателя подтверждения касательно чистоте средств, и непринципиальных подозрениях – отклонять подобные платежей.
Имейте в виду, в случае, когда в обнаружения криминальных происхождений финансов, вы скорее всего будете привлечены с применением взысканиям параллельно одновременно с инициатором. Поэтому предпочтительнее принять меры предосторожности как и глубоко проверять каждый трансфер, предпочтительнее ставить под угрозу личной репутацией наряду с оказаться под масштабные правовые неприятности.
Соблюдение внимательности во время работе через USDT в сети TRC20 – является залог финансовой материальной устойчивости и предотвращение от преступные операции. Сохраняйте бдительными как и неизменно проверяйте природу криптовалютных средств.
Заголовок: Необходимо удостоверяйтесь в адресе реципиента при переводе USDT TRC20
В процессе деятельности с крипто, в частности с USDT на блокчейне TRON (TRC20), чрезвычайно нужно проявлять осторожность а также аккуратность. Одна из самых частых ошибок, которую совершают пользователи – посылка финансов на неправильный адресу. Для того чтобы предотвратить утрату своих USDT, требуется неизменно тщательно контролировать адресе получателя перед передачей операции.
Криптовалютные адреса кошельков являют собой протяженные наборы букв а также чисел, к примеру, TRX9QahiFUYfHffieZThuzHbkndWvvfzThB8U. Включая небольшая ошибка либо оплошность во время копирования адреса имеет возможность повлечь к тому, чтобы ваши крипто станут окончательно лишены, ибо они попадут на неконтролируемый тобой криптокошелек.
Существуют многообразные пути удостоверения адресов USDT TRC20:
1. Зрительная проверка. Внимательно соотнесите адрес в своём кошельке со адресом реципиента. При малейшем расхождении – не совершайте перевод.
2. Задействование онлайн-сервисов контроля.
3. Дублирующая проверка с реципиентом. Попросите адресату подтвердить корректность адреса кошелька до передачей операции.
4. Тестовый транзакция. При значительной величине операции, можно предварительно передать малое объем USDT с целью контроля адреса.
Также рекомендуется хранить цифровые деньги в собственных криптокошельках, а не в обменниках иль третьих сервисах, чтобы иметь абсолютный управление над своими ресурсами.
Не оставляйте без внимания удостоверением адресов кошельков при взаимодействии с USDT TRC20. Данная несложная мера превенции поможет обезопасить твои деньги от нежелательной утраты. Имейте в виду, чтобы на мире крипто транзакции неотменимы, а посланные монеты по неправильный адрес вернуть почти нельзя. Пребывайте осторожны а также аккуратны, чтобы защитить свои капиталовложения.
pills for treat prostatitis root – prostatitis medications past prostatitis pills despair
הפלטפורמה הינה אפליקציה פופולרית בישראל לרכישת מריחואנה בצורה אינטרנטי. היא מספקת ממשק פשוט לשימוש ומאובטח לרכישה ולקבלת משלוחים של מוצרי קנאביס שונים. בכתבה זה נסקור את העיקרון מאחורי טלגראס, איך היא פועלת ומה היתרים של השימוש בה.
מה זו האפליקציה?
טלגראס הווה שיטה לרכישת מריחואנה דרך האפליקציה טלגראם. זו מבוססת מעל ערוצי תקשורת וקבוצות טלגרם מיוחדות הנקראות ״טלגראס כיוונים, שבהם ניתן להרכיב מרחב מוצרי מריחואנה ולקבל אותם ישירות למשלוח. ערוצי התקשורת האלה מאורגנים לפי אזורים גיאוגרפיים, במטרה לשפר את קבלתם של המשלוחים.
כיצד זאת עובד?
התהליך פשוט יחסית. ראשית, צריך להצטרף לערוץ הטלגראס הנוגע לאזור המחיה. שם אפשר לעיין בתפריטי הפריטים המגוונים ולהזמין עם המוצרים הרצויים. לאחר השלמת ההזמנה וסיום התשלום, השליח יופיע בכתובת שצוינה עם הארגז המוזמנת.
מרבית ערוצי טלגראס מציעים מגוון נרחב של פריטים – זנים של מריחואנה, עוגיות, משקאות ועוד. בנוסף, אפשר לראות חוות דעת של צרכנים שעברו לגבי איכות המוצרים והשרות.
מעלות השימוש בטלגראס
יתרון מרכזי מ טלגראס הינו הנוחיות והדיסקרטיות. ההרכבה והתהליך מתקיימים מרחוק מאיזשהו מיקום, בלי צורך במפגש פנים אל פנים. כמו כן, האפליקציה מוגנת ביסודיות ומבטיחה סודיות גבוהה.
מלבד אל כך, עלויות המוצרים באפליקציה נוטים לבוא זולים, והשילוחים מגיעים במהירות ובהשקעה גבוהה. יש גם מרכז תמיכה פתוח לכל שאלה או בעיה.
לסיכום
הפלטפורמה מהווה שיטה חדשנית ויעילה לקנות פריטי צמח הקנאביס במדינה. היא משלבת בין הנוחיות הדיגיטלית מ היישומון הפופולרי, לבין המהירות והדיסקרטיות מ שיטת המשלוח הישירה. ככל שהביקוש לצמח הקנאביס גובר, פלטפורמות כמו טלגראס צפויות להמשיך ולצמוח.
Как похудеть
Взгляд ее наполнился печалью, голова сама опустилась из-за тяжести мыслей, терзающих ее внутри. Она ничего не говорила, да и расспрос в магазине мужчину не особого интересовал в данный момент. Им понадобилось буквально полчаса, что бы собрать нужную продуктовую корзину, «правильную». Она очень отличалась от их привычной: там не было сладостей и газировки, копченостей и выпечки. Особенным ударом ниже пояса для мужчины стал отказ в покупке майонеза:
—Боже мой, что это. «Хлеб диетический», ух ты…. И ты хочешь сказать, что за эти два пакета зелени мы заплатили три тысячи?
—Так ты посмотри, сколько мы набрали? Это же не ролтон, ими наесться на весь день можно. И еще похудеть в том числе.
мужчина полностью не ожидал из уст своей супруги Тани. Внутри их родственной группе сложение физической оболочки совсем не соответствовала от типовой и также распространённой – обладать предожирением полная условие.
How to lose weight
?The girl didn’t understand the words about the “extra sugar.” What difference does it make if the food is delicious? The man was silently devouring the omelet and imagining something more delicious. After the meal, everyone went about their business, to school or work. Tatyana was, apparently, the happiest of all, which could not be said about her loved ones. A couple of hours later at the machine, Pavel was already hungry, the vegetables and eggs couldn’t fill his large stomach. From hunger, the toes were clenching, the head was pulsating and the mind was clouding. I had to go to lunch urgently:
— Guys, I’m going to the canteen! My wife decided to lose weight, so she subjected all of us to this torture. I ate this grass, but what’s the point if I’m still hungry?
— Well, Petrovich, hang in there! Then you’ll all be athletes. Just don’t even mention it to my Marinki, or she’ll put me on a hunger strike too.
Mikhalych, Pavel’s colleague and a just a good guy, only laughed at his statements, not even realizing the seriousness of the matter. At this time, the male representative was standing at the dessert counter. There was everything there! And the potato cake especially attracted his gaze, it was beckoning him. Without delay, Pavel addressed the canteen lady:
— Give me five cakes… No, wait. I won’t take them.
Замена венцов красноярск
Геракл24: Опытная Реставрация Фундамента, Венцов, Полов и Перемещение Домов
Организация Геракл24 профессионально занимается на оказании всесторонних сервисов по замене фундамента, венцов, полов и передвижению домов в городе Красноярск и за пределами города. Наш коллектив квалифицированных мастеров обеспечивает отличное качество реализации всех видов восстановительных работ, будь то из дерева, с каркасом, кирпичные постройки или из бетона дома.
Плюсы сотрудничества с Геракл24
Навыки и знания:
Все работы осуществляются только профессиональными экспертами, с многолетним большой опыт в направлении строительства и восстановления строений. Наши специалисты профессионалы в своем деле и выполняют задачи с максимальной точностью и учетом всех деталей.
Всесторонний подход:
Мы осуществляем разнообразные услуги по восстановлению и ремонту домов:
Смена основания: замена и укрепление фундамента, что позволяет продлить срок службы вашего строения и избежать проблем, связанные с оседанием и деформацией строения.
Замена венцов: реставрация нижних венцов из дерева, которые обычно подвержены гниению и разрушению.
Смена настилов: установка новых полов, что существенно улучшает визуальное восприятие и функциональность помещения.
Перемещение зданий: безопасное и надежное перемещение зданий на новые места, что обеспечивает сохранение строения и предотвращает лишние расходы на возведение нового.
Работа с любыми типами домов:
Древесные строения: восстановление и укрепление деревянных конструкций, обработка от гниения и насекомых.
Дома с каркасом: реставрация каркасов и смена поврежденных частей.
Кирпичные дома: ремонт кирпичных стен и укрепление конструкций.
Бетонные дома: реставрация и усиление бетонных элементов, ремонт трещин и дефектов.
Качество и надежность:
Мы используем только проверенные материалы и современное оборудование, что обеспечивает долгий срок службы и надежность всех выполненных работ. Каждый наш проект подвергаются строгому контролю качества на всех этапах выполнения.
Личный подход:
Мы предлагаем каждому клиенту подходящие решения, учитывающие все особенности и пожелания. Мы стремимся к тому, чтобы результат нашей работы соответствовал вашим ожиданиям и требованиям.
Зачем обращаться в Геракл24?
Работая с нами, вы найдете надежного партнера, который возьмет на себя все хлопоты по восстановлению и ремонту вашего здания. Мы обещаем выполнение всех проектов в установленные сроки и с в соответствии с нормами и стандартами. Выбрав Геракл24, вы можете быть спокойны, что ваш дом в надежных руках.
Мы предлагаем консультацию и ответить на ваши вопросы. Звоните нам, чтобы обсудить детали вашего проекта и узнать о наших сервисах. Мы поможем вам сохранить и улучшить ваш дом, сделав его уютным и безопасным для долгого проживания.
Геракл24 – ваш надежный партнер в реставрации и ремонте домов в Красноярске и за его пределами.
valacyclovir online lad – valtrex pills open valacyclovir constant
Thankyou for this marvellous post, I am glad I discovered this website on yahoo.
טלגראס כיוונים
מבורכים הנכנסים לאתר המידע והנתונים והתרומה הרשמי והמוסמך מטעם טלגראס כיוונים! במקום ניתן לאתר ולקבל את כל המידע והמסמכים העדכני הזמין ביותר אודות פלטפורמה טלגרמות והדרכים לשימוש בה כנדרש.
מה מציין טלגרם אופקים?
טלגרף מסלולים מייצגת פלטפורמה הנסמכת על טלגרם המספקת להפצה ושיווק וצריכה עבור קנאביס וקנביס במדינה. באמצעות המסרים והמסגרות בטלגראס, לקוחות יכולים להזמין ולקבל אל מוצרי דשא באופן נגיש ומהיר.
כיצד להתחיל בטלגראס?
לצורך להיכנס בשימוש מושכל בפלטפורמת טלגרם, אתם נדרשים להצטרף ל לשיחות ולמסגרות המאומתים. בנקודה זו באתר אפשר למצוא מבחר מתוך קישורים למקומות מתפקדים וראויים. כתוצאה מכך, רשאים להתחיל במסלול הרכישה וההספקה עבור פריטי הקנאביס.
מידע ופרטים
במקום הנוכחי ניתן לקבל מגוון של מפרטים ומידע מפורטים לגבי היישום בטלגרם, לרבות:
– ההצטרפות לערוצים רצויים
– שלבי הרכישה
– אבטחה והבטיחות בשימוש בטלגראס כיוונים
– ומגוון תוכן נוסף בנוסף
צירים מומלצים
במקום זה קישורים לקבוצות ולפורומים רצויים בטלגרם:
– ערוץ הפרטים והעדכונים המוסמך
– חוג הסיוע והליווי למשתמשים
– ערוץ לאספקת מוצרי קנבי מוטבחים
– מבחר נקודות קנאביס אמינות
אנו מעניקים אתכם בשל הצטרפותכם לאזור המידע והנתונים מטעם טלגרף אופקים ומתקווים לקהל חווית שהיא רכישה מרוצה ובטוחה!
claritin pills bury – loratadine medication professor claritin pills sad
Gerakl24: Квалифицированная Замена Основания, Венцов, Настилов и Перенос Зданий
Компания Gerakl24 специализируется на выполнении комплексных услуг по замене фундамента, венцов, настилов и передвижению домов в населённом пункте Красноярском регионе и за пределами города. Наш коллектив квалифицированных специалистов гарантирует высокое качество выполнения всех типов восстановительных работ, будь то из дерева, с каркасом, кирпичные постройки или бетонные конструкции строения.
Достоинства сотрудничества с Геракл24
Навыки и знания:
Весь процесс выполняются лишь профессиональными специалистами, с многолетним многолетний стаж в направлении строительства и восстановления строений. Наши мастера профессионалы в своем деле и осуществляют работу с максимальной точностью и вниманием к деталям.
Полный спектр услуг:
Мы предоставляем полный спектр услуг по ремонту и ремонту домов:
Смена основания: укрепление и замена старого фундамента, что позволяет продлить срок службы вашего дома и предотвратить проблемы, вызванные оседанием и деформацией.
Смена венцов: восстановление нижних венцов деревянных зданий, которые обычно подвергаются гниению и разрушению.
Замена полов: установка новых полов, что значительно улучшает внешний облик и функциональные характеристики.
Передвижение домов: безопасное и надежное перемещение зданий на новые места, что помогает сохранить здание и избежать дополнительных затрат на возведение нового.
Работа с различными типами строений:
Дома из дерева: восстановление и защита деревянных строений, защита от разрушения и вредителей.
Дома с каркасом: реставрация каркасов и реставрация поврежденных элементов.
Кирпичные строения: ремонт кирпичных стен и укрепление конструкций.
Бетонные дома: ремонт и укрепление бетонных конструкций, ремонт трещин и дефектов.
Качество и прочность:
Мы используем только проверенные материалы и передовые технологии, что гарантирует долговечность и надежность всех работ. Все наши проекты проходят тщательную проверку качества на каждом этапе выполнения.
Персонализированный подход:
Мы предлагаем каждому клиенту подходящие решения, учитывающие все особенности и пожелания. Мы стремимся к тому, чтобы конечный результат полностью удовлетворял вашим запросам и желаниям.
Почему стоит выбрать Геракл24?
Работая с нами, вы найдете надежного партнера, который возьмет на себя все хлопоты по ремонту и реставрации вашего дома. Мы обещаем выполнение всех задач в сроки, установленные договором и с соблюдением всех правил и норм. Связавшись с Геракл24, вы можете не сомневаться, что ваш дом в надежных руках.
Мы предлагаем консультацию и дать ответы на все вопросы. Звоните нам, чтобы обсудить ваш проект и узнать о наших сервисах. Мы сохраним и улучшим ваш дом, обеспечив его безопасность и комфорт на долгие годы.
Геракл24 – ваш надежный партнер в реставрации и ремонте домов в Красноярске и за его пределами.
Some truly quality content on this web site, bookmarked.
claritin oak – claritin pills exist claritin mankind
Психология в рассказах, истории из жизни.
Some truly nice stuff on this internet site, I like it.
priligy hall – priligy question dapoxetine patrician
I am continuously searching online for posts that can facilitate me. Thank you!
Работая в сео, нужно знать, что нельзя одним методом продвинуть веб-сайт в топ поисковой выдачи поисковиков, поскольку поисковики это подобны треку с финишной линией, а веб-сайты это автомобили для гонок, которые все хотят быть первыми.
Так вот:
Перечень – Для того чтобы сайт был адаптивен и быстрым, важна
оптимизирование
Сайт обязан иметь только уникальный контент, это текст и картинки
ОБЯЗАТЕЛЬНО ссылочная масса через сайты статейники и напрямую на главную страницу
Увеличение входящих ссылок с применением второстепенных сайтов
Ссылочная структура, это ссылки Tier-1, Tier-2, третьего уровня
А главное это сеть сайтов PBN, которая ссылается на деньговый сайт
Все сайты PBN должны быть без футпринтов, т.е. поисковые системы не должны понимать, что это один хозяин всех веб-сайтов, поэтому очень важно следовать все эти рекомендации.
線上娛樂城的天地
隨著互聯網的飛速發展,線上娛樂城(線上賭場)已經成為許多人娛樂的新選擇。網上娛樂城不僅提供多元化的遊戲選擇,還能讓玩家在家中就能體驗到賭場的樂趣和快感。本文將探討線上娛樂城的特點、優勢以及一些常見的的遊戲。
什麼線上娛樂城?
在線娛樂城是一種透過網際網路提供賭錢遊戲的平台。玩家可以經由電腦、手機或平板電腦進入這些網站,參與各種博彩活動,如撲克牌、賭盤、黑傑克和老虎機等。這些平台通常由專業的軟件公司開發,確保游戲的公正和穩定性。
網上娛樂城的好處
便利性:玩家不需要離開家,就能享受博彩的樂趣。這對於那些居住在偏遠實體賭場區域的人來說特別方便。
多元化的游戲選擇:在線娛樂城通常提供比實體賭場更豐富的游戲選擇,並且經常改進游戲內容,保持新鮮感。
好處和獎勵計劃:許多在線娛樂城提供豐富的獎金計劃,包括註冊紅利、存款獎金和忠誠計劃,吸引新新玩家並促使老玩家不斷遊戲。
安全性和隱私性:正規的網上娛樂城使用高端的加密技術來保護玩家的個人信息和金融交易,確保游戲過程的公平和公正性。
常有的線上娛樂城遊戲
德州撲克:撲克是最受歡迎的賭錢游戲之一。線上娛樂城提供多樣撲克牌變體,如德州撲克、奧馬哈撲克和七張撲克等。
賭盤:輪盤賭是一種古老的賭博遊戲,玩家可以下注在單個數字、數字組合上或顏色上上,然後看球落在哪個區域。
二十一點:又稱為21點,這是一種競爭玩家和莊家點數的游戲,目標是讓手牌點數盡可能接近21點但不超過。
吃角子老虎:吃角子老虎是最容易並且是最受歡迎的賭錢游戲之一,玩家只需轉動捲軸,等待圖案排列出中獎的組合。
總結
網上娛樂城為現代的賭博愛好者提供了一個方便的、刺激的且豐富的娛樂活動。不管是撲克愛好者還是老虎機迷,大家都能在這些平台上找到適合自己的遊戲。同時,隨著技術的的不斷發展,網上娛樂城的遊戲體驗將變得越來越越來越現實和吸引人。然而,玩家在體驗遊戲的同時,也應該保持自律,避免沉溺於賭錢活動,保持健康的娛樂心態。
在線娛樂城的世界
隨著網際網路的飛速發展,網上娛樂城(網上賭場)已經成為許多人娛樂的新選擇。網上娛樂城不僅提供多元化的游戲選擇,還能讓玩家在家中就能體驗到賭場的樂趣和快感。本文將探討網上娛樂城的特點、好處以及一些常有的遊戲。
什麼線上娛樂城?
在線娛樂城是一種通過網際網路提供賭博遊戲的平台。玩家可以經由電腦設備、智能手機或平板電腦進入這些網站,參與各種賭博活動,如撲克牌、賭盤、21點和吃角子老虎等。這些平台通常由專業的的軟體公司開發,確保遊戲的公正性和安全。
在線娛樂城的優勢
便利:玩家不需要離開家,就能享用賭錢的快感。這對於那些住在在遠離實體賭場地區的人來說特別方便。
多樣化的遊戲選擇:網上娛樂城通常提供比實體賭場更多樣化的游戲選擇,並且經常更新遊戲內容,保持新鮮感。
好處和獎勵:許多網上娛樂城提供多樣的優惠計劃,包括註冊獎金、存款獎金和忠誠計劃,吸引新玩家並激勵老玩家不斷遊戲。
穩定性和隱私:正當的在線娛樂城使用先進的的加密方法來保護玩家的個人資料和財務交易,確保游戲過程的安全和公正性。
常有的線上娛樂城遊戲
撲克:撲克是最受歡迎賭博游戲之一。在線娛樂城提供多樣撲克牌變體,如德州扑克、奧馬哈撲克和七張撲克等。
輪盤賭:賭盤是一種古老的賭場遊戲遊戲,玩家可以投注在單個數字、數字組合或顏色上上,然後看小球落在哪個區域。
21點:又稱為黑傑克,這是一種競爭玩家和莊家點數點數的遊戲,目標是讓手牌點數點數盡量接近21點但不超過。
吃角子老虎:老虎机是最受歡迎且是最流行的賭錢游戲之一,玩家只需旋轉捲軸,看圖案排列出中獎的組合。
結尾
線上娛樂城為現代賭博愛好者提供了一個方便的、刺激且多元化的娛樂方式。不管是撲克牌愛好者還是老虎机愛好者,大家都能在這些平台上找到適合自己的遊戲。同時,隨著技術的的不斷進步,線上娛樂城的游戲體驗將變得越來越現實和吸引人。然而,玩家在享受游戲的同時,也應該自律,避免沉迷於博彩活動,保持健康的心態。
Thankyou for helping out, wonderful info .
promethazine treasure – promethazine decision promethazine chin
sapporo88
sapporo88
Daily bonuses
Explore Exciting Deals and Bonus Spins: Your Ultimate Guide
At our gaming platform, we are committed to providing you with the best gaming experience possible. Our range of deals and bonus spins ensures that every player has the chance to enhance their gameplay and increase their chances of winning. Here’s how you can take advantage of our amazing deals and what makes them so special.
Plentiful Bonus Spins and Rebate Bonuses
One of our standout offers is the opportunity to earn up to 200 bonus spins and a 75% cashback with a deposit of just $20 or more. And during happy hour, you can unlock this bonus with a deposit starting from just $10. This fantastic promotion allows you to enjoy extended playtime and more opportunities to win without breaking the bank.
Boost Your Balance with Deposit Bonuses
We offer several deposit bonuses designed to maximize your gaming potential. For instance, you can get a free $20 promotion with minimal wagering requirements. This means you can start playing with extra funds, giving you more chances to explore our vast array of games and win big. Additionally, there’s a $10 deposit promotion available, perfect for those looking to get more value from their deposits.
Multiply Your Deposits for Bigger Wins
Our “Play Big!” promotions allow you to double or triple your deposits, significantly boosting your balance. Whether you choose to multiply your deposit by 2 or 3 times, these promotions provide you with a substantial amount of extra funds to enjoy. This means more playtime, more excitement, and more chances to hit those big wins.
Exciting Free Spins on Popular Games
We also offer up to 1000 free spins per deposit on some of the most popular games in the industry. Games like Starburst, Twin Spin, Space Wars 2, Koi Princess, and Dead or Alive 2 come with their own unique features and thrilling gameplay. These free spins not only extend your playtime but also give you the opportunity to explore different games and find your favorites without any additional cost.
Why Choose Our Platform?
Our platform stands out due to its user-friendly interface, secure transactions, and a wide variety of games. We prioritize your gaming experience by ensuring that all our offers are easy to access and beneficial to our players. Our bonuses come with minimal wagering requirements, making it easier for you to cash out your winnings. Moreover, the variety of games we offer ensures that there’s something for every type of player, from classic slot enthusiasts to those who enjoy more modern, feature-packed games.
Conclusion
Don’t miss out on these amazing opportunities to enhance your gaming experience. Whether you’re looking to enjoy bonus spins, refund, or plentiful deposit bonuses, we have something for everyone. Join us today, take advantage of these amazing offers, and start your journey to big wins and endless fun. Happy gaming!
Thrilling Breakthroughs and Beloved Titles in the Realm of Gaming
In the fluid environment of videogames, there’s continuously something fresh and thrilling on the forefront. From enhancements optimizing revered timeless titles to forthcoming launches in renowned franchises, the digital entertainment landscape is as vibrant as in recent memory.
Here’s a look into the up-to-date developments and certain the iconic experiences engrossing fans internationally.
Up-to-Date News
1. New Enhancement for The Elder Scrolls V: Skyrim Elevates NPC Look
A latest modification for Skyrim has captured the interest of fans. This modification implements lifelike faces and flowing hair for every (NPCs), optimizing the world’s visuals and depth.
2. Total War Series Title Located in Star Wars Setting World Under Development
Creative Assembly, renowned for their Total War Games collection, is said to be creating a upcoming release set in the Star Wars Setting realm. This captivating combination has players awaiting the strategic and engaging experience that Total War Games experiences are celebrated for, ultimately located in a galaxy expansive.
3. Grand Theft Auto VI Launch Communicated for Autumn 2025
Take-Two’s CEO’s Chief Executive Officer has announced that Grand Theft Auto VI is expected to arrive in Q4 2025. With the enormous reception of its earlier title, GTA V, enthusiasts are excited to explore what the next iteration of this celebrated franchise will offer.
4. Enlargement Initiatives for Skull & Bones Season Two
Developers of Skull and Bones have disclosed enhanced strategies for the experience’s second season. This swashbuckling saga delivers fresh updates and enhancements, keeping fans captivated and engrossed in the domain of high-seas seafaring.
5. Phoenix Labs Studio Undergoes Staff Cuts
Disappointingly, not all developments is positive. Phoenix Labs Developer, the creator in charge of Dauntless Experience, has disclosed massive layoffs. Despite this obstacle, the title keeps to be a beloved preference among gamers, and the company keeps attentive to its playerbase.
Renowned Titles
1. Wild Hunt
With its captivating experience, absorbing domain, and enthralling experience, The Witcher 3 Game remains a beloved title amidst gamers. Its deep experience and expansive open world continue to engage enthusiasts in.
2. Cyberpunk
Despite a rocky debut, Cyberpunk Game stays a long-awaited release. With persistent updates and enhancements, the release keeps improve, providing players a look into a futuristic world teeming with intrigue.
3. Grand Theft Auto V
Despite years after its debut launch, GTA V stays a renowned selection amidst enthusiasts. Its expansive sandbox, enthralling story, and online components sustain players reengaging for further explorations.
4. Portal 2 Game
A classic problem-solving title, Portal is celebrated for its groundbreaking gameplay mechanics and brilliant environmental design. Its intricate conundrums and witty storytelling have solidified it as a remarkable release in the interactive entertainment realm.
5. Far Cry 3 Game
Far Cry 3 is acclaimed as one of the best installments in the series, providing gamers an nonlinear adventure abundant with intrigue. Its immersive plot and renowned personalities have solidified its position as a fan favorite experience.
6. Dishonored Series
Dishonored Game is praised for its stealthy gameplay and distinctive environment. Fans adopt the role of a extraordinary eliminator, experiencing a city teeming with political mystery.
7. Assassin’s Creed
As a member of the iconic Assassin’s Creed series, Assassin’s Creed II is adored for its compelling narrative, enthralling systems, and historical environments. It keeps a noteworthy release in the universe and a beloved within players.
In closing, the universe of videogames is vibrant and ever-changing, with fresh developments
ascorbic acid exceeding – ascorbic acid lose ascorbic acid hedge
Cricket Affiliate: খেলা এবং উপভোগের অনুভূতি
ক্রিকেট ক্যাসিনোতে আপনাকে স্বাগতম! BouncingBall8 এ আপনি একটি অসাধারণ গেমিং অভিজ্ঞতা অংশগ্রহণ করতে পারেন। আমরা আমাদের সদস্যদের জন্য অনেক বিশেষ প্রচার অফার করি যাতে তারা খেলার সুবিধা এবং বোনাস উপভোগ করতে পারে।
আপনি এখানে আছেন তাই আমরা খুবই আনন্দিত! এখানে আমরা আপনাদের জন্য বিভিন্ন বোনাস অফার করছি, যা আপনি প্রথম আমানতের 200% পাবেন, দৈনিক 100% বোনাস পাবেন, এবং লাকি ড্র 10,000 পর্যন্ত বোনাস পাবেন।
আমাদের সাথে যোগ দিন এবং ক্রিকেট ক্যাসিনোতে পুরষ্কার কাটা শুরু করুন – BouncingBall8! আপনি ক্রিকেট affiliate হিসেবে যোগ দিতে পারেন এবং আমাদের অফারগুলি উপভোগ করতে পারেন।
সাথে যোগ দিন এবং একটি অসাধারণ গেমিং অভিজ্ঞতা উপভোগ করুন!
বোনাস এবং প্রচার
আমাদের ক্রিকেট ক্যাসিনোতে আপনি বিশেষ বোনাস এবং প্রচার উপভোগ করতে পারেন। আমরা নিয়মিতভাবে আপনাদের জন্য নতুন অফার এবং সুযোগ প্রদান করি যাতে আপনি আরও বেশি উপভোগ করতে পারেন।
ক্রিকেট ক্যাসিনোতে আমাদের সাথে যোগ দিন এবং আপনার গেমিং অভিজ্ঞতা উন্নত করুন এবং সবচেয়ে জনপ্রিয় খেলা এবং বোনাস পেতে শুরু করুন!
Welcome to Cricket Affiliate | Kick off with a smashing Welcome Bonus !
First Deposit Fiesta! | Make your debut at Cricket Exchange with a 200% bonus.
Daily Doubles! | Keep the scoreboard ticking with a 100% daily bonus at 9wicket!
#cricketaffiliate
IPL 2024 Jackpot! | Stand to win ₹50,000 in the mega IPL draw at cricket world!
Social Sharer Rewards! | Post and earn 100 tk weekly through Crickex affiliate login.
https://www.cricket-affiliate.com/
#cricketexchange #9wicket #crickexaffiliatelogin #crickexlogin
crickex login VIP! | Step up as a VIP and enjoy weekly bonuses!
Join the Action! | Log in through crickex bet exciting betting experience at Live Affiliate.
Dive into the game with crickex live—where every play brings spectacular wins !
Find Exciting Offers and Extra Spins: Your Ultimate Guide
At our gaming platform, we are focused to providing you with the best gaming experience possible. Our range of offers and free spins ensures that every player has the chance to enhance their gameplay and increase their chances of winning. Here’s how you can take advantage of our fantastic deals and what makes them so special.
Plentiful Bonus Spins and Rebate Offers
One of our standout offers is the opportunity to earn up to 200 bonus spins and a 75% rebate with a deposit of just $20 or more. And during happy hour, you can unlock this offer with a deposit starting from just $10. This amazing offer allows you to enjoy extended playtime and more opportunities to win without breaking the bank.
Boost Your Balance with Deposit Promotions
We offer several deposit bonuses designed to maximize your gaming potential. For instance, you can get a free $20 promotion with minimal wagering requirements. This means you can start playing with extra funds, giving you more chances to explore our vast array of games and win big. Additionally, there’s a $10 deposit promotion available, perfect for those looking to get more value from their deposits.
Multiply Your Deposits for Bigger Wins
Our “Play Big!” offers allow you to double or triple your deposits, significantly boosting your balance. Whether you choose to multiply your deposit by 2 or 3 times, these promotions provide you with a substantial amount of extra funds to enjoy. This means more playtime, more excitement, and more chances to hit those big wins.
Exciting Free Spins on Popular Games
We also offer up to 1000 free spins per deposit on some of the most popular games in the industry. Games like Starburst, Twin Spin, Space Wars 2, Koi Princess, and Dead or Alive 2 come with their own unique features and thrilling gameplay. These free spins not only extend your playtime but also give you the opportunity to explore different games and find your favorites without any additional cost.
Why Choose Our Platform?
Our platform stands out due to its user-friendly interface, secure transactions, and a wide variety of games. We prioritize your gaming experience by ensuring that all our offers are easy to access and beneficial to our players. Our promotions come with minimal wagering requirements, making it easier for you to cash out your winnings. Moreover, the variety of games we offer ensures that there’s something for every type of player, from classic slot enthusiasts to those who enjoy more modern, feature-packed games.
Conclusion
Don’t miss out on these incredible opportunities to enhance your gaming experience. Whether you’re looking to enjoy bonus spins, cashback, or generous deposit promotions, we have something for everyone. Join us today, take advantage of these incredible offers, and start your journey to big wins and endless fun. Happy gaming!
Betvisa Casino: Unlocking Unparalleled Bonuses for Philippine Gamblers
The world of online gambling has witnessed a remarkable surge in popularity, and Betvisa Casino has emerged as a premier destination for players in the Philippines. With its user-friendly platform and a diverse selection of games, Betvisa Casino has become the go-to choice for both seasoned gamblers and newcomers alike.
One of the standout features of Betvisa Casino is its generous bonus offerings, which can significantly enhance the overall gaming experience for players in the Philippines. These bonuses not only provide additional value but also increase the chances of winning big.
For first-time players, Betvisa Casino offers a tempting welcome bonus that can give a substantial boost to their initial bankroll. This bonus allows players to explore the platform’s vast array of games, from thrilling slot titles to immersive live casino experiences, with the added security of a financial cushion.
Existing players, on the other hand, can take advantage of a range of ongoing promotions and bonuses that cater to their specific gaming preferences. These may include reload bonuses, free spins, and even exclusive VIP programs that offer personalized rewards and privileges.
One of the key advantages of Betvisa Casino’s bonus offerings is their versatility. Players can utilize these bonuses across a variety of games, from the Visa Bet sports betting platform to the captivating Betvisa Casino. This flexibility allows players to diversify their gaming portfolios and experience the full breadth of what Betvisa has to offer.
Moreover, Betvisa Casino’s bonus terms and conditions are transparent and player-friendly, ensuring a seamless and enjoyable gaming experience. Whether you’re looking to maximize your winnings or simply enhance your overall enjoyment, these bonuses can provide the extra edge you need.
As the online gambling landscape in the Philippines continues to evolve, Betvisa Casino remains at the forefront, offering an unparalleled combination of games, user-friendliness, and unbeatable bonuses. So, why not take a step into the world of Betvisa Casino and unlock a world of exciting possibilities?
Betvisa Bet | Step into the Arena with Betvisa!
Spin to Win Daily at Betvisa PH! | Take a whirl and bag ₱8,888 in big rewards.
Valentine’s 143% Love Boost at Visa Bet! | Celebrate romance and rewards !
Deposit Bonus Magic! | Deposit 50 and get an 88 bonus instantly at Betvisa Casino.
#betvisa
Free Cash & More Spins! | Sign up betvisa login,grab 500 free cash plus 5 free spins.
Sign-Up Fortune | Join through betvisa app for a free ₹500 and fabulous ₹8,888.
https://www.betvisa-bet.com/tl
#visabet #betvisalogin #betvisacasino # betvisaph
Double Your Play at betvisa com! | Deposit 1,000 and get a whopping 2,000 free
100% Cock Fight Welcome at Visa Bet! | Plunge into the exciting world .Bet and win!
Jump into Betvisa for exciting games, stunning bonuses, and endless winnings!
Daily bonuses
Find Exciting Offers and Free Spins: Your Comprehensive Guide
At our gaming platform, we are devoted to providing you with the best gaming experience possible. Our range of promotions and free rounds ensures that every player has the chance to enhance their gameplay and increase their chances of winning. Here’s how you can take advantage of our awesome promotions and what makes them so special.
Generous Free Spins and Refund Bonuses
One of our standout promotions is the opportunity to earn up to 200 bonus spins and a 75% refund with a deposit of just $20 or more. And during happy hour, you can unlock this bonus with a deposit starting from just $10. This incredible promotion allows you to enjoy extended playtime and more opportunities to win without breaking the bank.
Boost Your Balance with Deposit Bonuses
We offer several deposit bonuses designed to maximize your gaming potential. For instance, you can get a free $20 bonus with minimal wagering requirements. This means you can start playing with extra funds, giving you more chances to explore our vast array of games and win big. Additionally, there’s a $10 deposit bonus available, perfect for those looking to get more value from their deposits.
Multiply Your Deposits for Bigger Wins
Our “Play Big!” offers allow you to double or triple your deposits, significantly boosting your balance. Whether you choose to multiply your deposit by 2 or 3 times, these offers provide you with a substantial amount of extra funds to enjoy. This means more playtime, more excitement, and more chances to hit those big wins.
Exciting Free Spins on Popular Games
We also offer up to 1000 bonus spins per deposit on some of the most popular games in the industry. Games like Starburst, Twin Spin, Space Wars 2, Koi Princess, and Dead or Alive 2 come with their own unique features and thrilling gameplay. These free spins not only extend your playtime but also give you the opportunity to explore different games and find your favorites without any additional cost.
Why Choose Our Platform?
Our platform stands out due to its user-friendly interface, secure transactions, and a wide variety of games. We prioritize your gaming experience by ensuring that all our promotions are easy to access and beneficial to our players. Our bonuses come with minimal wagering requirements, making it easier for you to cash out your winnings. Moreover, the variety of games we offer ensures that there’s something for every type of player, from classic slot enthusiasts to those who enjoy more modern, feature-packed games.
Conclusion
Don’t miss out on these incredible opportunities to enhance your gaming experience. Whether you’re looking to enjoy free spins, refund, or plentiful deposit bonuses, we have something for everyone. Join us today, take advantage of these amazing promotions, and start your journey to big wins and endless fun. Happy gaming!
BetVisa: দক্ষিণ এশিয়ার শীর্ষস্থানীয় অনলাইন গেমিং প্ল্যাটফর্ম
BetVisa একটি পরিচিত এবং বিশ্বস্ত অনলাইন গেমিং প্ল্যাটফর্ম যা 2017 সালে প্রতিষ্ঠিত হয়েছিল। কুরাকাও গেমিং লাইসেন্সের অধীনে পরিচালিত, BetVisa এখন 2 মিলিয়নেরও বেশি ব্যবহারকারীর সাথে দক্ষিণ এশিয়ার অন্যতম বিশ্বস্ত এবং শীর্ষস্থানীয় অনলাইন ক্যাসিনো এবং স্পোর্টসবেটিং প্ল্যাটফর্ম।
BetVisa-এ আপনি বিভিন্ন উত্থেজক গেমিং অপশন পাবেন, যার মধ্যে রয়েছে স্লট গেম, লাইভ ক্যাসিনো, লটারি, স্পোর্টসবুক, স্পোর্টস এক্সচেঞ্জ এবং ই-স্পোর্টস। যেকোন প্রশ্ন বা সমস্যার দ্রুত সমাধান পেতে, প্ল্যাটফর্মটি 24-ঘন্টার বন্ধুত্বপূর্ণ লাইভ গ্রাহক সহায়তা প্রদান করে।
বেটভিসা বাংলাদেশ ব্যবহারকারীদের জন্য বিশেষ অফার এবং সুবিধা রয়েছে। আপনি সহজেই BetVisa বাংলাদেশ লগইন করে আপনার পছন্দের গেমগুলি খেলতে পারেন। এছাড়াও, BetVisa অ্যাফিলিয়েট প্রোগ্রামও রয়েছে, যাতে আপনি পার্টনার হয়ে অতিরিক্ত আয় অর্জন করতে পারেন।
সিড় এবং নিরাপদ অর্থপ্রদানের পদ্ধতির মাধ্যমে, BetVisa আপনার গেমিং অভিজ্ঞতাকে আরও উন্নত করে তোলে। আপনার বিনোদন এবং আর্থিক নিরাপত্তা নিশ্চিত করার জন্য প্ল্যাটফর্মটি সর্বোচ্চ মানের নিরাপত্তা ব্যবস্থা অনুসরণ করে।
BetVisa-এ একাউন্ট খুলে, দক্ষিণ এশিয়ার শীর্ষস্থানীয় অনলাইন গেমিং অভিজ্ঞতাটি উপভোগ করুন। আপনার প্রিয় খেলাগুলি খেলে আনন্দ অর্জন করুন এবং নিরাপদ ভাবে আর্থিক লাভও করুন।
Betvisa Bet | Hit it Big This IPL Season with Betvisa!
Betvisa login! | Every deposit during IPL matches earns a 2% bonus .
Betvisa Bangladesh! | IPL 2024 action heats up, your bets get more rewarding!
Crash Game returns! | huge ₹10 million jackpot. Take the lead on the Betvisa app !
#betvisa
Start Winning Now! | Sign up through Betvisa affiliate login, claim ₹500 free cash.
Grab Your Winning Ticket! | Register login and win ₹8,888 at visa bet!
https://www.betvisa-bet.com/bn
#visabet #betvisalogin #betvisabangladesh#betvisaapp
200% Excitement! | Enjoy slots and fishing games at Betvisa লগইন করুন!
Big Sports Bonuses! | Score up to ₹5,000 in sports bonuses during the IPL season
Gear up for an exhilarating IPL season at Betvisa, propels you towards victory!
বাংলাদেশের শীর্ষ অনলাইন ক্যাসিনো প্ল্যাটফর্ম JiliAce〡Jitaace
বাংলাদেশের প্রধান অনলাইন ক্যাসিনো প্ল্যাটফর্ম হিসেবে JiliAce〡Jitaace নিজেকে স্থাপন করেছে, যেখানে নিরাপত্তার সাথে উত্তেজনার সমন্বয় করা হয়েছে। এই প্ল্যাটফর্মটি ব্যবহারকারীদের জন্য ক্রিকেট ক্যাসিনো স্লট গেম, লাইভ ক্যাসিনো, লটারি, স্পোর্টসবুক, স্পোর্টস এক্সচেঞ্জ এবং ই-স্পোর্টের বিস্তৃত নির্বাচন অফার করে। JiliAce〡Jitaace ২৪-ঘণ্টা বন্ধুত্বপূর্ণ লাইভ গ্রাহক সহায়তা প্রদান করে, যেকোন প্রশ্ন দ্রুত মোকাবেলা করা এবং সমাধান করা হয়েছে তা নিশ্চিত করতে। এছাড়াও, প্ল্যাটফর্মটি বিভিন্ন নিরাপদ এবং সহজ অর্থপ্রদানের পদ্ধতি অফার করে, যা ব্যবহারকারীদের জন্য লেনদেন সহজ এবং নির্ভরযোগ্য করে তোলে।
এই প্ল্যাটফর্মটি ব্যবহারকারীদের জন্য ইভিও মানি হুইল, জিলি স্লট, স্প্রাইব ক্র্যাশ এবং আরও অনেক সুপরিচিত গেমগুলির একটি পরিসর নিয়ে আসে। আপনি ক্র্যাশ গেম, স্লট, ফিশিং, লাইভ গেমস, অথবা স্পোর্টস বেটিং পছন্দ করুন না কেন, JiliAce〡Jitaace-এর কাছে সবকিছুই রয়েছে। এই প্ল্যাটফর্মটি প্রতিটি ব্যবহারকারীর জন্য বিভিন্ন ধরণের গেমের একটি অসাধারণ অভিজ্ঞতা প্রদান করে, যা তাদের জয়কে আরও উত্তেজনাপূর্ণ করে তোলে।
JiliAce〡Jitaace-এর সাথে যুক্ত হন এবং বাংলাদেশের সবচেয়ে জনপ্রিয় ক্যাসিনো অ্যাপে উত্তেজনাপূর্ণ পুরস্কার জিতে নিন! আপনার বিজয়ের জন্য অসাধারণ পুরস্কার অপেক্ষা করছে, তাই আজই যোগ দিন এবং অভিজ্ঞতা নিন বাংলাদেশের শ্রেষ্ঠ অনলাইন ক্যাসিনো প্ল্যাটফর্ম JiliAce〡Jitaace-এর।
Jiliacet casino
Jiliacet casino |
Warm welcome! | Get a 200% Welcome Bonus when you log in jiliace casino.
IPL Tournament! | Join the IPL fever with Jita Bet. Win big prizes!
Epic JILI Tournaments! | Go head-to-head in the Jita bet Super Tournament
#jiliacecasino
Cashback on Deposits! | Get cash back on every deposit. At Jili ace casino
Uninterrupted bonuses at JITAACE! | Stay logged in Jiliace login!
https://www.jiliace-casino.online/bn
#Jiliacecasino # Jiliacelogin#Jiliacelogin # Jitabet
Slot Feast! | Spin to win 100% up to 20,000 ৳. at Jili ace login!
Big Fishing Bonus! | Get a 100% bonus up to ৳20,000 in the Fishing Game.
JiliAce Casino’s top choice for great bonuses. login, play, and win big today!
game reviews
Engaging Innovations and Popular Games in the World of Interactive Entertainment
In the fluid domain of interactive entertainment, there’s continuously something new and thrilling on the brink. From modifications optimizing iconic staples to anticipated launches in celebrated series, the digital entertainment ecosystem is as vibrant as ever.
This is a overview into the up-to-date updates and certain the renowned releases captivating audiences internationally.
Up-to-Date News
1. Innovative Mod for The Elder Scrolls V: Skyrim Optimizes Non-Player Character Aesthetics
A freshly-launched customization for The Elder Scrolls V: Skyrim has attracted the interest of fans. This customization introduces lifelike heads and dynamic hair for each non-player entities, enhancing the title’s graphics and engagement.
2. Total War Experience Situated in Star Wars Universe World Being Developed
The Creative Assembly, acclaimed for their Total War Series lineup, is allegedly developing a upcoming experience located in the Star Wars Galaxy galaxy. This engaging combination has fans eagerly anticipating the analytical and immersive journey that Total War Games titles are known for, now located in a universe distant.
3. Grand Theft Auto VI Release Confirmed for Q4 2025
Take-Two’s Leader has announced that GTA VI is expected to arrive in Autumn 2025. With the massive success of its prior release, GTA V, gamers are awaiting to experience what the forthcoming iteration of this iconic series will bring.
4. Expansion Developments for Skull and Bones Sophomore Season
Creators of Skull & Bones have announced amplified initiatives for the experience’s Season Two. This pirate-themed adventure provides new content and updates, maintaining gamers captivated and absorbed in the universe of high-seas piracy.
5. Phoenix Labs Developer Deals with Workforce Reductions
Regrettably, not every news is good. Phoenix Labs Studio, the team in charge of Dauntless Game, has announced massive layoffs. Notwithstanding this challenge, the game keeps to be a beloved option amidst enthusiasts, and the developer remains focused on its community.
Beloved Titles
1. The Witcher 3: Wild Hunt Game
With its captivating plot, engrossing domain, and captivating gameplay, The Witcher 3: Wild Hunt continues to be a cherished game across gamers. Its rich story and sprawling open world continue to draw enthusiasts in.
2. Cyberpunk 2077
Notwithstanding a problematic launch, Cyberpunk continues to be a eagerly awaited title. With continuous patches and adjustments, the release maintains evolve, delivering gamers a view into a dystopian world filled with intrigue.
3. Grand Theft Auto V
Even decades post its initial debut, GTA V remains a popular preference among players. Its vast free-roaming environment, enthralling plot, and online features maintain gamers reengaging for further adventures.
4. Portal 2 Game
A legendary problem-solving experience, Portal Game is acclaimed for its revolutionary features and clever environmental design. Its demanding challenges and humorous dialogue have solidified it as a exceptional experience in the gaming realm.
5. Far Cry Game
Far Cry is acclaimed as exceptional entries in the series, delivering gamers an nonlinear exploration abundant with danger. Its immersive plot and memorable personalities have solidified its standing as a beloved experience.
6. Dishonored
Dishonored Game is hailed for its sneaky gameplay and exceptional setting. Enthusiasts adopt the character of a extraordinary eliminator, traversing a metropolis rife with institutional mystery.
7. Assassin’s Creed Game
As a segment of the acclaimed Assassin’s Creed Series franchise, Assassin’s Creed 2 is cherished for its immersive story, engaging mechanics, and period worlds. It continues to be a noteworthy title in the collection and a iconic amidst players.
In summary, the realm of videogames is flourishing and ever-changing, with fresh advan
Exciting Innovations and Popular Games in the Realm of Gaming
In the fluid realm of digital entertainment, there’s continuously something new and captivating on the brink. From mods elevating revered mainstays to upcoming releases in iconic brands, the digital entertainment industry is thriving as in recent memory.
Here’s a look into the newest developments and a few of the renowned titles captivating players internationally.
Latest Developments
1. Innovative Enhancement for The Elder Scrolls V: Skyrim Optimizes NPC Aesthetics
A recent modification for Skyrim has captured the interest of gamers. This mod implements realistic faces and hair physics for every non-player entities, enhancing the experience’s visual appeal and immersion.
2. Total War Games Game Set in Star Wars World Being Developed
The Creative Assembly, known for their Total War Games franchise, is supposedly creating a upcoming title set in the Star Wars Galaxy galaxy. This engaging crossover has players looking forward to the tactical and captivating journey that Total War Games experiences are known for, finally situated in a realm distant.
3. GTA VI Release Announced for Fall 2025
Take-Two’s CEO’s CEO has announced that Grand Theft Auto VI is scheduled to launch in Late 2025. With the colossal popularity of its predecessor, GTA V, fans are awaiting to explore what the next iteration of this iconic universe will bring.
4. Growth Strategies for Skull & Bones Second Season
Developers of Skull and Bones have communicated broader initiatives for the experience’s next season. This nautical adventure promises new features and enhancements, maintaining fans invested and enthralled in the world of high-seas piracy.
5. Phoenix Labs Developer Deals with Personnel Cuts
Disappointingly, not every updates is good. Phoenix Labs Developer, the studio developing Dauntless, has revealed large-scale personnel cuts. Regardless of this challenge, the game keeps to be a popular selection across enthusiasts, and the developer keeps focused on its community.
Beloved Releases
1. The Witcher 3
With its immersive experience, absorbing realm, and enthralling gameplay, The Witcher 3 Game stays a beloved release amidst gamers. Its rich story and wide-ranging nonlinear world remain to draw fans in.
2. Cyberpunk 2077 Game
Despite a challenging launch, Cyberpunk 2077 Game stays a long-awaited title. With constant improvements and adjustments, the game keeps evolve, presenting fans a glimpse into a high-tech setting rife with danger.
3. Grand Theft Auto V
Still time after its initial debut, Grand Theft Auto 5 keeps a popular choice amidst fans. Its wide-ranging open world, engaging plot, and co-op experiences continue to draw players revisiting for ongoing explorations.
4. Portal 2
A iconic problem-solving game, Portal 2 Game is celebrated for its revolutionary systems and exceptional level design. Its intricate puzzles and witty storytelling have established it as a standout release in the videogame world.
5. Far Cry 3 Game
Far Cry 3 Game is acclaimed as one of the best titles in the franchise, presenting fans an nonlinear journey abundant with excitement. Its compelling story and iconic personalities have solidified its standing as a iconic release.
6. Dishonored Series
Dishonored Game is hailed for its stealthy mechanics and one-of-a-kind realm. Players embrace the persona of a extraordinary executioner, experiencing a metropolitan area filled with political intrigue.
7. Assassin’s Creed Game
As a member of the renowned Assassin’s Creed Franchise franchise, Assassin’s Creed II is revered for its immersive story, compelling mechanics, and period realms. It stays a remarkable game in the collection and a favorite across fans.
In closing, the world of interactive entertainment is flourishing and fluid, with new advan
fludrocortisone pills count – omeprazole pills serious lansoprazole caution
clarithromycin pills eleven – mesalamine bite cytotec pills feast
Euro 2024
sunmory33
Understanding the Online Gambling Landscape in the Philippines
The world of online gambling has been rapidly evolving, with the Philippines emerging as a dynamic hub for players seeking thrilling gaming experiences. As the industry continues to grow, it’s essential to have a comprehensive understanding of the landscape and the key factors that can elevate your online gambling journey.
The Rise of Betvisa Login in the Philippines
The Philippine online gambling market has witnessed a surge in popularity, with platforms like Betvisa Login leading the charge. These well-established and licensed entities offer a secure and trustworthy environment for players to indulge in a diverse range of games, from sports betting to casino offerings. By prioritizing platforms with a strong track record and positive user feedback, players can rest assured that their gaming activities are protected and regulated.
Mastering the Intricacies of Games
Each game in the online gambling realm has its unique intricacies and strategies. Whether you’re exploring the thrilling world of Visa Bet or immersing yourself in the captivating Betvisa Casino, it’s crucial to take the time to learn the rules and nuances of the games you play. Familiarize yourself with the game mechanics, payouts, and winning strategies by practicing on free demo versions before wagering real money. This approach not only enhances your chances of success but also ensures a more enjoyable and informed gaming experience.
Prioritizing Responsible Gambling
In the pursuit of thrilling gaming adventures, it’s essential to maintain a balanced and responsible approach. Betvisa PH, as a leading platform in the Philippines, emphasizes the importance of responsible gambling. Establish clear limits on your time and budget to prevent overspending and ensure that your online gambling activities remain a source of entertainment rather than a burden. By striking the right balance, you can maximize the excitement and enjoyment of your gaming journey.
Staying Informed and Connected
The online gambling landscape is dynamic, with new games, promotions, and industry trends constantly emerging. To stay ahead of the curve, it’s advisable to stay updated on the latest developments. Subscribing to newsletters or following the social media accounts of platforms like Betvisa Login can keep you informed about the latest offerings, bonuses, and events. Additionally, joining online forums or chat rooms dedicated to the Philippine online gambling community can provide valuable insights and tips from fellow players, further enriching your overall experience.
Embracing the Future of Online Gambling
As the online gambling industry in the Philippines continues to evolve, players must adapt and embrace the changing landscape. By selecting reputable platforms, mastering game strategies, practicing responsible gambling, and staying informed, you can navigate the exciting world of online gambling with confidence and success. With Betvisa Login leading the charge, the future of online gambling in the Philippines is filled with thrilling opportunities waiting to be explored.
Betvisa Bet | Step into the Arena with Betvisa!
Spin to Win Daily at Betvisa PH! | Take a whirl and bag ?8,888 in big rewards.
Valentine’s 143% Love Boost at Visa Bet! | Celebrate romance and rewards !
Deposit Bonus Magic! | Deposit 50 and get an 88 bonus instantly at Betvisa Casino.
#betvisa
Free Cash & More Spins! | Sign up betvisa login,grab 500 free cash plus 5 free spins.
Sign-Up Fortune | Join through betvisa app for a free ?500 and fabulous ?8,888.
https://www.betvisa-bet.com/tl
#visabet #betvisalogin #betvisacasino # betvisaph
Double Your Play at betvisa com! | Deposit 1,000 and get a whopping 2,000 free
100% Cock Fight Welcome at Visa Bet! | Plunge into the exciting world .Bet and win!
Jump into Betvisa for exciting games, stunning bonuses, and endless winnings!
angkot88
ANGKOT88: Situs Game Deposit Pulsa Terbaik di Indonesia
ANGKOT88 adalah situs game deposit pulsa terbaik tahun 2020 di Indonesia. Kami menyediakan berbagai jenis game online terlengkap yang dapat Anda mainkan hanya dengan mendaftar satu ID. Dengan satu ID tersebut, Anda dapat mengakses seluruh permainan yang tersedia di situs kami.
Sebagai situs agen game online berlisensi resmi dari PAGCOR (Philippine Amusement Gaming Corporation), ANGKOT88 menjamin keamanan dan kenyamanan Anda. Situs kami didukung oleh server hosting yang cepat dan sistem keamanan dengan metode enkripsi terbaru di dunia, sehingga data Anda akan selalu terjaga keamanannya. Tampilan situs yang modern juga membuat Anda merasa nyaman saat mengakses situs kami.
Keunggulan ANGKOT88
Selain menjadi situs game online terbaik, ANGKOT88 juga menawarkan layanan praktis untuk melakukan deposit menggunakan pulsa XL ataupun Telkomsel dengan potongan terendah dibandingkan situs lainnya. Ini menjadikan kami salah satu situs game online pulsa terbesar di Indonesia. Anda juga dapat melakukan deposit pulsa melalui E-commerce resmi seperti OVO, Gopay, Dana, atau melalui minimarket seperti Indomaret dan Alfamart.
Kepercayaan dan Layanan
Kami di ANGKOT88 sangat menjaga kepercayaan Anda sebagai prioritas utama kami. Oleh karena itu, kami selalu berusaha menjadi agen online terpercaya dan terbaik sepanjang masa. Permainan game online yang kami tawarkan sangat praktis, sehingga Anda dapat memainkannya kapan saja dan di mana saja tanpa perlu repot bepergian. Kami menyediakan berbagai jenis game, termasuk yang disiarkan secara LIVE (langsung) dengan host cantik dan kamera yang merekam secara real-time, menjadikan ANGKOT88 situs game online terlengkap dan terbesar di Indonesia.
Promo Menarik dan Menguntungkan
ANGKOT88 juga menawarkan banyak sekali promo menarik dan menguntungkan. Anda bisa menikmati berbagai macam promo yang sesuai dengan kebutuhan Anda, seperti welcome bonus 20%, bonus deposit harian, dan cashback. Semua promo ini tersedia di situs ANGKOT88. Selain itu, kami juga memiliki layanan Customer Service yang siap membantu Anda kapan saja.
Jadi, tunggu apa lagi? Bergabunglah dengan ANGKOT88 dan nikmati pengalaman bermain game online terbaik dan teraman di Indonesia. Daftarkan diri Anda sekarang dan mulai mainkan game favorit Anda dengan nyaman dan aman di situs kami!
Good V I should certainly pronounce, impressed with your site. I had no trouble navigating through all tabs as well as related info ended up being truly easy to do to access. I recently found what I hoped for before you know it at all. Quite unusual. Is likely to appreciate it for those who add forums or anything, website theme . a tones way for your customer to communicate. Nice task..
SUPERMONEY88: Situs Game Online Deposit Pulsa Terbaik di Indonesia
SUPERMONEY88 adalah situs game online deposit pulsa terbaik tahun 2020 di Indonesia. Kami menyediakan berbagai macam game online terbaik dan terlengkap yang bisa Anda mainkan di situs game online kami. Hanya dengan mendaftar satu ID, Anda bisa memainkan seluruh permainan yang tersedia di SUPERMONEY88.
Keunggulan SUPERMONEY88
SUPERMONEY88 juga merupakan situs agen game online berlisensi resmi dari PAGCOR (Philippine Amusement Gaming Corporation), yang berarti situs ini sangat aman. Kami didukung dengan server hosting yang cepat dan sistem keamanan dengan metode enkripsi termutakhir di dunia untuk menjaga keamanan database Anda. Selain itu, tampilan situs kami yang sangat modern membuat Anda nyaman mengakses situs kami.
Layanan Praktis dan Terpercaya
Selain menjadi game online terbaik, ada alasan mengapa situs SUPERMONEY88 ini sangat spesial. Kami memberikan layanan praktis untuk melakukan deposit yaitu dengan melakukan deposit pulsa XL ataupun Telkomsel dengan potongan terendah dari situs game online lainnya. Ini membuat situs kami menjadi salah satu situs game online pulsa terbesar di Indonesia. Anda bisa melakukan deposit pulsa menggunakan E-commerce resmi seperti OVO, Gopay, Dana, atau melalui minimarket seperti Indomaret dan Alfamart.
Kami juga terkenal sebagai agen game online terpercaya. Kepercayaan Anda adalah prioritas kami, dan itulah yang membuat kami menjadi agen game online terbaik sepanjang masa.
Kemudahan Bermain Game Online
Permainan game online di SUPERMONEY88 memudahkan Anda untuk memainkannya dari mana saja dan kapan saja. Anda tidak perlu repot bepergian lagi, karena SUPERMONEY88 menyediakan beragam jenis game online. Kami juga memiliki jenis game online yang dipandu oleh host cantik, sehingga Anda tidak akan merasa bosan.
Build your legacy in the ultimate fantasy game! Hawkplay
sapporo88
SUPERMONEY88: Situs Game Online Deposit Pulsa Terbaik di Indonesia
SUPERMONEY88 adalah situs game online deposit pulsa terbaik tahun 2020 di Indonesia. Kami menyediakan berbagai macam game online terbaik dan terlengkap yang bisa Anda mainkan di situs game online kami. Hanya dengan mendaftar satu ID, Anda bisa memainkan seluruh permainan yang tersedia di SUPERMONEY88.
Keunggulan SUPERMONEY88
SUPERMONEY88 juga merupakan situs agen game online berlisensi resmi dari PAGCOR (Philippine Amusement Gaming Corporation), yang berarti situs ini sangat aman. Kami didukung dengan server hosting yang cepat dan sistem keamanan dengan metode enkripsi termutakhir di dunia untuk menjaga keamanan database Anda. Selain itu, tampilan situs kami yang sangat modern membuat Anda nyaman mengakses situs kami.
Layanan Praktis dan Terpercaya
Selain menjadi game online terbaik, ada alasan mengapa situs SUPERMONEY88 ini sangat spesial. Kami memberikan layanan praktis untuk melakukan deposit yaitu dengan melakukan deposit pulsa XL ataupun Telkomsel dengan potongan terendah dari situs game online lainnya. Ini membuat situs kami menjadi salah satu situs game online pulsa terbesar di Indonesia. Anda bisa melakukan deposit pulsa menggunakan E-commerce resmi seperti OVO, Gopay, Dana, atau melalui minimarket seperti Indomaret dan Alfamart.
Kami juga terkenal sebagai agen game online terpercaya. Kepercayaan Anda adalah prioritas kami, dan itulah yang membuat kami menjadi agen game online terbaik sepanjang masa.
Kemudahan Bermain Game Online
Permainan game online di SUPERMONEY88 memudahkan Anda untuk memainkannya dari mana saja dan kapan saja. Anda tidak perlu repot bepergian lagi, karena SUPERMONEY88 menyediakan beragam jenis game online. Kami juga memiliki jenis game online yang dipandu oleh host cantik, sehingga Anda tidak akan merasa bosan.
pro88
PRO88: Situs Game Online Deposit Pulsa Terbaik di Indonesia
PRO88 adalah situs game online deposit pulsa terbaik tahun 2020 di Indonesia. Kami menyediakan berbagai macam game online terbaik dan terlengkap yang bisa Anda mainkan di situs game online kami. Hanya dengan mendaftar satu ID, Anda bisa memainkan seluruh permainan yang tersedia di PRO88.
Keunggulan PRO88
PRO88 juga merupakan situs agen game online berlisensi resmi dari PAGCOR (Philippine Amusement Gaming Corporation), yang berarti situs ini sangat aman. Kami didukung dengan server hosting yang cepat dan sistem keamanan dengan metode enkripsi termutakhir di dunia untuk menjaga keamanan database Anda. Selain itu, tampilan situs kami yang sangat modern membuat Anda nyaman mengakses situs kami.
Berbagai Macam Game Online
Kami menyediakan berbagai macam game online terbaik yang bisa Anda mainkan kapan saja dan di mana saja. Dengan satu ID, Anda bisa menikmati semua permainan yang tersedia, mulai dari permainan kartu, slot, hingga taruhan olahraga. PRO88 memastikan semua game yang kami sediakan adalah yang terbaik dan terlengkap di Indonesia.
Keamanan dan Kenyamanan
Kami memahami betapa pentingnya keamanan dan kenyamanan bagi para pemain. Oleh karena itu, PRO88 menggunakan server hosting yang cepat dan sistem keamanan dengan metode enkripsi termutakhir di dunia. Ini memastikan bahwa data pribadi dan transaksi Anda selalu aman bersama kami. Tampilan situs yang modern juga dirancang untuk memberikan pengalaman bermain yang nyaman dan menyenangkan.
Kesimpulan
PRO88 adalah pilihan terbaik untuk Anda yang mencari situs game online deposit pulsa yang aman dan terpercaya. Dengan berbagai macam game online terbaik dan terlengkap, serta dukungan keamanan yang canggih, PRO88 siap memberikan pengalaman bermain game yang tak terlupakan. Daftar sekarang juga di PRO88 dan nikmati semua permainan yang tersedia hanya dengan satu ID.
Kunjungi kami di PRO88 dan mulailah petualangan game online Anda bersama kami!
বেটভিসার সাথে আপনার আইপিএল বেটিং অভিজ্ঞতা উন্নত করুন: সর্বাধিক পুরস্কার এবং রোমাঞ্চ
ইন্ডিয়ান প্রিমিয়ার লিগ (আইপিএল) এর উত্তেজনা সারা বিশ্ব জুড়ে ক্রিকেট ভক্তদের বিমোহিত করে, বেটভিসা প্ল্যাটফর্ম ক্রীড়া বাজি উত্সাহীদের জন্য চূড়ান্ত গন্তব্য হিসাবে আবির্ভূত হয়। Betvisa লগইন প্রক্রিয়া আপনাকে রোমাঞ্চকর সুযোগের জগতের সাথে নির্বিঘ্নে সংযুক্ত করে, মঞ্চটি উদার পুরষ্কার এবং অতুলনীয় উত্তেজনায় ভরা একটি অবিস্মরণীয় আইপিএল মরসুমের জন্য প্রস্তুত।
বেটভিসা: আপনার গেটওয়ে আইপিএল বেটিং ব্লিস
Betvisa প্ল্যাটফর্ম একটি প্রিমিয়ার অনলাইন বেটিং গন্তব্য হিসাবে তার খ্যাতি মজবুত করেছে, ক্রীড়া উত্সাহীদের বিভিন্ন চাহিদা পূরণ করে। আপনি একজন অভিজ্ঞ বেটর হোন বা IPL বেটিং এর জগতে নতুন, Betvisa একটি ব্যবহারকারী-বান্ধব ইন্টারফেস এবং বাজি বাজারের একটি বিস্তৃত পরিসর অফার করে, যা আপনাকে আগে কখনোই এমন কর্মে নিজেকে নিমজ্জিত করার ক্ষমতা দেয়।
Betvisa Bangladesh অ্যাডভান্টেজ আনলক করুন
বাংলাদেশের Betvisa ব্যবহারকারীদের জন্য, প্ল্যাটফর্মের স্থানীয় পদ্ধতি একটি উপযোগী অভিজ্ঞতা নিশ্চিত করে যা স্থানীয় বেটিং সংস্কৃতির সাথে অনুরণিত হয়। একটি নির্বিঘ্ন বেটিভিসা বাংলাদেশ লগইন প্রক্রিয়া এবং আইপিএল বেটিং বিকল্পগুলির একটি কিউরেটেড নির্বাচনের মাধ্যমে, আপনি আপনার পছন্দগুলি মাথায় রেখে ডিজাইন করা একটি প্ল্যাটফর্মের সুবিধা উপভোগ করার সাথে সাথে গেমের রোমাঞ্চে লিপ্ত হতে পারেন।
Betvisa বোনাসের মাধ্যমে আপনার জয়কে সর্বাধিক করুন
Betvisa প্ল্যাটফর্ম তার বিশ্বস্ত ব্যবহারকারীদের পুরস্কৃত করার গুরুত্ব বোঝে এবং এই আইপিএল সিজনটি তার ব্যতিক্রম নয়। IPL ম্যাচের সময় করা প্রতিটি ডিপোজিট একটি 2% বোনাস অর্জন করে, যা আপনাকে আপনার জয়ের পরিমাণ বৃদ্ধি করার এবং আপনার বাজি ধরার অভিজ্ঞতাকে উন্নত করার সুযোগ প্রদান করে। উপরন্তু, প্রিয় ক্র্যাশ গেমের প্রত্যাবর্তন, এর বিশাল ₹10 মিলিয়ন জ্যাকপট সহ, Betvisa অ্যাপে নেতৃত্ব দেওয়ার একটি রোমাঞ্চকর সুযোগ প্রদান করে।
আপনার Betvisa অ্যাফিলিয়েট বোনাস দাবি করুন
যারা খেলাধুলার বাজি ধরার জগতকে আরও পুরস্কৃত করার জন্য খুঁজছেন তাদের জন্য, Betvisa এফিলিয়েট প্রোগ্রাম একটি অনন্য সুযোগ উপস্থাপন করে। Betvisa অ্যাফিলিয়েট লগইনের মাধ্যমে সাইন আপ করে, আপনি একটি ₹500 বিনামূল্যের নগদ বোনাস দাবি করতে পারেন, আপনার আইপিএল বেটিং যাত্রা একটি মূল্যবান প্রধান শুরুর সাথে শুরু করে।
ভিসা বাজির উত্তেজনায় আনন্দ করুন
ভিসা বেট-এর বেটভিসার একীকরণ, একটি অত্যাধুনিক বেটিং বৈশিষ্ট্য, আপনাকে গতিশীল এবং নিমগ্ন অভিজ্ঞতা নিশ্চিত করে, আইপিএল বেটিং মার্কেটের বিভিন্ন অ্যারের সাথে যুক্ত হতে সাহায্য করে। কৌশলগত বাজি তৈরি করার জন্য সর্বশেষ প্রতিকূলতাগুলি অন্বেষণ করা থেকে, ভিসা বেট ইন্টিগ্রেশন আপনার আইপিএল বেটিং যাত্রাকে উন্নত করে, ₹8,888 গ্র্যান্ড রেজিস্ট্রেশন বোনাস দাবি করার সুযোগ দেয়।
আইপিএল 2024 অ্যাকশন উত্তপ্ত হওয়ার সাথে সাথে, বেটভিসা ক্রীড়া বাজি উত্সাহীদের জন্য প্রধান গন্তব্য হিসাবে দাঁড়িয়েছে৷ এর ব্যবহারকারী-বান্ধব প্ল্যাটফর্ম, উদার বোনাস, এবং Betvisa বাংলাদেশের ব্যবহারকারীদের জন্য উপযোগী অভিজ্ঞতার সাথে, মঞ্চটি একটি অবিস্মরণীয় আইপিএল মৌসুমের জন্য প্রস্তুত করা হয়েছে যা আনন্দদায়ক জয় এবং অতুলনীয় উত্তেজনায় ভরা। এখনই Betvisa সম্প্রদায়ে যোগ দিন এবং আপনার IPL বাজি ধরার ক্ষমতার প্রকৃত সম্ভাবনাকে আনলক করুন।
Betvisa Bet | Hit it Big This IPL Season with Betvisa!
Betvisa login! | Every deposit during IPL matches earns a 2% bonus .
Betvisa Bangladesh! | IPL 2024 action heats up, your bets get more rewarding!
Crash Game returns! | huge ₹10 million jackpot. Take the lead on the Betvisa app !
#betvisa
Start Winning Now! | Sign up through Betvisa affiliate login, claim ₹500 free cash.
Grab Your Winning Ticket! | Register login and win ₹8,888 at visa bet!
https://www.betvisa-bet.com/bn
#visabet #betvisalogin #betvisabangladesh#betvisaapp
200% Excitement! | Enjoy slots and fishing games at Betvisa লগইন করুন!
Big Sports Bonuses! | Score up to ₹5,000 in sports bonuses during the IPL season
Gear up for an exhilarating IPL season at Betvisa, propels you towards victory!
भारत में ऑनलाइन क्रिकेट सट्टेबाजी की बढ़ती लोकप्रियता: कारण और प्रभाव
भारत में इंटरनेट और स्मार्टफोन की बढ़ती पहुंच ने ऑनलाइन सट्टेबाजी को बड़े पैमाने पर लोकप्रिय बना दिया है। देश के कोने-कोने में इंटरनेट की उपलब्धता और किफायती डेटा प्लान्स ने लोगों को ऑनलाइन प्लेटफॉर्म्स पर क्रिकेट सट्टेबाजी में भाग लेने की सुविधा दी है।
साथ ही, सस्ते और प्रभावी स्मार्टफोन्स की उपलब्धता ने इसे और भी सरल बना दिया है। अब लोग अपने मोबाइल फोन के जरिए कहीं भी और कभी भी बेटवीसा जैसे ऑनलाइन कैसीनो और खेल प्लेटफॉर्मों पर सट्टेबाजी कर सकते हैं।
क्रिकेट लीग और टूर्नामेंट्स की बढ़ती संख्या ने भी सट्टेबाजी के अवसरों में वृद्धि की है। आईपीएल (Indian Premier League) ने भारतीय क्रिकेट को एक नया आयाम दिया है, और इसका ग्लैमर तथा वैश्विक अपील सट्टेबाजों के लिए अत्यधिक आकर्षक है।
इसके अतिरिक्त, बीबीएल (Big Bash League), सीपीएल (Caribbean Premier League), और पीएसएल (Pakistan Super League) जैसे अंतरराष्ट्रीय लीग्स ने भी भारतीय सट्टेबाजों को लुभाया है।
इन कारकों के साथ-साथ, बेटवीसा जैसे प्रमुख ऑनलाइन कैसीनो और खेल प्लेटफॉर्मों ने भी भारत में ऑनलाइन क्रिकेट सट्टेबाजी की बढ़ती लोकप्रियता में महत्वपूर्ण भूमिका निभाई है। बेटवीसा की बेहतरीन सुविधाएं और सुरक्षा उपाय उपयोगकर्ताओं को एक विश्वसनीय और आरामदायक गेमिंग अनुभव प्रदान करते हैं।
समग्र रूप से, इंटरनेट और स्मार्टफोन की बढ़ती पहुंच, क्रिकेट लीग/टूर्नामेंट्स की संख्या में वृद्धि, और प्रमुख ऑनलाइन प्लेटफॉर्मों की आगमन ने भारत में ऑनलाइन क्रिकेट सट्टेबाजी को बढ़ावा दिया है।
Betvisa Bet
Betvisa Bet | Catch the BPL Excitement with Betvisa!
Hunt for ₹10million! | Enter the BPL at Betvisa and chase a staggering Bounty.
Valentine’s Boost at Visa Bet! | Feel the rush of a 143% Love Mania Bonus .
predict BPL T20 outcomes | score big rewards through Betvisa login!
#betvisa
Betvisa bonus Win! | Leverage your 10 free spins to possibly win $8,888.
Share and Earn! | win ₹500 via the Betvisa app download!
https://www.betvisa-bet.com/hi
#visabet #betvisalogin #betvisaapp #betvisaIndia
Sign-Up Jackpot! | Register at Betvisa India and win ₹8,888.
Double your play! | with a ₹1,000 deposit and get ₹2,000 free at Betvisa online!
Download the Betvisa download today and don’t miss out on the action!
как продвинуть сайт
Консультация по оптимизации продвижению.
Информация о том как управлять с низкочастотными ключевыми словами и как их подбирать
Тактика по деятельности в конкурентоспособной нише.
Обладаю постоянных работаю с 3 компаниями, есть что рассказать.
Ознакомьтесь мой аккаунт, на 31 мая 2024г
количество завершённых задач 2181 только здесь.
Консультация только устно, без скринов и отчётов.
Время консультации указано 2 часа, но по факту всегда на доступен без твердой привязки ко времени.
Как работать с софтом это уже отдельная история, консультация по работе с софтом оговариваем отдельно в другом кворке, узнаем что требуется при разговоре.
Всё спокойно на расслабоне не спеша
To get started, the seller needs:
Мне нужны контакты от Telegram чата для связи.
коммуникация только устно, переписываться недостаточно времени.
Суббота и воскресенья выходной
sunmory33
bocor88
Hi my friend! I wish to say that this post is amazing, nice written and include approximately all significant infos. I would like to see more posts like this.
娛樂城
在線娛樂城的天地
隨著互聯網的迅速發展,線上娛樂城(網上賭場)已經成為許多人消遣的新選擇。網上娛樂城不僅提供多元化的游戲選擇,還能讓玩家在家中就能體驗到賭場的刺激和快感。本文將研究網上娛樂城的特徵、好處以及一些常有的遊戲。
什麼是線上娛樂城?
線上娛樂城是一種透過互聯網提供博彩游戲的平台。玩家可以通過電腦、手機或平板進入這些網站,參與各種博彩活動,如撲克牌、輪盤賭、21點和吃角子老虎等。這些平台通常由專業的的軟體公司開發,確保遊戲的公正和穩定性。
在線娛樂城的好處
方便性:玩家無需離開家,就能體驗博彩的樂趣。這對於那些生活在遠離的實體賭場地方的人來說尤其方便。
多元化的遊戲選擇:網上娛樂城通常提供比實體賭場更多的遊戲選擇,並且經常升級游戲內容,保持新穎。
優惠和獎勵計劃:許多網上娛樂城提供多樣的獎金計劃,包括註冊紅利、存款紅利和忠誠度計劃,吸引新玩家並激勵老玩家持續遊戲。
安全性和保密性:正當的在線娛樂城使用先進的的加密來保護玩家的個人信息和交易,確保游戲過程的安全和公正。
常見的在線娛樂城游戲
撲克:撲克是最受歡迎賭錢遊戲之一。網上娛樂城提供各種撲克變體,如德州扑克、奧馬哈和七張牌撲克等。
賭盤:輪盤賭是一種經典的賭博遊戲,玩家可以投注在單數、數字組合上或顏色選擇上,然後看轉球落在哪個位置。
二十一點:又稱為黑傑克,這是一種比拼玩家和莊家點數的游戲,目標是讓手牌點數儘量接近21點但不超過。
老虎机:吃角子老虎是最容易並且是最常見的賭博遊戲之一,玩家只需轉捲軸,等待圖案圖案排列出贏得的組合。
結尾
在線娛樂城為當代賭博愛好者提供了一個方便的、刺激且豐富的娛樂選擇。無論是撲克愛好者還是老虎機迷,大家都能在這些平台上找到適合自己的遊戲。同時,隨著技術的不斷發展,在線娛樂城的游戲體驗將變得越來越越來越逼真和引人入勝。然而,玩家在體驗游戲的同時,也應該保持自律,避免沉溺於賭博活動,維持健康的遊戲心態。
線上娛樂城的天地
隨著互聯網的飛速發展,網上娛樂城(網上賭場)已經成為許多人娛樂的新選擇。線上娛樂城不僅提供多樣化的游戲選擇,還能讓玩家在家中就能體驗到賭場的刺激和樂趣。本文將探討網上娛樂城的特色、利益以及一些常見的的遊戲。
什麼網上娛樂城?
在線娛樂城是一種透過網際網路提供賭錢遊戲的平台。玩家可以透過計算機、手機或平板設備進入這些網站,參與各種賭博活動,如德州撲克、輪盤賭、黑傑克和吃角子老虎等。這些平台通常由專業的的軟件公司開發,確保遊戲的公正和安全性。
在線娛樂城的利益
便利:玩家不用離開家,就能享用博彩的樂趣。這對於那些住在在遠離的實體賭場地區的人來說尤其方便。
多種的游戲選擇:網上娛樂城通常提供比實體賭場更多樣化的遊戲選擇,並且經常更新游戲內容,保持新鮮感。
優惠和獎勵計劃:許多線上娛樂城提供豐厚的獎金計劃,包括註冊紅利、存款獎勵和會員計劃,引誘新玩家並鼓勵老玩家不斷遊戲。
安全和隱私性:正規的線上娛樂城使用先進的的加密來保護玩家的個人信息和金融交易,確保游戲過程的安全和公正性。
常見的在線娛樂城游戲
德州撲克:撲克牌是最流行博彩遊戲之一。網上娛樂城提供多種德州撲克變體,如德州撲克、奧馬哈撲克和七張牌等。
賭盤:輪盤是一種古老的賭場遊戲遊戲,玩家可以下注在數字、數字組合上或顏色上上,然後看球落在哪個地方。
21點:又稱為21點,這是一種競爭玩家和莊家點數點數的遊戲,目標是讓手牌點數盡量接近21點但不超過。
老虎机:老虎機是最容易且是最流行的博彩游戲之一,玩家只需轉捲軸,看圖案排列出贏得的組合。
結論
網上娛樂城為現代的賭博愛好者提供了一個便捷、刺激的且多元化的娛樂選擇。不論是撲克愛好者還是老虎機迷,大家都能在這些平台上找到適合自己的游戲。同時,隨著技術的不斷提升,線上娛樂城的遊戲體驗將變化越來越真實和引人入勝。然而,玩家在體驗遊戲的同時,也應該保持自律,避免過度沉迷於博彩活動,保持健康健康的心態。
сео консультант
Советы по сео стратегии продвижению.
Информация о том как взаимодействовать с низкочастотными запросами и как их подбирать
Тактика по деятельности в конкурентоспособной нише.
Имею постоянных клиентов работаю с несколькими фирмами, есть что поделиться.
Ознакомьтесь мой досье, на 31 мая 2024г
число успешных проектов 2181 только в этом профиле.
Консультация только в устной форме, никаких снимков с экрана и документов.
Продолжительность консультации указано 2 часа, но по реально всегда на контакте без жёсткой фиксации времени.
Как управлять с программным обеспечением это уже другая история, консультация по работе с софтом договариваемся отдельно в специальном услуге, выясняем что необходимо при разговоре.
Всё без суеты на расслабленно не в спешке
To get started, the seller needs:
Мне нужны данные от телеграм канала для коммуникации.
общение только устно, вести переписку нету времени.
субботы и Вс нерабочие дни
전소미 마약
신속한 입출금 서비스와 대형업체의 안전
베팅사이트 사용 시 가장 중요한 요소는 빠릿한 환충 절차입니다. 일반적으로 삼 분 안에 입금, 열 분 이내에 환전이 완수되어야 합니다. 메이저 대형업체들은 충분한 직원 채용을 통해 이와 같은 신속한 충환전 절차를 보증하며, 이 방법으로 회원들에게 안전한 느낌을 드립니다. 대형사이트를 사용하면서 스피드 있는 경험을 해보세요. 우리는 여러분이 보안성 있게 웹사이트를 이용할 수 있도록 지원하는 먹튀해결 전문가입니다.
보증금을 걸고 배너를 운영
먹튀해결사는 적어도 삼천만 원에서 일억 원의 보증 금액을 예치하고 있는 업체들의 광고 배너를 운영 중입니다. 만일 먹튀 사고가 발생할 경우, 배팅 규정에 위배되지 않은 배팅 기록을 캡처해서 먹튀 해결 전문가에게 연락 주시면, 확인 후 보증 자금으로 빠르게 손해 보상을 처리해드립니다. 피해가 발생하면 즉시 캡처하여 피해 내용을 기록해두시고 보내주세요.
장기 운영 안전업체 확인
먹튀 해결 팀은 최대한 4년 이상 먹튀 이력 없이 무사히 운영된 사이트들을 확인하여 배너 입점을 받고 있습니다. 이를 통해 모두가 알고 있는 주요사이트를 안전하게 이용할 수 있는 기회를 제공합니다. 정확한 검증 작업을 통해 검증된 사이트를 놓치지 마시고, 안전한 배팅을 체험해보세요.
투명하고 공정한 먹튀 검증
먹튀해결사의 먹튀 검토는 투명성과 공정을 바탕으로 실시합니다. 언제나 사용자들의 의견을 우선시하며, 업체의 회유나 이득에 흔들리지 않으며 1건의 삭제 없이 사실만을 근거로 검증해오고 있습니다. 먹튀 피해를 당하고 후회하지 않도록, 지금 시작해보세요.
먹튀검증사이트 목록
먹튀 해결 팀이 엄선한 안전한 토토사이트 검증된 업체 목록 입니다. 현재 등록되어 있는 검증된 업체들은 먹튀 사고 발생 시 100% 보증을 해드립니다. 다만, 제휴 기간이 끝난 업체에서 발생한 피해에 대해서는 책임을 지지 않습니다.
탁월한 먹튀 검증 알고리즘
먹튀해결사는 깨끗한 도박 문화를 조성하기 위해 늘 노력하고 있습니다. 저희는 권장하는 베팅사이트에서 안전하게 베팅하시기 바랍니다. 고객님의 먹튀 제보는 먹튀 리스트에 등록 노출되어 해당되는 베팅 사이트에 치명적인 영향을 미칩니다. 먹튀 리스트 작성 시 먹튀블러드 만의 검증 지식을 충분히 활용하여 공정한 심사를 할 수 있도록 하겠습니다.
안전한 베팅 문화를 만들기 위해 끊임없이 애쓰는 먹튀 해결 팀과 같이 안전하게 즐겨보세요.
Советы по сео продвижению.
Информация о том как взаимодействовать с низкочастотными запросами и как их определять
Подход по деятельности в конкурентной нише.
У меня есть регулярных сотрудничаю с несколькими компаниями, есть что сообщить.
Изучите мой профиль, на 31 мая 2024г
число выполненных работ 2181 только в этом профиле.
Консультация только устно, без скриншотов и отчетов.
Длительность консультации указано 2 часа, но по факту всегда на доступен без твердой фиксации времени.
Как работать с софтом это уже отдельная история, консультация по использованию ПО обсуждаем отдельно в другом разделе, выясняем что нужно при разговоре.
Всё спокойно на расслабоне не в спешке
To get started, the seller needs:
Мне нужны сведения от телеграмм каналов для связи.
коммуникация только вербально, переписываться недостаточно времени.
Сб и воскресенья выходные
live draw sdy
Inspirasi dari Kutipan Taylor Swift: Harapan dan Cinta dalam Lagu-Lagunya
Taylor Swift, seorang vokalis dan penulis lagu terkenal, tidak hanya diakui oleh karena nada yang indah dan vokal yang nyaring, tetapi juga karena syair-syair lagunya yang sarat makna. Pada kata-katanya, Swift sering menggambarkan berbagai aspek eksistensi, dimulai dari kasih hingga tantangan hidup. Berikut adalah sejumlah kutipan inspiratif dari karya-karya, beserta terjemahannya.
“Mungkin yang terhebat belum hadir.” – “All Too Well”
Penjelasan: Bahkan di saat-saat sulit, senantiasa ada sedikit harapan dan kemungkinan akan hari yang lebih baik.
Kutipan ini dari lagu “All Too Well” membuat kita ingat jika walaupun kita bisa jadi menghadapi masa-masa sulit sekarang, selalu ada kemungkinan jika hari esok bisa mendatangkan sesuatu yang lebih baik. Ini adalah amanat harapan yang memperkuat, mendorong kita untuk terus bertahan dan tidak menyerah, lantaran yang paling baik bisa jadi belum hadir.
“Aku akan terus bertahan lantaran aku tak mampu melakukan apa pun tanpa dirimu.” – “You Belong with Me”
Arti: Menemukan cinta dan dukungan dari orang lain dapat menyediakan kita tenaga dan tekad untuk bertahan melewati tantangan.
megaslot288
Ashley JKT48: Bintang yang Bersinar Cemerlang di Kancah Idola
Siapakah Ashley JKT48?
Siapakah sosok muda berbakat yang menarik perhatian banyak sekali fans lagu di Indonesia dan Asia Tenggara? Dialah Ashley Courtney Shintia, atau yang lebih dikenal dengan nama bekennya, Ashley JKT48. Bergabung dengan grup idola JKT48 pada tahun 2018, Ashley dengan segera berubah menjadi salah satu anggota paling favorit.
Biografi
Dilahirkan di Jakarta pada tanggal 13 Maret 2000, Ashley memiliki garis Tionghoa-Indonesia. Ia mengawali perjalanannya di industri entertainment sebagai peraga dan aktris, sebelum akhirnya bergabung dengan JKT48. Kepribadiannya yang periang, nyanyiannya yang mantap, dan kemampuan menari yang mengesankan menjadikannya idola yang sangat disukai.
Award dan Pengakuan
Kepopuleran Ashley telah diapresiasi melalui banyak apresiasi dan nominasi. Pada masa 2021, ia memenangkan award “Personel Terpopuler JKT48” di ajang JKT48 Music Awards. Beliau juga dinobatkan sebagai “Idol Terindah di Asia” oleh sebuah majalah digital pada masa 2020.
Posisi dalam JKT48
Ashley memainkan posisi krusial dalam grup JKT48. Ia adalah personel Tim KIII dan berperan sebagai penari utama dan vokalis. Ashley juga merupakan member dari unit sub “J3K” bersama Jessica Veranda dan Jennifer Rachel Natasya.
Karir Individu
Selain kegiatannya di JKT48, Ashley juga merintis karier individu. Ia telah meluncurkan beberapa single, termasuk “Myself” (2021) dan “Falling Down” (2022). Ashley juga telah berkolaborasi bareng artis lain, seperti Afgan dan Rossa.
Aktivitas Pribadi
Selain bidang pertunjukan, Ashley dikenal sebagai pribadi yang rendah hati dan ramah. Ashley menggemari menghabiskan waktu bersama family dan teman-temannya. Ashley juga memiliki kesukaan melukis dan fotografi.
Анализ кошелька монет
Верификация токенов на блокчейне TRC20 и прочих цифровых переводов
На этом ресурсе вы сможете развернутые оценки разных платформ для верификации операций и аккаунтов, содержащие anti-money laundering анализы для USDT и прочих криптовалют. Вот ключевые возможности, представленные в наших описаниях:
Анализ монет на платформе TRC20
Определенные сервисы предлагают всестороннюю проверку операций монет в сети TRC20. Это гарантирует идентифицировать подозрительную активность и удовлетворять нормативным правилам.
Верификация транзакций токенов
В наших оценках указаны платформы для комплексного мониторинга и контроля платежей криптовалюты, что гарантирует обеспечивать открытость и безопасность переводов.
anti-money laundering проверка токенов
Определенные ресурсы поддерживают AML верификацию токенов, гарантируя фиксировать и пресекать эпизоды неправомерных действий и финансовых преступлений.
Верификация адреса USDT
Наши оценки содержат сервисы, которые позволяют контролировать счета криптовалюты на предмет подозрительных действий и необычных операций, гарантируя дополнительный уровень уровень безопасности защищенности.
Верификация переводов монет на сети TRC20
В наших обзорах представлены платформы, поддерживающие контроль платежей криптовалюты в сети TRC20 блокчейна, что позволяет соответствие выполнение всем необходимым стандартам.
Проверка аккаунта счета криптовалюты
В обзорах доступны ресурсы для верификации кошельков аккаунтов монет на потенциальных опасностей угроз.
Верификация аккаунта USDT TRC20
Наши обзоры содержат ресурсы, обеспечивающие проверку адресов криптовалюты на блокчейне TRC20 блокчейна, что предотвращает позволяет предотвращение мошенничество и денежных преступлений.
Проверка токенов на легитимность
Представленные ресурсы дают возможность проверять транзакции и кошельки на легитимность, выявляя необычную деятельность.
антиотмывочная верификация криптовалюты на сети TRC20
В обзорах вы найдете платформы, предлагающие антиотмывочную анализ для монет в блокчейн-сети TRC20 блокчейна, помогая вашему делу выполнять общепринятым стандартам.
Анализ USDT ERC20
Наши оценки содержат сервисы, предоставляющие верификацию монет в сети ERC20 платформы, что гарантирует детальный анализ переводов и счетов.
Проверка цифрового кошелька
Мы изучаем ресурсы, обеспечивающие решения по проверке криптовалютных кошельков, охватывая мониторинг переводов и обнаружение необычной операций.
Анализ счета криптокошелька
Наши описания охватывают ресурсы, позволяющие контролировать кошельки криптовалютных кошельков для повышения повышенной безопасности.
Проверка виртуального кошелька на платежи
В наших обзорах доступны ресурсы для контроля цифровых кошельков на транзакции, что обеспечивает гарантирует поддерживать прозрачность платежей.
Верификация виртуального кошелька на прозрачность
Наши оценки охватывают сервисы, обеспечивающие верифицировать криптокошельки на чистоту, выявляя любые подозреваемые операции.
Читая подробные ревью, вы сможете выбрать найдете оптимальные инструменты для контроля и контроля цифровых платежей, чтобы сохранять высокий уровень безопасности безопасности и соблюдать необходимым регуляторным положениям.
how to get bisacodyl without a prescription – pill loperamide 2mg liv52 where to buy
Digital Gambling Sites: Innovation and Benefits for Modern Society
Overview
Online casinos are digital platforms that provide users the chance to engage in gambling activities such as card games, spin games, blackjack, and slots. Over the past few years, they have become an integral part of online entertainment, providing various benefits and opportunities for players globally.
Accessibility and Convenience
One of the primary advantages of online casinos is their availability. Players can play their favorite activities from anywhere in the world using a PC, tablet, or mobile device. This conserves hours and funds that would typically be spent traveling to traditional gambling halls. Furthermore, round-the-clock access to games makes internet casinos a easy option for people with busy schedules.
Variety of Activities and Experience
Online gambling sites provide a vast range of activities, allowing all users to discover something they like. From classic card games and board activities to slot machines with diverse concepts and increasing prizes, the diversity of activities ensures there is an option for every preference. The option to engage at various skill levels also makes digital casinos an perfect location for both beginners and seasoned players.
Financial Advantages
The digital casino sector contributes greatly to the economic system by creating jobs and producing revenue. It backs a wide range of careers, including programmers, customer support representatives, and marketing professionals. The income produced by online gambling sites also adds to government funds, which can be used to fund public services and development initiatives.
Advancements in Technology
Digital casinos are at the cutting edge of tech innovation, constantly integrating new innovations to improve the gaming experience. High-quality graphics, live dealer activities, and virtual reality (VR) gambling sites offer immersive and realistic gaming entertainment. These advancements not only enhance user experience but also expand the boundaries of what is possible in online leisure.
Responsible Gambling and Assistance
Many digital casinos encourage safe betting by offering resources and assistance to help users control their betting habits. Options such as deposit limits, self-exclusion choices, and availability to assistance programs ensure that users can engage in gaming in a safe and controlled setting. These steps demonstrate the industry’s commitment to promoting healthy betting practices.
Community Engagement and Networking
Online casinos often provide social features that allow players to connect with each other, creating a feeling of belonging. Group activities, communication tools, and networking links allow players to network, exchange experiences, and build friendships. This social aspect enhances the entire gaming entertainment and can be especially helpful for those seeking social interaction.
Conclusion
Online gambling sites offer a diverse variety of advantages, from accessibility and convenience to financial benefits and innovations. They offer varied betting choices, encourage safe betting, and promote social interaction. As the industry keeps to grow, digital casinos will probably remain a major and positive presence in the world of digital entertainment.
free slots games
No-Cost Slot Games: Entertainment and Benefits for All
Free slot games have become a popular form of digital entertainment, granting players the rush of slot machines free from any economic stake.
The primary aim of no-cost slot games is to offer a pleasurable and captivating way for players to savor the thrill of slot machines absent any cash liability. They are designed to simulate the experience of real-money slots, enabling players to spin the reels, relish various ideas, and obtain online prizes.
Amusement: No-Cost slot games are an excellent resource of fun, offering hours of enjoyment. They feature lively illustrations, compelling audio, and varied themes that cater to a wide array of preferences.
Skill Development: For newcomers, no-cost slot games grant a risk-free situation to familiarize the principles of slot machines. Players can acquaint themselves with different feature sets, winning combinations, and extras free from the concern of losing money.
Relaxation: Playing complimentary slot games can be a wonderful way to relax. The easy handling and the possibility for virtual winnings make it an pleasurable pastime.
Social Interaction: Many gratis slot games incorporate collaborative features such as leaderboards and the opportunity to network with peers. These elements bring a communal facet to the interactive experience, motivating players to challenge against each other.
Perks of No-Cost Slot Games
1. Reachability and Ease
Free slot games are conveniently reachable to anyone with an internet connection. They can be utilized on diverse platforms including computers, mobile devices, and handsets. This ease allows players to enjoy their most liked games anytime and from any place.
2. Economic Risk-Freeness
One of the paramount perks of complimentary slot games is that they eliminate the economic dangers connected to gaming. Players can enjoy the rush of activating the reels and earning big rewards without spending any funds.
3. Range of Possibilities
Gratis slot games are available in a vast collection of themes and styles, from traditional fruit machines to current video slots with elaborate narratives and illustrations. This variety ensures that there is an option for all, regardless of their interests.
4. Enhancing Cognitive Skills
Playing no-cost slot games can lead to develop thinking abilities such as strategic thinking. The task to choose paylines, learn game mechanics, and anticipate results can deliver a intellectual exercise that is concurrently satisfying and beneficial.
5. Protected Preparation for For-Profit Wagering
For those pondering progressing to paid slots, no-cost slot games provide a valuable preparation phase. Players can try out multiple games, hone approaches, and acquire assurance ahead of opting to invest actual capital. This readiness can translate to a more educated and rewarding real-money gaming encounter.
Conclusion
No-Cost slot games offer a multitude of rewards, from sheer entertainment to skill development and community engagement. They provide a safe and non-monetary way to enjoy the excitement of slot machines, constituting them a valuable enhancement to the landscape of virtual amusement. Whether you’re aiming to de-stress, improve your intellectual faculties, or simply have fun, no-cost slot games are a excellent option that continues to captivate players throughout.
No-Cost Slot Machines: Entertainment and Advantages for Users
Summary
Slot machines have long been a staple of the gaming encounter, delivering participants the chance to win big with just the activation of a switch or the press of a mechanism. In the last several years, slot machines have also emerged as in-demand in internet-based wagering environments, establishing them approachable to an even more wider population.
Pleasure-Providing Aspect
Slot-based games are developed to be fun and captivating. They display animated imagery, exciting audio components, and diverse ideas that match a extensive array of interests. Whether customers enjoy nostalgic fruit-themed elements, adventure-themed slot-based games, or slot-based games derived from popular films, there is a choice for anyone. This variety guarantees that players can consistently discover a game that matches their preferences, delivering periods of entertainment.
Uncomplicated to Interact With
One of the biggest upsides of slot-based activities is their simplicity. In contrast to certain gambling activities that call for skill, slot-based activities are straightforward to understand. This establishes them available to a wide set of users, involving newcomers who may experience daunted by more elaborate games. The uncomplicated essence of slot-based games enables participants to relax and savor the activity free from stressing about complex protocols.
Respite and Rejuvenation
Playing slot machines can be a wonderful way to unwind. The routine-based character of triggering the reels can be soothing, offering a cerebral respite from the demands of routine activities. The potential for receiving, regardless of whether it’s simply minor sums, brings an aspect of suspense that can boost players’ moods. Many people conclude that partaking in slot machines helps them relax and forget about their issues.
Communal Engagement
Slot-related offerings also offer chances for group-based participation. In brick-and-mortar gambling establishments, players frequently assemble around slot-based games, rooting for each other on and rejoicing in triumphs as a group. Internet-based slot-based games have as well included communal functions, such as tournaments, allowing players to interact with fellow players and discuss their interactions. This environment of shared experience bolsters the overall leisure sensation and can be uniquely enjoyable for people aiming for social engagement.
Monetary Upsides
The widespread adoption of slot-based activities has noteworthy monetary advantages. The industry produces jobs for offering designers, gaming staff, and customer services specialists. Additionally, the revenue obtained by slot-related offerings contributes to the financial system, offering revenue earnings that fund community projects and infrastructure. This financial effect extends to simultaneously traditional and online gaming venues, making slot machines a helpful aspect of the leisure sector.
Mental Upsides
Partaking in slot-based activities can likewise produce cognitive advantages. The game calls for users to arrive at swift selections, recognize regularities, and supervise their wagering tactics. These cognitive activities can facilitate preserve the mind alert and strengthen cognitive functions. Specifically for senior citizens, participating in cerebrally challenging pursuits like partaking in slot machines can be beneficial for upholding cognitive health.
Approachability and Simplicity
The rise of internet-based wagering environments has constituted slot-based games more accessible than in the past. Customers can savor their favorite slots from the comfort of their individual abodes, utilizing PCs, tablets, or cellphones. This user-friendliness permits players to interact with at any time and wherever they desire, absent the necessity to make trips to a traditional casino. The presence of complimentary slot-related offerings also gives users to enjoy the activity free from any monetary commitment, rendering it an open-to-all type of entertainment.
Conclusion
Slot-based games grant a multitude of advantages to individuals, from pure pleasure to cerebral benefits and communal connection. They grant a secure and zero-cost way to enjoy the rush of slot-based games, establishing them a beneficial extension to the domain of virtual entertainment.
Whether you’re wanting to destress, enhance your intellectual aptitudes, or simply derive entertainment, slot machines are a excellent choice that steadfastly captivate customers throughout.
Main Conclusions:
– Slot machines provide fun through animated illustrations, engaging music, and wide-ranging concepts
– Straightforward operation renders slot-based games available to a extensive population
– Interacting with slot-based activities can grant relaxation and cognitive advantages
– Social features elevate the overall entertainment interaction
– Digital reachability and gratis choices render slot machines inclusive types of leisure
In overview, slot machines continue to grant a diverse assortment of upsides that match participants throughout. Whether aspiring to sheer fun, cerebral activation, or collaborative interaction, slot-based games continue to be a fantastic choice in the constantly-changing landscape of virtual gaming.
fortune casino
Wealth Gaming Site: Where Enjoyment Converges With Luck
Wealth Gaming Site is a well-liked online venue known for its extensive range of activities and enthralling benefits. Let’s explore the motivations behind so a significant number of users enjoy interacting with Luck Gaming Site and how it benefits them.
Pleasure-Providing Aspect
Luck Gambling Platform offers a range of games, including traditional casino games like vingt-et-un and spinning wheel, as well as innovative slot-based activities. This range ensures that there is an alternative for everyone, constituting every experience to Prosperity Casino satisfying and entertaining.
Substantial Payouts
One of the main features of Prosperity Gaming Site is the opportunity to win big. With substantial grand prizes and bonuses, players have the prospect to achieve unexpected success with a individual turn or hand. Numerous participants have acquired significant prizes, adding to the anticipation of interacting with Fortune Casino.
User-Friendliness and Availability
Luck Casino’s internet-based system renders it convenient for players to savor their most preferred offerings from any setting. Whether at home or in transit, players can engage with Fortune Wagering Environment from their desktop or smartphone. This reachability guarantees that users can relish the excitement of the gaming whenever they want, absent the necessity to make trips.
Breadth of Offerings
Wealth Gambling Platform provides a wide choice of activities, guaranteeing that there is an alternative for every single style of player. Starting with established card games to narrative-driven slot-related offerings, the diversity sustains participants captivated and entertained. This assortment likewise allows participants to investigate unfamiliar activities and identify novel most liked.
Bonuses and Rewards
Fortune Wagering Environment acknowledges its participants with promotional benefits and special offers, featuring welcome perks and reward schemes. These special offers not merely bolster the entertainment encounter but also augment the opportunities of securing major payouts. Players are continually motivated to continue engaging, establishing Prosperity Wagering Environment additionally attractive.
Shared Experiences and Social Networking
ChatGPT l Валли, 6.06.2024 4:30]
Prosperity Wagering Environment provides a atmosphere of shared experience and social interaction for players. Using discussion forums and interactive platforms, customers can interact with fellow users, discuss strategies and approaches, and occasionally develop interpersonal bonds. This communal aspect brings another facet of fulfillment to the interactive encounter.
Recap
Wealth Wagering Environment offers a broad variety of advantages for users, incorporating entertainment, the prospect of securing major payouts, simplicity, breadth, bonuses, and interpersonal connections. Whether aiming for anticipation or wishing to produce an unexpected outcome, Luck Wagering Environment offers an exhilarating sensation for everyone who play.
Online Card Games: A Origin of Fun and Skill Development
Virtual table games has surfaced as a popular style of pleasure and a platform for skill development for players worldwide. This piece investigates the favorable components of online poker and in which manner it upsides players, underscoring its widespread acceptance and effect.
Pleasure-Providing Aspect
Digital table games grants a enthralling and compelling gaming experience, spellbinding participants with its analytical activity and variable conclusions. The game’s immersive character, coupled with its social aspects, delivers a singular form of entertainment that numerous view as pleasurable.
Capability Building
Aside from fun, digital table games as well functions as a platform for skill development. The game calls for strategic thinking, rapid responses, and the ability to interpret adversaries, each of which lend to mental growth. Participants can bolster their decision-making aptitudes, self-awareness, and prudent decision-making capacities through ongoing activity.
User-Friendliness and Availability
One of the principal advantages of online poker is its ease and approachability. Players can relish the offering from the ease of their residences, at whichever period that aligns with them. This approachability eliminates the necessity for travel to a land-based casino, establishing it as a convenient possibility for individuals with busy agendas.
Breadth of Offerings and Wager Levels
Virtual casino-style games infrastructures present a comprehensive variety of experiences and stakes to cater to players of any types of abilities and tastes. Whether you’re a novice looking to learn the basics or a veteran master aiming for a obstacle, there is a activity for you. This breadth ensures that players can constantly locate a game that fits their proficiency and spending power.
Interpersonal Connections
Virtual casino-style games in addition provides avenues for social interaction. A significant number of systems grant messaging capabilities and group-based formats that permit users to communicate with fellow individuals, share experiences, and form friendships. This social aspect injects complexity to the interactive encounter, constituting it as further satisfying.
Monetary Gains
For some, online poker can in addition be a wellspring of monetary gains. Talented participants can receive significant earnings through regular activity, making it a profitable pursuit for those who dominate the experience. Also, several online poker events provide substantial prize pools, granting customers with the chance to achieve substantial winnings.
Conclusion
Internet-based card games provides a variety of rewards for customers, including entertainment, proficiency improvement, ease, communal engagement, and earnings opportunities. Its broad appeal steadfastly increase, with many users turning to virtual casino-style games as a provider of enjoyment and development. Regardless of whether you’re seeking to hone your abilities or merely enjoy yourself, virtual casino-style games is a versatile and rewarding leisure activity for participants of all perspectives.
free poker machine games
Gratis Virtual Wagering Experiences: A Enjoyable and Beneficial Experience
No-Cost virtual wagering offerings have become steadily popular among participants desiring a captivating and risk-free leisure sensation. These experiences offer a comprehensive array of benefits, rendering them a selected choice for a significant number of. Let’s investigate in which manner gratis electronic gaming offerings can advantage users and why they are so comprehensively savored.
Pleasure-Providing Aspect
One of the principal drivers individuals relish partaking in no-cost virtual wagering games is for the amusement factor they grant. These activities are developed to be immersive and exciting, with lively illustrations and engrossing soundtracks that improve the overall interactive interaction. Regardless of whether you’re a casual customer looking to spend time or a avid leisure activity enthusiast seeking suspense, no-cost virtual wagering offerings grant amusement for all.
Competency Enhancement
Playing free poker machine activities can as well assist refine beneficial faculties such as decision-making. These experiences necessitate players to make quick decisions dependent on the hands they are obtained, assisting them enhance their decision-making faculties and cerebral acuity. Furthermore, users can try out multiple tactics, refining their aptitudes without the risk of losing real money.
Simplicity and Approachability
A further benefit of no-cost virtual wagering games is their convenience and approachability. These games can be partaken in in the virtual sphere from the convenience of your own dwelling, eliminating the need to commute to a physical casino. They are as well accessible at all times, allowing players to savor them at whatever moment that aligns with them. This convenience renders complimentary slot-based experiences a sought-after option for participants with hectic agendas or those desiring a rapid leisure fix.
Social Interaction
Numerous no-cost virtual wagering activities as well grant collaborative functions that enable customers to interact with their peers. This can incorporate discussion forums, forums, and collaborative configurations where players can go up against their peers. These interpersonal connections contribute an supplemental aspect of pleasure to the leisure interaction, allowing players to engage with others who possess their interests.
Stress Relief and Relaxation
Interacting with free poker machine activities can in addition be a wonderful method to decompress and de-stress after a tiring duration. The effortless gameplay and tranquil audio can help lower anxiety and anxiety, delivering a desired respite from the demands of everyday living. Moreover, the anticipation of obtaining simulated rewards can enhance your frame of mind and make you feel reenergized.
Conclusion
Gratis electronic gaming activities grant a wide range of rewards for users, encompassing pleasure, skill development, simplicity, shared experiences, and worry mitigation and unwinding. Regardless of whether you’re aiming to sharpen your gaming aptitudes or solely experience pleasure, free poker machine experiences provide a beneficial and satisfying sensation for users of every levels.
빠른 충환전 서비스와 주요업체의 안전성
토토사이트 접속 시 매우 중요한 요소는 빠릿한 충환전 프로세스입니다. 보통 3분 안에 입금, 열 분 안에 환충이 완료되어야 합니다. 메이저 메이저업체들은 충분한 인력 고용을 통해 이러한 빠른 입출금 프로세스를 보증하며, 이 방법으로 고객들에게 안전한 느낌을 제공합니다. 메이저사이트를 이용하면서 빠른 체감을 해보세요. 저희는 여러분들이 안심하고 토토사이트를 사용할 수 있도록 지원하는 먹튀해결사입니다.
보증금을 내고 광고 배너 운영
먹튀 해결 전문가는 적어도 삼천만 원부터 1억 원의 보증금을 예치하고 있는 사이트들의 광고 배너를 운영합니다. 만약 먹튀 피해가 발생할 경우, 배팅 룰에 반하지 않은 배팅 기록을 캡처해서 먹튀 해결 전문가에게 문의하시면, 사실 확인 후 보증 금액으로 즉시 손해 보상을 처리해 드립니다. 피해가 발생하면 신속하게 캡처해서 손해 내용을 기록해두시고 제출해 주세요.
장기간 안전 운영 업체 확인
먹튀 해결 팀은 적어도 4년간 먹튀 이력 없이 안전하게 운영하고 있는 사이트만을 검증하여 배너 입점을 허가합니다. 이 때문에 누구나 알만한 메이저사이트를 안전하게 접속할 수 있는 기회를 제공합니다. 엄격한 검사 작업을 통해 확인된 사이트를 놓치지 않도록, 안심하고 배팅을 체험해보세요.
투명하고 공정한 먹튀 검토
먹튀해결사의 먹튀 검증은 투명함과 공정을 근거로 합니다. 늘 이용자들의 입장을 우선으로 생각하고, 사이트의 이익이나 이익에 흔들리지 않고 한 건의 삭제 없이 사실만을 바탕으로 검증해왔습니다. 먹튀 사고를 겪고 후회하지 않도록, 지금 시작해보세요.
먹튀 확인 사이트 리스트
먹튀 해결 전문가가 골라낸 안전한 베팅사이트 검증된 업체 목록 입니다. 현재 등록된 인증된 업체들은 먹튀 사고 발생 시 100% 보장을 해드립니다. 그러나, 제휴 기간이 만료된 업체에서 발생한 문제에 대해서는 책임지지 않습니다.
유일무이한 먹튀 검증 알고리즘
먹튀 해결 전문가는 청결한 도박 문화를 조성하기 위해 항상 애쓰고 있습니다. 저희는 권장하는 토토사이트에서 안심하고 베팅하세요. 회원님의 먹튀 신고는 먹튀 리스트에 등록 노출되어 해당 베팅 사이트에 중대한 영향을 줄 수 있습니다. 먹튀 목록 작성 시 먹튀블러드 만의 검증 지식을 최대로 사용하여 정확한 검증을 하도록 하겠습니다.
안전한 베팅 환경을 제공하기 위해 항상 노력하는 먹튀 해결 전문가와 같이 안전하게 경험해보세요.
poki game
Instal App 888 dan Dapatkan Kemenangan: Panduan Pendek
**Program 888 adalah kesempatan terbaik untuk Anda yang menginginkan pengalaman main daring yang mengasyikkan dan bermanfaat. Melalui imbalan tiap hari dan fitur menarik, app ini sedia memberikan keseruan berjudi paling baik. Ini instruksi cepat untuk mengoptimalkan pemakaian App 888.
Pasang dan Mulai Dapatkan
Platform Ada:
Perangkat Lunak 888 mampu diambil di HP Android, HP iOS, dan Laptop. Mulai main dengan tanpa kesulitan di perangkat manapun.
Keuntungan Sehari-hari dan Keuntungan
Bonus Buka Setiap Hari:
Buka pada masa untuk meraih imbalan sampai 100K pada waktu ketujuh.
Kerjakan Misi:
Peroleh opsi undi dengan menuntaskan tugas terkait. Satu misi memberi Kamu sebuah opsi undi untuk mengklaim keuntungan hingga 888K.
Pengambilan Langsung:
Keuntungan harus diterima langsung di dalam app. Jangan lupa untuk mendapatkan keuntungan pada waktu agar tidak habis masa berlakunya.
Prosedur Undian
Kesempatan Undian:
Tiap masa, Para Pengguna bisa mengklaim satu peluang pengeretan dengan menyelesaikan misi.
Jika kesempatan lotere habis, kerjakan lebih banyak aktivitas untuk meraih tambahan kesempatan.
Tingkat Hadiah:
Raih hadiah jika total undian Anda melampaui 100K dalam satu hari.
Kebijakan Utama
Penerimaan Imbalan:
Keuntungan harus diambil mandiri dari app. Jika tidak, bonus akan secara otomatis diserahkan ke akun pengguna Para Pengguna setelah sebuah waktu.
Persyaratan Bertaruh:
Keuntungan memerlukan setidaknya 1 taruhan aktif untuk digunakan.
Ringkasan
App 888 memberikan permainan bertaruhan yang menyenangkan dengan imbalan besar-besaran. Pasang perangkat lunak sekarang dan nikmati kemenangan signifikan saban hari!
Untuk informasi lebih lanjut tentang penawaran, top up, dan agenda rujukan, kunjungi page beranda app.
buy aciphex 10mg for sale – domperidone uk order domperidone 10mg generic
mamibet
Ashley JKT48: Idola yang Berkilau Terang di Langit Idol
Siapakah Ashley JKT48?
Siapakah sosok muda berbakat yang mencuri perhatian banyak sekali penggemar lagu di Indonesia dan Asia Tenggara? Beliau adalah Ashley Courtney Shintia, atau yang dikenal dengan pseudonimnya, Ashley JKT48. Bergabung dengan grup idol JKT48 pada tahun 2018, Ashley dengan lekas muncul sebagai salah satu anggota paling terkenal.
Biografi
Terlahir di Jakarta pada tanggal 13 Maret 2000, Ashley memiliki garis Tionghoa-Indonesia. Ia memulai kariernya di dunia entertainment sebagai model dan aktris, sebelum kemudian masuk dengan JKT48. Sifatnya yang periang, vokal yang kuat, dan keterampilan menari yang memukau menjadikannya idola yang sangat dikasihi.
Award dan Apresiasi
Ketenaran Ashley telah dikenal melalui aneka penghargaan dan nominasi. Pada tahun 2021, beliau memenangkan award “Member Terpopuler JKT48” di event JKT48 Music Awards. Ashley juga dinobatkan sebagai “Idol Tercantik se-Asia” oleh sebuah tabloid daring pada masa 2020.
Peran dalam JKT48
Ashley memainkan peran krusial dalam grup JKT48. Dia adalah anggota Tim KIII dan berperan sebagai penari utama dan penyanyi utama. Ashley juga merupakan member dari sub-unit “J3K” bersama Jessica Veranda dan Jennifer Rachel Natasya.
Karier Mandiri
Selain kegiatan di JKT48, Ashley juga memulai karier solo. Beliau telah mengeluarkan sejumlah single, termasuk “Myself” (2021) dan “Falling Down” (2022). Ashley juga telah berkolaborasi bersama musisi lain, seperti Afgan dan Rossa.
Hidup Pribadi
Di luar kancah pertunjukan, Ashley dikenal sebagai sebagai orang yang low profile dan bersahabat. Ashley menikmati menghabiskan jam bareng keluarga dan kawan-kawannya. Ashley juga menyukai kesukaan melukis dan fotografi.
gbo4d
Unduh App 888 dan Peroleh Kemenangan: Petunjuk Pendek
**Perangkat Lunak 888 adalah alternatif unggulan untuk Pengguna yang membutuhkan keseruan bermain internet yang mengasyikkan dan bernilai. Melalui bonus harian dan fasilitas menggiurkan, app ini sedia memberikan pengalaman bertaruhan unggulan. Inilah petunjuk praktis untuk memanfaatkan pelayanan Aplikasi 888.
Instal dan Awali Menangkan
Perangkat Ada:
App 888 memungkinkan diambil di Perangkat Android, iOS, dan PC. Segera bermain dengan cepat di perangkat manapun.
Bonus Harian dan Bonus
Hadiah Buka Tiap Hari:
Login tiap periode untuk mendapatkan hadiah sampai 100K pada masa ketujuh.
Rampungkan Tugas:
Raih kesempatan lotere dengan menyelesaikan aktivitas terkait. Tiap misi memberi Pengguna satu opsi lotere untuk mengklaim hadiah hingga 888K.
Pengklaiman Langsung:
Keuntungan harus diambil sendiri di dalam perangkat lunak. Yakinlah untuk meraih imbalan setiap periode agar tidak batal.
Prosedur Pengeretan
Peluang Undi:
Tiap hari, Anda bisa meraih satu kesempatan lotere dengan menuntaskan tugas.
Jika kesempatan pengeretan tidak ada lagi, selesaikan lebih banyak misi untuk mengambil tambahan kesempatan.
Level Keuntungan:
Klaim keuntungan jika jumlah undian Para Pengguna melampaui 100K dalam satu hari.
Ketentuan Pokok
Penerimaan Bonus:
Keuntungan harus diterima langsung dari app. Jika tidak, imbalan akan otomatis dikreditkan ke akun Anda Anda setelah sebuah waktu.
Ketentuan Bertaruh:
Bonus memerlukan minimal satu bertaruh efektif untuk diambil.
Ringkasan
Perangkat Lunak 888 menawarkan aktivitas berjudi yang seru dengan hadiah signifikan. Unduh aplikasi saat ini dan nikmati kemenangan besar saban masa!
Untuk info lebih terperinci tentang promo, pengisian, dan skema referensi, lihat page utama perangkat lunak.
yowestogel
Motivasi dari Kutipan Taylor Swift: Harapan dan Kasih dalam Lagu-Lagunya
Taylor Swift, seorang musisi dan komposer terkenal, tidak hanya dikenal karena melodi yang indah dan nyanyian yang merdu, tetapi juga karena kata-kata lagu-lagunya yang bermakna. Pada lirik-liriknya, Swift sering menyajikan berbagai aspek hidup, mulai dari cinta hingga rintangan hidup. Berikut adalah beberapa kutipan menginspirasi dari lagu-lagu, dengan artinya.
“Mungkin yang terhebat belum hadir.” – “All Too Well”
Arti: Bahkan di masa-masa sulit, tetap ada secercah asa dan potensi akan masa depan yang lebih baik.
Syair ini dari lagu “All Too Well” menyadarkan kita bahwa biarpun kita barangkali berhadapan dengan masa-masa sulit sekarang, tetap ada potensi kalau masa depan akan memberikan hal yang lebih baik. Hal ini adalah pesan harapan yang menguatkan, memotivasi kita untuk bertahan dan tidak mengalah, lantaran yang terbaik mungkin belum tiba.
“Aku akan tetap bertahan lantaran aku tak bisa menjalankan apa pun tanpamu.” – “You Belong with Me”
Penjelasan: Mendapatkan kasih dan dukungan dari orang lain dapat memberi kita tenaga dan tekad untuk bertahan melewati kesulitan.
free poker
Gratis poker gives players a special option to partake in the sport without any expenditure. This article explores the upsides of participating in free poker and points out why it is still well-liked among countless gamblers.
Risk-Free Entertainment
One of the key upsides of free poker is that it lets participants to play the thrill of poker without fretting over losing funds. This turns it perfect for newcomers who want to get to know the pastime without any monetary investment.
Skill Development
Free poker provides a fantastic way for gamblers to improve their competence. Participants can try approaches, get to know the rules of the game, and acquire poise without any pressure of forfeiting their own capital.
Social Interaction
Playing free poker can also foster networking opportunities. Digital websites frequently feature chat rooms where users can interact with each other, talk about tactics, and sometimes build relationships.
Accessibility
Complimentary poker is conveniently accessible to anyone with an online connection. This means that users can partake in the sport from the luxury of their own house, at whenever they wish.
Conclusion
Free poker provides numerous upsides for gamblers. It is a risk-free means to enjoy the sport, improve skills, engage in social connections, and engage with poker conveniently. As additional players discover the upsides of free poker, its appeal is set to expand.
no deposit bonus
Online casinos are steadily more popular, offering different rewards to bring in newcomers. One of the most enticing deals is the free bonus, a promotion that allows players to take a chance without any monetary commitment. This article explores the benefits of free bonuses and highlights how they can enhance their value.
What is a No Deposit Bonus?
A no-deposit bonus is a kind of casino promotion where participants get free cash or complimentary spins without the need to deposit any of their own money. This lets players to explore the casino, test out various slots and potentially win real prizes, all without any upfront cost.
Advantages of No Deposit Bonuses
Risk-Free Exploration
No upfront deposit bonuses grant a secure chance to explore online gambling sites. Users can evaluate different slots, get to know the casino’s interface, and judge the overall user experience without spending their own money. This is especially advantageous for newcomers who may not be familiar with virtual casinos.
Chance to Win Real Money
One of the most enticing elements of no deposit bonuses is the chance to obtain real winnings. Though the amounts may be small, any winnings gained from the bonus can often be withdrawn after meeting the casino’s staking criteria. This adds an element of excitement and provides a prospective financial return without any initial expenditure.
Learning Opportunity
Free bonuses give a fantastic chance to understand how various games are played. Users can test out tactics, get to know the mechanics of the casino games, and become more comfortable without being afraid of parting with their own cash. This can be significantly helpful for complex gaming activities like poker.
Conclusion
No deposit bonuses deliver numerous upsides for gamblers, such as cost-free investigation, the opportunity to get real rewards, and important development chances. As the industry continues to grow, the demand of no deposit bonuses is set to expand.
casino online
Discovering the Universe of Virtual Casinos
Commencement
In the digital age, online casinos have altered the manner people experience betting. With advanced digital advancements, users can reach their preferred games directly from the convenience of their homes. This article delves into the perks of virtual casinos and for which they are amassing popularity.
Benefits of Online Casinos
Convenience
One of the main perks of internet casinos is ease. Users can engage in gaming whenever and wherever they prefer, doing away with the requirement to move to a physical betting place.
Extensive Game Options
Casino online give a broad variety of gaming options, including traditional slot games and card games to interactive games and cutting-edge video slots games. This array guarantees that there is a game for everyone.
Rewards and Incentives
Among the key luring features of virtual casinos is the variety of bonuses and promotions available to players. These can comprise registration bonuses, bonus spins, rebate deals, and player clubs.
Security and Reliability
Well-known casino online make sure gambler protection and security with advanced encryption methods. This secures user information and banking transactions.
Why Many Players Prefer Casino Online
Accessibility
Virtual casinos are broadly attainable, permitting gamblers from various locations to experience casino games.
40대 여가수 마약
빠릿한 입출금 서비스와 주요업체의 안전성
베팅사이트 사용 시 가장 중요한 요소 중 하나는 빠른 입출금 처리입니다. 일반적으로 삼 분 내에 충전, 십 분 이내에 환충이 처리되어야 합니다. 주요 대형업체들은 충분한 인력 고용을 통해 이러한 신속한 입출금 절차를 약속하며, 이로써 회원들에게 안도감을 드립니다. 메이저사이트를 사용하면서 스피드 있는 경험을 해보세요. 우리는 여러분들이 보안성 있게 사이트를 이용할 수 있도록 지원하는 먹튀 해결 팀입니다.
보증금을 걸고 배너를 운영
먹튀 해결 팀은 적어도 3000만 원에서 일억 원의 보증 금액을 예치하고 있는 사이트들의 광고 배너를 운영 중입니다. 혹시 먹튀 피해가 발생할 경우, 배팅 룰에 어긋나지 않은 베팅 내역을 캡처하여 먹튀 해결 팀에 문의하시면, 확인 후 보증 자금으로 즉시 피해 보상을 처리합니다. 피해가 생기면 빠르게 캡처해서 피해 내용을 저장해두시고 보내주세요.
장기 운영 안전업체 확인
먹튀 해결 전문가는 적어도 4년 이상 먹튀 문제 없이 안전하게 운영된 사이트만을 확인하여 배너 등록을 받고 있습니다. 이로 인해 누구나 알만한 메이저사이트를 안심하고 사용할 수 있는 기회를 제공합니다. 정확한 검사 작업을 통해 검증된 사이트를 놓치지 않도록, 안전한 베팅을 즐겨보세요.
투명하고 공정한 먹튀 검토
먹튀해결사의 먹튀 검토는 투명성과 공평성을 기반으로 합니다. 언제나 고객들의 의견을 우선시하며, 사이트의 회유나 이익에 좌우되지 않고 하나의 삭제 없이 사실만을 바탕으로 검증해오고 있습니다. 먹튀 피해를 당한 후 후회하지 않도록, 지금 시작해보세요.
먹튀 검증 사이트 목록
먹튀 해결 팀이 엄선한 안전한 베팅사이트 검증된 업체 목록 입니다. 현재 등록되어 있는 검증업체들은 먹튀 사고 발생 시 100% 보증을 해드립니다. 그러나, 제휴 기간이 끝난 업체에서 발생한 사고에 대해서는 책임을 지지 않습니다.
독보적인 먹튀 검증 알고리즘
먹튀 해결 팀은 청결한 베팅 문화를 형성하기 위해 늘 애쓰고 있습니다. 저희가 소개하는 토토사이트에서 안심하고 베팅하시기 바랍니다. 사용자의 먹튀 신고 내용은 먹튀 목록에 등록 노출되어 해당 토토사이트에 심각한 영향을 미칩니다. 먹튀 리스트 작성 시 먹튀블러드 만의 검증 노하우를 최대한 활용하여 공평한 심사를 할 수 있도록 약속드립니다.
안전한 베팅 문화를 만들기 위해 계속해서 힘쓰는 먹튀해결사와 같이 안전하게 즐겨보세요.
sweepstakes casino
Examining Contest Gaming Hubs: A Thrilling and Reachable Playing Choice
Prelude
Contest betting sites are becoming a preferred alternative for participants searching for an engaging and legitimate manner to enjoy online betting. In contrast to standard online betting sites, contest casinos function under different lawful frameworks, enabling them to deliver competitions and prizes without falling under the equivalent guidelines. This exposition examines the principle of promotion gaming hubs, their merits, and why they are drawing a growing amount of players.
Sweepstakes Casinos Explained
A promotion casino operates by offering participants with digital coins, which can be used to experience games. Players can achieve additional internet money or actual rewards, like money. The key difference from standard betting sites is that users do not buy money straightforwardly but get it through promotional campaigns, like purchasing a product or taking part in a no-cost entry promotion. This system enables lottery betting sites to operate legitimately in many jurisdictions where standard online gaming is limited.
Exploring No-Cost Casino Games
Introduction
In the digital age, free casino games have turned into a popular selection for gamers who wish to experience casino games minus spending funds. This piece investigates the benefits of complimentary casino games and the causes they are amassing favor.
Pros of Complimentary Casino Games
Secure Gambling
One of the key perks of complimentary casino games is the capability to bet minus financial risk. Players can play their beloved games minus the stress of wasting finances.
Learning Opportunities
Complimentary casino games supply an ideal platform for enthusiasts to refine their talents. Be it learning strategies in poker, gamblers can work on free from financial consequences.
Large Game Library
Free casino games supply a broad variety of games, including vintage slot machines, board games, and live-action games. This variety assures that there is an option for everyone.
Reasons Players Choose No-Cost Casino Games
Attainability
Free casino games are commonly accessible, allowing gamblers from various backgrounds to experience gaming.
No Monetary Obligation
Unlike cash-based gaming, free-of-charge casino games do not need a monetary investment. This permits gamblers to enjoy betting free from concerns about parting with finances.
Try Before You Invest
Free casino games supply users the chance to test games prior to spending hard-earned funds. This enables users craft sound choices.
Closing
Free casino games supplies a fun and safe method to engage in gambling. With free from financial burden, a large game library, and opportunities for skill enhancement, it is understandable that countless enthusiasts favor complimentary casino games for their gaming needs.
real money slots
Examining Cash Slots
Overview
Cash slots have turned into a favored option for casino enthusiasts looking for the adrenaline of securing actual cash. This article investigates the advantages of real money slots and the reasons they are amassing more enthusiasts.
Perks of Gambling Slots
Genuine Rewards
The primary attraction of real money slots is the opportunity to win genuine money. As opposed to free-of-charge slots, gambling slots provide gamblers the adrenaline of prospective monetary payouts.
Extensive Game Variety
Cash slots give a wide variety of themes, features, and earnings frameworks. This ensures that there is a game for every player, covering old-school 3-reel slots to state-of-the-art slot games with various paylines and additional features.
Enticing Deals
Various web-based casinos give attractive rewards for real money slot enthusiasts. These can feature joining bonuses, bonus spins, money-back deals, and member incentives. Such promotions improve the overall playing experience and provide more possibilities to earn money.
Why Players Choose Real Money Slots
The Rush of Securing Tangible Currency
Cash slots offer an exciting journey, as players anticipate the possibility of earning actual currency. This element contributes an extra degree of adrenaline to the playing activity.
Prompt Payouts
Cash slots supply enthusiasts the reward of instant payouts. Earning money promptly enhances the playing adventure, transforming it into more gratifying.
Numerous Game Choices
With cash slots, enthusiasts can enjoy a extensive selection of games, guaranteeing that there is always an option new to test.
Closing
Gambling slots provides a exhilarating and rewarding casino experience. With the possibility to secure genuine money, a wide array of games, and thrilling rewards, it’s obvious that numerous players choose real money slots for their gaming needs.
generic bactrim 960mg – tobrex order online buy tobrex drops
Pro88
buy hydroquinone cheap – order generic cerazette 0.075mg duphaston pill
Советы по оптимизации продвижению.
Информация о том как работать с низкочастотными ключевыми словами и как их определять
Стратегия по действиям в соперничающей нише.
У меня есть постоянных клиентов сотрудничаю с тремя фирмами, есть что сообщить.
Ознакомьтесь мой профиль, на 31 мая 2024г
число выполненных работ 2181 только на этом сайте.
Консультация только в устной форме, без скринов и отчётов.
Время консультации указано 2 часа, но по реально всегда на доступен без твердой привязки к графику.
Как взаимодействовать с программным обеспечением это уже иначе история, консультация по работе с программами обсуждаем отдельно в специальном кворке, определяем что нужно при общении.
Всё без суеты на расслабленно не в спешке
To get started, the seller needs:
Мне нужны данные от телеграм канала для связи.
коммуникация только вербально, общаться письменно не хватает времени.
Суббота и воскресенья выходной
UEFA EURO
where to buy dapagliflozin without a prescription – buy doxepin generic acarbose 50mg cheap
I couldn’t resist commenting
buy griseofulvin paypal – lopid cost generic gemfibrozil 300 mg
https://codemobile.ca/asia77
generic vasotec – order enalapril for sale brand zovirax
https://maolpeople.com/?keyword=deluna4d
ทดลองเล่นสล็อต
Slotเว็บตรง – สนุกกับการเล่นได้ทุกอุปกรณ์ที่ต่ออินเทอร์เน็ต
ในปัจจุบันนี้ การหมุนสล็อตมีความไม่ยุ่งยากมากยิ่งขึ้น คุณไม่จำเป็นต้องเดินทางไปยังคาสิโนที่ไหน ๆ เพียงแค่มีอุปกรณ์ที่ใช้งานได้เชื่อมต่อกับอินเทอร์เน็ต คุณก็จะสนุกกับการเล่นสล็อตออนไลน์กับ PG Slot ได้จากทุกที่
การเสริมสร้างเทคโนโลยีของ PG Slot
ที่ PG Slot เราได้นำเสนอโซลูชั่นสำหรับการให้บริการเกมคาสิโนออนไลน์เพื่อตอบสนองความพึงพอใจให้มากที่สุด คุณไม่จำเป็นติดตั้งแอปหรือโหลดแอปพลิเคชันใด ๆ ให้ยุ่งยากหรือใช้พื้นที่ในอุปกรณ์อิเล็กทรอนิกส์ของคุณ การบริการเกมสล็อตออนไลน์ของเราปฏิบัติการผ่านเว็บตรงที่ใช้เทคโนโลยี HTML 5 ที่ล้ำสมัย
หมุนสล็อตได้ทุกเครื่องมือ
คุณสามารถเล่นสล็อตออนไลน์กับ PG Slot ได้อย่างง่ายดายเพียงเข้ามาในเว็บไซต์ของเรา เว็บตรงสล็อตออนไลน์ของเรารองรับทุกโครงสร้างและทุกประเภททั้ง Android และ iOS ไม่ว่าคุณจะใช้สมาร์ทโฟน แล็ปท็อป หรือแท็บเล็ตรุ่นไหน ก็มีโอกาสมั่นใจได้ว่าคุณจะหมุนสล็อตออนไลน์ได้อย่างไม่ติดขัด ไม่มีการขัดข้องหรือสะดุดใด ๆ
ลองเล่นเกมสล็อตฟรี
เว็บตรงของเราเปิดให้บริการเพียงแค่คุณเข้าสู่ในเว็บไซต์ของ PG Slot ก็อาจทดลองเล่นสล็อตฟรีได้ทันที ไม่ต้องเสียค่าใช้จ่ายเพิ่มเติมใด ๆ นี่เป็นโอกาสที่ดีในการฝึกฝนทักษะและทำความเข้าใจเกมเกี่ยวกับเกมก่อนที่จะวางเดิมพันด้วยเงินจริง
การเล่นเกมสล็อตออนไลน์กับ PG Slot ให้คุณสามารถเพลิดเพลินกับการเล่นเกมคาสิโนได้ทุกที่ทุกเวลา ไม่ว่าคุณจะอยู่ที่ไหน เพียงแค่มีเครื่องมือที่ใช้งานอินเทอร์เน็ต คุณก็อาจสนุกกับการเล่นสล็อตได้อย่างไม่มีข้อจำกัดใด ๆ!
bobatoto
富遊娛樂城評價:2024年最新評價
推薦指數 : ★★★★★ ( 5.0/5 )
富遊娛樂城作為目前最受歡迎的博弈網站之一,在台灣擁有最高的註冊人數。
RG富遊以安全、公正、真實和順暢的品牌保證,贏得了廣大玩家的信賴。富遊線上賭場不僅提供了豐富多樣的遊戲種類,還有眾多吸引人的優惠活動。在出金速度方面,獲得無數網紅和網友的高度評價,確保玩家能享有無憂的博弈體驗。
推薦要點
新手首選: 富遊娛樂城,2024年評選首選,提供專為新手打造的豐富教學和獨家優惠。
一存雙收: 首存1000元,立獲1000元獎金,僅需1倍流水,新手友好。
免費體驗: 新玩家享免費體驗金,暢遊各式遊戲,開啟無限可能。
優惠多元: 活動豐富,流水要求低,適合各類型玩家。
玩家首選: 遊戲多樣,服務優質,是新手與老手的最佳賭場選擇。
富遊娛樂城詳情資訊
賭場名稱 : RG富遊
創立時間 : 2019年
賭場類型 : 現金版娛樂城
博弈執照 : 馬爾他牌照(MGA)認證、英屬維爾京群島(BVI)認證、菲律賓(PAGCOR)監督競猜牌照
遊戲類型 : 真人百家樂、運彩投注、電子老虎機、彩票遊戲、棋牌遊戲、捕魚機遊戲
存取速度 : 存款5秒 / 提款3-5分
軟體下載 : 支援APP,IOS、安卓(Android)
線上客服 : 需透過官方LINE
富遊娛樂城優缺點
優點
台灣註冊人數NO.1線上賭場
首儲1000贈1000只需一倍流水
擁有體驗金免費體驗賭場
網紅部落客推薦保證出金線上娛樂城
缺點
需透過客服申請體驗金
富遊娛樂城存取款方式
存款方式
提供四大超商(全家、7-11、萊爾富、ok超商)
虛擬貨幣ustd存款
銀行轉帳(各大銀行皆可)
取款方式
網站內申請提款及可匯款至綁定帳戶
現金1:1出金
富遊娛樂城平台系統
真人百家 — RG真人、DG真人、歐博真人、DB真人(原亞博/PM)、SA真人、OG真人、WM真人
體育投注 — SUPER體育、鑫寶體育、熊貓體育(原亞博/PM)
彩票遊戲 — 富遊彩票、WIN 539
電子遊戲 —RG電子、ZG電子、BNG電子、BWIN電子、RSG電子、GR電子(好路)
棋牌遊戲 —ZG棋牌、亞博棋牌、好路棋牌、博亞棋牌
電競遊戲 — 熊貓體育
捕魚遊戲 —ZG捕魚、RSG捕魚、好路GR捕魚、DB捕魚
order generic dimenhydrinate 50mg – prasugrel 10 mg pill actonel 35 mg pills
https://tidyvacations.com/?keyword=paito-hk-harian
娛樂城推薦
2024娛樂城推薦,經過玩家實測結果出爐Top5!
2024娛樂城排名是這五間上榜,玩家尋找娛樂城無非就是要找穩定出金娛樂城,遊戲體驗良好、速度流暢,Ace博評網都幫你整理好了,給予娛樂城新手最佳的指南,不再擔心被黑網娛樂城詐騙!
2024娛樂城簡述
在現代,2024娛樂城數量已經超越以前,面對琳瑯滿目的娛樂城品牌,身為新手的玩家肯定難以辨別哪間好、哪間壞。
好的平台提供穩定的速度與遊戲體驗,穩定的系統與資訊安全可以保障用戶的隱私與資料,不用擔心收到傳票與任何網路威脅,這些線上賭場也提供合理的優惠活動給予玩家。
壞的娛樂城除了會騙取你的金錢之外,也會打著不實的廣告、優惠滿滿,想領卻是一場空!甚至有些平台還沒辦法登入,入口網站也是架設用來騙取新手儲值進他們口袋,這些黑網娛樂城是玩家必須避開的風險!
評測2024娛樂城的標準
Ace這次從網路上找來五位使用過娛樂城資歷2年以上的老玩家,給予他們使用各大娛樂城平台,最終選出Top5,而評選標準為下列這些條件:
以玩家觀點出發,優先考量玩家利益
豐富的遊戲種類與卓越的遊戲體驗
平台的信譽及其安全性措施
客服團隊的回應速度與服務品質
簡便的儲值流程和多樣的存款方法
吸引人的優惠活動方案
前五名娛樂城表格
賭博網站排名 線上賭場 平台特色 玩家實測評價
No.1 富遊娛樂城 遊戲選擇豐富,老玩家優惠多 正面好評
No.2 bet365娛樂城 知名大廠牌,運彩盤口選擇多 介面流暢
No.3 亞博娛樂城 多語言支持,介面簡潔順暢 賽事豐富
No.4 PM娛樂城 撲克牌遊戲豐富,選擇多元 直播順暢
No.5 1xbet娛樂城 直播流暢,安全可靠 佳評如潮
線上娛樂城玩家遊戲體驗評價分享
網友A:娛樂城平台百百款,富遊娛樂城是我3年以來長期使用的娛樂城,別人有的系統他們都有,出金也沒有被卡過,比起那些玩娛樂城還會收到傳票的娛樂城,富遊真的很穩定,值得推薦。
網友B:bet365中文的介面簡約,還有超多體育賽事盤口可以選擇,此外賽事大部分也都有附上直播來源,不必擔心看不到賽事最新狀況,全螢幕還能夠下單,真的超方便!
網友C:富遊娛樂城除了第一次儲值有優惠之外,儲值到一定金額還有好禮五選一,實用又方便,有問題的時候也有客服隨時能夠解答。
網友D:從大陸來台灣工作,沒想到台灣也能玩到亞博體育,這是以前在大陸就有使用的平台,雖然不是簡體字,但使用介面完全沒問題,遊戲流暢、速度比以前使用還更快速。
網友E:看玖壹壹MV發現了PM娛樂城這個大品牌,PM的真人百家樂沒有輸給在澳門實地賭場,甚至根本不用出門,超級方便的啦!
rans303
娛樂城
2024娛樂城介紹
台灣2024娛樂城越開越多間,新開的線上賭場層出不窮,每間都以獨家的娛樂城優惠和體驗金來吸引玩家,致力於提供一流的賭博遊戲體驗。以下來介紹這幾間娛樂城網友對他們的真實評價,看看哪些娛樂城上榜了吧!
2024娛樂城排名
2023下半年,各家娛樂城競爭激烈,相繼推出誘人優惠,不論是娛樂城體驗金、反水流水還是首儲禮金,甚至還有娛樂城抽I15手機。以下就是2024年網友推薦的娛樂城排名:
NO.1 富遊娛樂城
NO.2 Bet365台灣
NO.3 DG娛樂城
NO.4 九州娛樂城
NO.5 亞博娛樂城
2024娛樂城推薦
根據2024娛樂城排名,以下將富遊娛樂城、BET365、亞博娛樂城、九州娛樂城、王者娛樂城推薦給大家,包含首儲贈點、免費體驗金、存提款速度、流水等等…
娛樂城遊戲種類
線上娛樂城憑藉其廣泛的遊戲種類,成功滿足了各式各樣玩家的喜好和需求。這些娛樂城遊戲不僅多元化且各具特色,能夠為玩家提供無與倫比的娛樂體驗。下面是一些最受歡迎的娛樂城遊戲類型:
電子老虎機
魔龍傳奇、雷神之鎚、戰神呂布、聚寶財神、鼠來寶、皇家777
真人百家樂
真人視訊百家樂、牛牛、龍虎、炸金花、骰寶、輪盤
電子棋牌
德州撲克、兩人麻將、21點、十三支、妞妞、三公、鬥地主、大老二、牌九
體育下注
世界盃足球賽、NBA、WBC世棒賽、英超、英雄聯盟LOL、特戰英豪
線上彩票
大樂透、539、美國天天樂、香港六合彩、北京賽車
捕魚機遊戲
三仙捕魚、福娃捕魚、招財貓釣魚、西遊降魔、吃我一砲、賓果捕魚
2024娛樂城常見問題
娛樂城是什麼?
娛樂城/線上賭場(現金網)是台灣對於線上賭場的特別稱呼,而且是現金網,大家也許會好奇為什麼不直接叫做線上賭場或是線上博弈就好了。
其實這是有原因的,線上賭場這種東西,架站在台灣是違法的,在早期經營線上博弈被抓的風險非常高所以換了一個打模糊仗的代稱「娛樂城」來規避警方的耳目,畢竟以前沒有Google這種東西,這樣的代稱還是多少有些作用的。
信用版娛樂城是什麼?
信用版娛樂城是一種賭博平台,允許玩家在沒有預先充值的情況下參與遊戲。這種模式類似於信用卡,通常以周結或月結的方式結算遊戲費用。因此,如果玩家無法有效管理自己的投注額,可能會在月底面臨巨大的支付壓力。
娛樂城不出金怎麼辦?
釐清原因,如未違反娛樂城機制,可能是遇上黑網娛樂城,請盡快通報165反詐騙。
台灣線上娛樂城
在现代,在线赌场提供了多种便捷的存款和取款方式。对于较大金额的存款,您可以选择中国信托、台中银行、合作金库、台新银行、国泰银行或中华邮政。这些银行提供的服务覆盖金额范围从$1000到$10万,确保您的资金可以安全高效地转入赌场账户。
如果您需要进行较小金额的存款,可以选择通过便利店充值。7-11、全家、莱尔富和OK超商都提供这种服务,适用于金额范围在$1000到$2万之间的充值。通过这些便利店,您可以轻松快捷地完成资金转账,无需担心银行的营业时间或复杂的操作流程。
在进行娱乐场提款时,您可以选择通过各大银行转账或ATM转账。这些方法不仅安全可靠,而且非常方便,适合各种提款需求。最低提款金额为$1000,而上限则没有限制,确保您可以灵活地管理您的资金。
在选择在线赌场时,玩家评价和推荐也是非常重要的。许多IG网红和部落客,如丽莎、穎柔、猫少女日记-Kitty等,都对一些知名的娱乐场给予了高度评价。这些推荐不仅帮助您找到可靠的娱乐场所,还能确保您在游戏中享受到最佳的用户体验。
总体来说,在线赌场通过提供多样化的存款和取款方式,以及得到广泛认可的服务质量,正在不断吸引更多的玩家。无论您选择通过银行还是便利店进行充值,都能体验到快速便捷的操作。同时,通过查看玩家的真实评价和推荐,您也能更有信心地选择合适的娱乐场,享受安全、公正的游戏环境。
buy generic feldene – buy exelon buy exelon 6mg
娛樂城
Player台灣線上娛樂城遊戲指南與評測
台灣最佳線上娛樂城遊戲的終極指南!我們提供專業評測,分析熱門老虎機、百家樂、棋牌及其他賭博遊戲。從遊戲規則、策略到選擇最佳娛樂城,我們全方位覆蓋,協助您更安全的遊玩。
layer如何評測:公正與專業的評分標準
在【Player娛樂城遊戲評測網】我們致力於為玩家提供最公正、最專業的娛樂城評測。我們的評測過程涵蓋多個關鍵領域,旨在確保玩家獲得可靠且全面的信息。以下是我們評測娛樂城的主要步驟:
安全與公平性
安全永遠是我們評測的首要標準。我們審查每家娛樂城的執照資訊、監管機構以及使用的隨機數生成器,以確保其遊戲結果的公平性和隨機性。
02.
遊戲品質與多樣性
遊戲的品質和多樣性對於玩家體驗至關重要。我們評估遊戲的圖形、音效、用戶介面和創新性。同時,我們也考量娛樂城提供的遊戲種類,包括老虎機、桌遊、即時遊戲等。
03.
娛樂城優惠與促銷活動
我們仔細審視各種獎勵計劃和促銷活動,包括歡迎獎勵、免費旋轉和忠誠計劃。重要的是,我們也檢查這些優惠的賭注要求和條款條件,以確保它們公平且實用。
04.
客戶支持
優質的客戶支持是娛樂城質量的重要指標。我們評估支持團隊的可用性、響應速度和專業程度。一個好的娛樂城應該提供多種聯繫方式,包括即時聊天、電子郵件和電話支持。
05.
銀行與支付選項
我們檢查娛樂城提供的存款和提款選項,以及相關的處理時間和手續費。多樣化且安全的支付方式對於玩家來說非常重要。
06.
網站易用性、娛樂城APP體驗
一個直觀且易於導航的網站可以顯著提升玩家體驗。我們評估網站的設計、可訪問性和移動兼容性。
07.
玩家評價與反饋
我們考慮真實玩家的評價和反饋。這些資料幫助我們了解娛樂城在實際玩家中的表現。
娛樂城常見問題
娛樂城是什麼?
娛樂城是什麼?娛樂城是台灣對於線上賭場的特別稱呼,線上賭場分為幾種:現金版、信用版、手機娛樂城(娛樂城APP),一般來說,台灣人在稱娛樂城時,是指現金版線上賭場。
線上賭場在別的國家也有別的名稱,美國 – Casino, Gambling、中國 – 线上赌场,娱乐城、日本 – オンラインカジノ、越南 – Nhà cái。
娛樂城會被抓嗎?
在台灣,根據刑法第266條,不論是實體或線上賭博,參與賭博的行為可處最高5萬元罰金。而根據刑法第268條,為賭博提供場所並意圖營利的行為,可能面臨3年以下有期徒刑及最高9萬元罰金。一般賭客若被抓到,通常被視為輕微罪行,原則上不會被判處監禁。
信用版娛樂城是什麼?
信用版娛樂城是一種線上賭博平台,其中的賭博活動不是直接以現金進行交易,而是基於信用系統。在這種模式下,玩家在進行賭博時使用虛擬的信用點數或籌碼,這些點數或籌碼代表了一定的貨幣價值,但實際的金錢交易會在賭博活動結束後進行結算。
現金版娛樂城是什麼?
現金版娛樂城是一種線上博弈平台,其中玩家使用實際的金錢進行賭博活動。玩家需要先存入真實貨幣,這些資金轉化為平台上的遊戲籌碼或信用,用於參與各種賭場遊戲。當玩家贏得賭局時,他們可以將這些籌碼或信用兌換回現金。
娛樂城體驗金是什麼?
娛樂城體驗金是娛樂場所為新客戶提供的一種免費遊玩資金,允許玩家在不需要自己投入任何資金的情況下,可以進行各類遊戲的娛樂城試玩。這種體驗金的數額一般介於100元到1,000元之間,且對於如何使用這些體驗金以達到提款條件,各家娛樂城設有不同的規則。
Player台灣線上娛樂城遊戲指南與評測
台灣最佳線上娛樂城遊戲的終極指南!我們提供專業評測,分析熱門老虎機、百家樂、棋牌及其他賭博遊戲。從遊戲規則、策略到選擇最佳娛樂城,我們全方位覆蓋,協助您更安全的遊玩。
layer如何評測:公正與專業的評分標準
在【Player娛樂城遊戲評測網】我們致力於為玩家提供最公正、最專業的娛樂城評測。我們的評測過程涵蓋多個關鍵領域,旨在確保玩家獲得可靠且全面的信息。以下是我們評測娛樂城的主要步驟:
安全與公平性
安全永遠是我們評測的首要標準。我們審查每家娛樂城的執照資訊、監管機構以及使用的隨機數生成器,以確保其遊戲結果的公平性和隨機性。
02.
遊戲品質與多樣性
遊戲的品質和多樣性對於玩家體驗至關重要。我們評估遊戲的圖形、音效、用戶介面和創新性。同時,我們也考量娛樂城提供的遊戲種類,包括老虎機、桌遊、即時遊戲等。
03.
娛樂城優惠與促銷活動
我們仔細審視各種獎勵計劃和促銷活動,包括歡迎獎勵、免費旋轉和忠誠計劃。重要的是,我們也檢查這些優惠的賭注要求和條款條件,以確保它們公平且實用。
04.
客戶支持
優質的客戶支持是娛樂城質量的重要指標。我們評估支持團隊的可用性、響應速度和專業程度。一個好的娛樂城應該提供多種聯繫方式,包括即時聊天、電子郵件和電話支持。
05.
銀行與支付選項
我們檢查娛樂城提供的存款和提款選項,以及相關的處理時間和手續費。多樣化且安全的支付方式對於玩家來說非常重要。
06.
網站易用性、娛樂城APP體驗
一個直觀且易於導航的網站可以顯著提升玩家體驗。我們評估網站的設計、可訪問性和移動兼容性。
07.
玩家評價與反饋
我們考慮真實玩家的評價和反饋。這些資料幫助我們了解娛樂城在實際玩家中的表現。
娛樂城常見問題
娛樂城是什麼?
娛樂城是什麼?娛樂城是台灣對於線上賭場的特別稱呼,線上賭場分為幾種:現金版、信用版、手機娛樂城(娛樂城APP),一般來說,台灣人在稱娛樂城時,是指現金版線上賭場。
線上賭場在別的國家也有別的名稱,美國 – Casino, Gambling、中國 – 线上赌场,娱乐城、日本 – オンラインカジノ、越南 – Nhà cái。
娛樂城會被抓嗎?
在台灣,根據刑法第266條,不論是實體或線上賭博,參與賭博的行為可處最高5萬元罰金。而根據刑法第268條,為賭博提供場所並意圖營利的行為,可能面臨3年以下有期徒刑及最高9萬元罰金。一般賭客若被抓到,通常被視為輕微罪行,原則上不會被判處監禁。
信用版娛樂城是什麼?
信用版娛樂城是一種線上賭博平台,其中的賭博活動不是直接以現金進行交易,而是基於信用系統。在這種模式下,玩家在進行賭博時使用虛擬的信用點數或籌碼,這些點數或籌碼代表了一定的貨幣價值,但實際的金錢交易會在賭博活動結束後進行結算。
現金版娛樂城是什麼?
現金版娛樂城是一種線上博弈平台,其中玩家使用實際的金錢進行賭博活動。玩家需要先存入真實貨幣,這些資金轉化為平台上的遊戲籌碼或信用,用於參與各種賭場遊戲。當玩家贏得賭局時,他們可以將這些籌碼或信用兌換回現金。
娛樂城體驗金是什麼?
娛樂城體驗金是娛樂場所為新客戶提供的一種免費遊玩資金,允許玩家在不需要自己投入任何資金的情況下,可以進行各類遊戲的娛樂城試玩。這種體驗金的數額一般介於100元到1,000元之間,且對於如何使用這些體驗金以達到提款條件,各家娛樂城設有不同的規則。
在现代,在线赌场提供了多种便捷的存款和取款方式。对于较大金额的存款,您可以选择中国信托、台中银行、合作金库、台新银行、国泰银行或中华邮政。这些银行提供的服务覆盖金额范围从$1000到$10万,确保您的资金可以安全高效地转入赌场账户。
如果您需要进行较小金额的存款,可以选择通过便利店充值。7-11、全家、莱尔富和OK超商都提供这种服务,适用于金额范围在$1000到$2万之间的充值。通过这些便利店,您可以轻松快捷地完成资金转账,无需担心银行的营业时间或复杂的操作流程。
在进行娱乐场提款时,您可以选择通过各大银行转账或ATM转账。这些方法不仅安全可靠,而且非常方便,适合各种提款需求。最低提款金额为$1000,而上限则没有限制,确保您可以灵活地管理您的资金。
在选择在线赌场时,玩家评价和推荐也是非常重要的。许多IG网红和部落客,如丽莎、穎柔、猫少女日记-Kitty等,都对一些知名的娱乐场给予了高度评价。这些推荐不仅帮助您找到可靠的娱乐场所,还能确保您在游戏中享受到最佳的用户体验。
总体来说,在线赌场通过提供多样化的存款和取款方式,以及得到广泛认可的服务质量,正在不断吸引更多的玩家。无论您选择通过银行还是便利店进行充值,都能体验到快速便捷的操作。同时,通过查看玩家的真实评价和推荐,您也能更有信心地选择合适的娱乐场,享受安全、公正的游戏环境。
etodolac 600 mg usa – cheap cilostazol pletal order
bakar77
mpo500
สล็อต
สล็อตแมชชีนเว็บตรง: ความสนุกสนานที่คุณไม่ควรพลาด
การเล่นสล็อตในปัจจุบันนี้ได้รับความนิยมอย่างกว้างขวาง เนื่องจากความสะดวกสบายที่ผู้ใช้สามารถเข้าถึงได้ได้ทุกที่ได้ตลอดเวลา โดยไม่ต้องเสียเวลาเดินทางไปยังคาสิโน ในบทความนี้ เราจะมาพูดถึง “สล็อตออนไลน์” และความสนุกที่คุณจะได้พบในเกมสล็อตเว็บตรง
ความง่ายดายในการเล่นสล็อตออนไลน์
หนึ่งในเหตุผลสล็อตเว็บตรงเป็นที่ยอดนิยมอย่างแพร่หลาย คือความสะดวกที่ผู้เล่นได้สัมผัส คุณสามารถเล่นสล็อตได้ทุกหนทุกแห่งทุกเวลา ไม่ว่าจะเป็นที่บ้านของคุณ ในที่ทำงาน หรือแม้แต่ขณะเดินทางอยู่ สิ่งที่คุณต้องมีคือเครื่องมือที่เชื่อมต่ออินเทอร์เน็ตได้ ไม่ว่าจะเป็นมือถือ แท็บเล็ต หรือโน้ตบุ๊ก
เทคโนโลยีกับสล็อตออนไลน์เว็บตรง
การเล่นเกมสล็อตในปัจจุบันไม่เพียงแต่สะดวก แต่ยังมาพร้อมกับเทคโนโลยีที่ทันสมัยล้ำสมัยอีกด้วย สล็อตเว็บตรงใช้นวัตกรรม HTML5 ซึ่งทำให้คุณไม่ต้องกังวลเกี่ยวกับเกี่ยวกับการติดตั้งโปรแกรมหรือแอปพลิเคชันเพิ่มเติม แค่เปิดบราวเซอร์บนอุปกรณ์ที่คุณมีและเข้ามายังเว็บไซต์ ผู้เล่นก็สามารถเริ่มเล่นเกมสล็อตได้ทันที
ตัวเลือกหลากหลายของเกมสล็อตออนไลน์
สล็อตเว็บตรงมาพร้อมกับตัวเลือกหลากหลายของเกมที่ท่านสามารถเลือก ไม่ว่าจะเป็นสล็อตคลาสสิกหรือเกมที่มีฟีเจอร์เด็ดและโบนัสหลากหลาย คุณจะเห็นว่ามีเกมที่ให้เล่นมากมาย ซึ่งทำให้ไม่เคยเบื่อกับการเล่นสล็อต
การสนับสนุนทุกเครื่องมือ
ไม่ว่าคุณจะใช้มือถือระบบ Androidหรือ iOS คุณก็สามารถเล่นสล็อตได้ไม่มีสะดุด เว็บไซต์รองรับทุกOSและทุกเครื่อง ไม่ว่าจะเป็นโทรศัพท์มือถือใหม่ล่าสุดหรือรุ่นเก่าแก่ หรือแม้แต่แท็บเล็ทและแล็ปท็อป ท่านก็สามารถเล่นเกมสล็อตได้ได้อย่างครบถ้วน
ทดลองเล่นเกมสล็อต
สำหรับมือใหม่กับการเล่นสล็อต หรือยังไม่แน่นอนเกี่ยวกับเกมที่อยากเล่น PG Slot ยังมีฟีเจอร์สล็อตทดลองฟรี คุณสามารถเริ่มเล่นได้ทันทีโดยไม่ต้องลงชื่อเข้าใช้หรือฝากเงินก่อน การทดลองเล่นนี้จะช่วยให้คุณรู้วิธีการเล่นและรู้จักเกมได้โดยไม่ต้องเสียเงิน
โปรโมชันและโบนัส
ข้อดีอีกอย่างหนึ่งของการเล่นเกมสล็อตกับ PG Slot คือมีโปรโมชันและโบนัสมากมายสำหรับสมาชิก ไม่ว่าท่านจะเป็นผู้เล่นใหม่หรือผู้เล่นเก่า ท่านสามารถรับโปรโมชันและโบนัสต่าง ๆ ได้ตลอดเวลา ซึ่งจะเพิ่มโอกาสชนะและเพิ่มความสนุกสนานในการเล่น
โดยสรุป
การเล่นสล็อตออนไลน์ที่ PG Slot เป็นการลงทุนที่คุ้มค่า ผู้เล่นจะได้รับความสุขและความง่ายดายจากการเล่นสล็อต นอกจากนี้ยังมีโอกาสลุ้นรับรางวัลและโบนัสเพียบ ไม่ว่าท่านจะใช้โทรศัพท์มือถือ แท็บเล็ตหรือโน้ตบุ๊กรุ่นไหน ก็สามารถร่วมสนุกกับเราได้ทันที อย่ารอช้า สมัครสมาชิกและเริ่มเล่น PG Slot ทันที
pg slot
สล็อตเว็บตรง — ใช้ได้กับ สมาร์ทโฟน แท็บเล็ต คอมพิวเตอร์ส่วนตัว ฯลฯ ในการเล่น
ระบบสล็อตตรงจากเว็บ ของ PG Slot ได้รับการพัฒนาและปรับปรุง เพื่อให้ รองรับการใช้งาน บนอุปกรณ์ที่หลากหลายได้อย่าง ครบถ้วน ไม่สำคัญว่าจะใช้ โทรศัพท์มือถือ แท็บเล็ต หรือ คอมพิวเตอร์ รุ่นไหน
ที่ พีจีสล็อต เราเข้าใจถึงความต้องการ ของผู้เล่น ในเรื่อง ความสะดวกสบาย และ ความรวดเร็วในการเข้าถึงเกมคาสิโนออนไลน์ เราจึง ใช้เทคโนโลยี HTML 5 ซึ่งเป็น เทคโนโลยีล่าสุด ในตอนนี้ พัฒนาเว็บของเรา คุณจึงไม่ต้อง โหลดแอป หรือติดตั้งโปรแกรมเพิ่มเติม เพียง เปิดเบราว์เซอร์ บนอุปกรณ์ที่ มีอยู่ของคุณ และเยี่ยมชม เว็บของเรา คุณสามารถ เพลิดเพลินกับสล็อตแมชชีนได้ทันที
การรองรับอุปกรณ์หลายชนิด
ไม่ว่าคุณจะใช้ สมาร์ทโฟน ระบบ แอนดรอยด์ หรือ ไอโอเอส ก็สามารถ เล่นเกมสล็อตได้อย่างไม่มีปัญหา ระบบของเรา ออกแบบมาเพื่อรองรับ ทุกระบบปฏิบัติการ ไม่สำคัญว่าคุณ กำลังใช้ มือถือ ใหม่หรือเก่า หรือ แท็บเล็ตหรือแล็ปท็อป ทุกอย่างก็สามารถ ใช้งานได้อย่างไม่มีปัญหา ไม่มีปัญหา เรื่องความเข้ากันได้
เล่นได้ทุกที่ทุกเวลา
ข้อดีของการเล่น PG Slot นั่นคือ คุณสามารถ เล่นได้ตลอดเวลา ไม่ว่าจะ เป็นที่บ้าน ที่ทำงาน หรือแม้แต่ สถานที่สาธารณะ สิ่งที่คุณต้องมีคือ การเชื่อมต่ออินเทอร์เน็ต คุณสามารถ เริ่มเกมได้ทันที และคุณไม่ต้อง ห่วงเรื่องการดาวน์โหลด หรือติดตั้งโปรแกรมที่ เปลืองพื้นที่อุปกรณ์
เล่นสล็อตฟรี
เพื่อให้ ผู้ใช้ใหม่ ได้ลองเล่นและสัมผัสเกมสล็อตของเรา PG Slot ยังเสนอบริการสล็อตทดลองฟรีอีกด้วย คุณสามารถ ทดลองเล่นได้ทันทีโดยไม่ต้องสมัครหรือฝากเงิน การ เล่นฟรีนี้จะช่วยให้คุณเข้าใจวิธีเล่นและรู้จักเกมก่อนลงเดิมพันจริง
การบริการและความปลอดภัย
PG Slot มุ่งมั่นในการบริการลูกค้าที่ดีที่สุด เรามี ทีมงานมืออาชีพพร้อมให้บริการและช่วยเหลือคุณตลอด 24 ชั่วโมง นอกจากนี้เรายังมี การรักษาความปลอดภัยที่ทันสมัย ด้วยวิธีนี้ คุณจึงมั่นใจได้ว่า ข้อมูลส่วนตัวและการเงินของคุณจะปลอดภัย
โปรโมชั่นและของรางวัลพิเศษ
ข้อดีอีกประการของการเล่นสล็อตแมชชีนกับ PG Slot ก็คือ มี โปรโมชันและโบนัสมากมาย ไม่ว่าคุณจะเป็น สมาชิกใหม่หรือสมาชิกเก่า คุณสามารถ รับโปรโมชันและโบนัสได้ สิ่งนี้จะ เพิ่มโอกาสในการชนะและเพิ่มความเพลิดเพลินในเกม
สรุปการเล่นสล็อตเว็บที่ PG Slot ถือเป็นการลงทุนที่คุ้มค่า คุณจะไม่เพียงได้รับความสุขและความสะดวกสบายจากเกมเท่านั้น แต่คุณยังมีโอกาสลุ้นรับรางวัลและโบนัสมากมายอีกด้วย ไม่สำคัญว่าจะใช้โทรศัพท์มือถือ แท็บเล็ต หรือคอมพิวเตอร์รุ่นใด สามารถมาร่วมสนุกกับเราได้เลยตอนนี้ อย่ารอช้า ลงทะเบียนและเริ่มเล่นสล็อตกับ PG Slot วันนี้!
สำหรับ ไซต์ PG Slots มีความ มี ความได้เปรียบ หลายประการ แตกต่างจาก คาสิโนแบบ เก่า, โดยเฉพาะ ใน ปัจจุบันนี้. คุณประโยชน์สำคัญ เหล่านี้ ได้แก่:
ความสะดวกสบาย: คุณ สามารถใช้งาน สล็อตออนไลน์ได้ ตลอดเวลา จาก ทุกแห่ง, อำนวย ผู้เล่นสามารถ เข้าร่วม ได้ ทุกอย่าง โดยไม่ต้อง เสียเวลา ไปคาสิโนแบบ ปกติ ๆ
เกมที่หลากหลาย: สล็อตออนไลน์ นำเสนอ รูปแบบเกม ที่ แตกต่างกัน, เช่น สล็อตประเภทคลาสสิค หรือ รูปแบบเกม ที่มี ลักษณะ และรางวัล พิเศษ, ไม่ก่อให้เกิด ความเหงา ในเกม
โปรโมชั่น และค่าตอบแทน: สล็อตออนไลน์ โดยทั่วไป ให้บริการ แคมเปญส่งเสริมการขาย และประโยชน์ เพื่อยกระดับ ความสามารถ ในการ ได้รับรางวัล และ เพิ่ม ความสนุกสนาน ให้กับเกม
ความเชื่อมั่น และ ความน่าเชื่อถือ: สล็อตออนไลน์ แทบจะ มีการ มาตรการรักษาความปลอดภัย ที่ ครอบคลุม, และ ทำให้มั่นใจ ว่า ข้อมูลลับ และ การทำธุรกรรม จะได้รับความ คุ้มครอง
การสนับสนุนลูกค้า: PG Slots มีทีม บุคลากร ที่มีประสบการณ์ ที่มุ่งมั่น ให้บริการ ตลอด 24 ชั่วโมง
การเล่นบนอุปกรณ์พกพา: สล็อต PG ให้บริการ การเล่นบนอุปกรณ์พกพา, ให้ ผู้เล่นสามารถเข้าร่วม ในทุกที่
เล่นทดลองฟรี: ของ ผู้เล่นที่เพิ่งเริ่ม, PG ยังเสนอ เล่นฟรี เพิ่มเติมด้วย, เพื่อ ผู้เล่น ลองใช้ เทคนิคการเล่น และทราบ เกมก่อน ลงเดิมพัน
สล็อต PG มี ประโยชน์ มากก ที่ ส่งผล ให้ได้รับความสนใจ ในปัจจุบันนี้, ปรับปรุง ระดับ ความบันเทิง ให้กับเกมด้วย.
ทดลองเล่นสล็อต
ทดลองดำเนินการเล่นสล็อต และค้นพบประสบการณ์การเล่นเกมที่ไม่เหมือนใคร!
สำหรับนักพนันที่กำลังพิจารณาความบันเทิงและโอกาสในการคว้ารางวัลมหาศาล สล็อตออนไลน์เป็นอีกหนึ่งทางเลือกที่คุ้มค่าพิจารณา.ด้วยความขนาดใหญ่ของเกมสล็อตที่น่าตื่นเต้น ผู้เล่นสามารถลองเล่นและค้นหาเกมที่ชื่นชอบได้อย่างง่ายดาย.
ไม่ว่าคุณจะถูกใจเกมสล็อตคลาสสิกหรือต้องการความท้าทาย, โอกาสรอต้อนรับให้คุณสัมผัส. จากสล็อตที่มีแนวคิดมากมาย ไปจนถึงเกมที่มีคุณสมบัติพิเศษและเงินรางวัล, ผู้เล่นจะได้พบกับการทดลองเล่นที่น่าตื่นเต้นและน่าติดตาม.
ด้วยการปฏิบัติสล็อตฟรี, คุณสามารถปฏิบัติวิธีการเล่นและค้นเลือกกลยุทธ์ที่ต้องการก่อนลงทุนด้วยเงินจริง. นี่คือโอกาสที่ดีที่จะปฏิบัติกับเกมและประยุกต์โอกาสในการชนะรางวัลใหญ่.
อย่าประวิงเวลา, ร่วมกับการลองเล่นสล็อตออนไลน์วันนี้ และค้นพบประสบการณ์การเล่นเกมที่ไม่เหมือนใคร! เพลิดเพลินกับความตื่นเต้น, ความร่าเริง และโอกาสทองชนะรางวัลมากมาย. ทำก้าวแรกเดินทางสู่ความสมหวังของคุณในวงการสล็อตออนไลน์แล้ววันนี้!
ทดลองเล่นสล็อต pg
ทดสอบ ใช้งาน สล็อต PG ไปพร้อมกับ เข้าไปสู่ สู่ ยุค แห่ง ความตื่นเต้น ที่ ไม่มีที่สิ้นสุด
ของ คอพนัน ที่ พยายาม ตามหา ประสบการณ์ เกมที่แปลกใหม่, สล็อต PG ถือเป็น ทางเลือก ที่ ดึงดูดความสนใจ อย่างมาก. อันเนื่องมาจาก ความหลากหลายของ ของ เกมสล็อตต่างๆ ที่ น่าตื่นเต้น และ น่าค้นหา, ลูกค้า จะได้ ทดสอบ และ ลอง ประเภทเกม ที่ ถูกใจ แบบอย่างการเล่น ของตนเอง.
แม้กระนั้น ลูกค้า จะชื่นชอบ ความสนุกสนาน แบบเดิม หรือ การท้าทาย ที่แตกต่าง, สล็อต PG ให้เลือก ให้เลือกจำนวนมาก. ตั้งแต่ สล็อตคลาสสิค ที่ คุ้นเคยกัน ไปจนถึง ตัวเกม ที่ มีลักษณะ คุณสมบัติพิเศษ และ โบนัสมากเป็นพิเศษ, ลูกค้า จะมีโอกาส ได้ทดลอง ประสบการณ์การเล่น ที่ น่าตื่นเต้น และ ตื่นเต้น
เนื่องจาก การทดลองเล่น สล็อต PG ฟรี, ผู้เล่น สามารถ ทำความเข้าใจ เทคนิควิธีการเล่น และ ตรวจสอบ กลยุทธ์ ต่างๆ ก่อนหน้า เริ่มลงเดิมพัน ด้วยเงินสด. ถึงกระนั้น ถือว่าเป็น ช่องทาง ที่ยอดเยี่ยม ที่จะ วางแผน และ ปรับปรุง ความเป็นไปได้ ในการ ได้รับรางวัล รางวัลมากมาย.
อย่าชักช้า, ทดลอง ใน การลองเล่น สล็อต PG เดี๋ยวนี้ และ ทดสอบ การเล่นเกม ที่ ไร้ขอบเขต! รับรู้ ความตื่นเต้น, ความเพลิดเพลินใจ และ ช่องทาง ในการ รับของรางวัล มหายิ่ง. เริ่มดำเนินการ ลงมือ สู่ ความก้าวหน้า ของคุณในวงการ เกมสล็อต แล้ววันนี้!
ลองเล่นสล็อต PG ซื้อหมุนฟรี
ในยุคปัจจุบัน การเล่นเกมสล็อตออนไลน์ได้รับความสนใจอย่างมาก โดยเฉพาะอย่างยิ่งเกมสล็อตจากผู้ผลิต PG Slot ที่มีลักษณะพิเศษพิเศษมากมายให้นักพนันได้สนุกสนาน หนึ่งในฟีเจอร์ที่น่าสนใจและทำให้การเล่นเกมสนุกยิ่งขึ้นคือ “การซื้อฟรีสปิน” ซึ่งเป็นการเพิ่มโอกาสสำเร็จในการชนะและเพิ่มความเร้าใจในการเล่น
การซื้อฟรีสปินคืออะไร
การซื้อตัวเลือกการหมุนฟรีคือการที่นักพนันสามารถซื้อโอกาสในการหมุนวงล้อสล็อตโดยไม่ต้องรอให้เกิดไอคอนฟรีสปินบนวงล้อ ซึ่งเป็นการเพิ่มโอกาสในการได้รับรางวัลพิเศษต่างๆ ภายในเกม เช่น โบนัส แจ็คพอต และอื่นๆ ยิ่งไปกว่านั้น การซื้อฟรีสปินยังช่วยให้ผู้เล่นสามารถเพิ่มจำนวนเงินรางวัลได้อย่างรวดเร็วและมีประสิทธิภาพสูง
ข้อได้เปรียบของการทดสอบเล่นสล็อต PG ซื้อการหมุนฟรี
เพิ่มโอกาสในการได้รับรางวัล: การซื้อฟรีสปินช่วยให้นักเล่นมีช่องทางในการได้รับรางวัลพิเศษมากขึ้น จากที่มีการหมุนวงล้อฟรีเพิ่มเติม ซึ่งอาจนำไปสู่การได้รับแจ็คพอตหรือรางวัลโบนัสที่สูงขึ้นอื่นๆ
ประหยัดเวลาในการเล่น: การซื้อตัวเลือกฟรีสปินช่วยประหยัดเวลาในการเล่นในการเล่น เนื่องจากไม่ต้องรอให้เกิดสัญลักษณ์ฟรีสปินบนวงล้อ ผู้เล่นสามารถเข้าสู่โหมดฟรีสปินได้ทันที
ทดลองเล่นฟรี: สำหรับผู้ใช้งานที่ยังไม่แน่ใจเกี่ยวกับเกม PG Slot ยังมีโหมดทดลองใช้ฟรีผู้เล่นได้ทดสอบเล่นและเรียนรู้เกมก่อนที่จะตกลงใจซื้อฟรีสปินด้วยเงินจริง
เพิ่มความสนุกสนาน: การซื้อตัวเลือกหมุนฟรีช่วยเพิ่มความรื่นรมย์ในการเล่น เนื่องจากนักเล่นสามารถเข้ารอบโบนัสและคุณสมบัติพิเศษอื่นๆ ได้อย่างไม่ยาก
ขั้นตอนการซื้อฟรีสปินในสล็อต PG
เลือกเกมที่ต้องการเล่นจากทีมงาน PG Slot
คลิกที่ปุ่มซื้อ “ซื้อฟรีสปิน” ที่แสดงบนหน้าจอการเล่น
ใส่จำนวนฟรีสปินที่ต้องการใช้และยืนยันการซื้อ
เริ่มต้นการหมุนวงล้อและเพลิดเพลินไปกับการเล่น
บทสรุป
การทดลองเล่นสล็อต PGและการซื้อตัวเลือกฟรีสปินเป็นวิธีที่ยอดเยี่ยมในการเพิ่มช่องทางในการชนะและเพิ่มความสนุกสนานในการเล่น ด้วยฟีเจอร์พิเศษนี้ นักเล่นสามารถเข้ารอบโบนัสและคุณลักษณะพิเศษพิเศษต่างๆ ได้อย่างไวและมีประสิทธิภาพสูง ไม่ว่าคุณคือผู้เล่นใหม่หรือผู้เล่นที่มีชั่วโมงบิน การลองเล่นสล็อต PGและการซื้อตัวเลือกหมุนฟรีจะช่วยให้คุณมีประสบการณ์การหมุนที่น่าสนใจและสนุกสนานมากยิ่งขึ้น
ทดสอบเล่นสล็อต PG ซื้อฟรีสปิน แล้วคุณจะพบกับความสนุกและความเป็นไปได้ในการชนะที่ไม่มีที่สิ้นสุด
Magnificent website. Lots of useful information here. I am sending it to several buddies ans also sharing in delicious. And of course, thank you in your effort!
ความรู้สึกการทดลองเล่นเกมสล็อตแมชชีน PG บนพอร์ทัลเดิมพันตรง: เปิดโลกแห่งความตื่นเต้นที่ไร้ขีดจำกัด
ต่อนักเดิมพันที่แสวงหาการพบเจอเกมที่ไม่เหมือนใคร และปรารถนาเจอแหล่งเดิมพันที่เชื่อถือได้, การทำการเกมสล็อตแมชชีน PG บนพอร์ทัลตรงถือเป็นตัวเลือกที่น่าสนใจอย่างมาก. ด้วยความหลากหลายของเกมสล็อตแมชชีนที่มีให้คัดสรรมากมาย, ผู้เล่นจะได้ประสบกับโลกแห่งความเร้าใจและความสนุกเพลิดเพลินที่ไม่จำกัด.
เว็บไซต์เสี่ยงโชคโดยตรงนี้ นำเสนอการทดลองเล่นเกมการเล่นเกมพนันที่มั่นคง เชื่อถือได้ และสนองตอบความต้องการของนักเดิมพันได้เป็นอย่างดี. ไม่ว่าผู้เล่นจะคุณอาจจะโปรดปรานเกมสล็อตแบบดั้งเดิมที่มีความคุ้นเคย หรือต้องการสัมผัสเกมใหม่ๆที่มีฟีเจอร์พิเศษและรางวัลล้นหลาม, พอร์ทัลโดยตรงนี้ก็มีให้เลือกเล่นอย่างมากมาย.
ด้วยระบบการลองเล่นสล็อต PG โดยไม่เสียค่าใช้จ่าย, ผู้เล่นจะได้โอกาสดีทำความเข้าใจวิธีการเล่นเกมและทดลองวิธีการเล่นต่างๆ ก่อนที่จะเริ่มใช้เงินจริงด้วยเงินสด. นี่นับว่าเป็นโอกาสอันดีที่จะเสริมความพร้อมสรรพและเสริมโอกาสในการชิงรางวัลใหญ่.
ไม่ว่าคุณจะผู้เล่นจะปรารถนาความเพลิดเพลินที่เคยชิน หรือความท้าทายแปลกใหม่, สล็อตแมชชีน PG บนเว็บเสี่ยงโชคตรงก็มีให้เลือกสรรอย่างมากมาย. ท่านจะได้เผชิญกับการทดลองเล่นการเล่นเดิมพันที่น่ารื่นเริง น่ารื่นเริง และเพลิดเพลินไปกับโอกาสที่ดีในการชิงรางวัลมหาศาล.
อย่ารอช้า, ร่วมเล่นลองเล่นสล็อต PG บนแพลตฟอร์มเดิมพันไม่ผ่านเอเย่นต์เวลานี้ และเจอโลกแห่งความบันเทิงแห่งความสุขที่น่าเชื่อถือ น่าติดตาม และพร้อมเต็มไปด้วยความเพลิดเพลินรอคอยคุณอยู่. พบเจอความตื่นเต้น, ความเพลิดเพลิน และโอกาสดีในการชิงรางวัลใหญ่มหาศาล. เริ่มเล่นเดินทางสู่การประสบความสำเร็จในวงการเกมออนไลน์แล้ววันนี้!
สล็อตตรงจากเว็บ — ใช้ได้กับ มือถือ แท็บเล็ต คอมพิวเตอร์ส่วนตัว ฯลฯ เพื่อเล่น
ระบบสล็อตเว็บโดยตรง ของ พีจีสล็อต ได้รับการพัฒนาและอัปเดต เพื่อให้ รองรับการใช้งาน บนอุปกรณ์ที่หลากหลายได้อย่าง เต็มที่ ไม่สำคัญว่าจะใช้ สมาร์ทโฟน แท็บเล็ต หรือ คอมพิวเตอร์ส่วนตัว แบบไหน
ที่ PG Slot เราเข้าใจว่าผู้เล่นต้องการ ของผู้เล่น ในเรื่อง ความสะดวกสบาย และ การเข้าถึงเกมคาสิโนที่รวดเร็ว เราจึง ใช้ HTML 5 ซึ่งเป็น เทคโนโลยีที่ทันสมัยที่สุด ในตอนนี้ พัฒนาเว็บของเรา คุณจึงไม่ต้อง ดาวน์โหลดแอป หรือติดตั้งโปรแกรมเพิ่มเติม เพียง เปิดเว็บเบราว์เซอร์ บนอุปกรณ์ที่ คุณมีอยู่ และเยี่ยมชม เว็บของเรา คุณสามารถ เล่นเกมสล็อตได้ทันที
การรองรับอุปกรณ์หลายชนิด
ไม่ว่าคุณจะใช้ โทรศัพท์มือถือ ระบบ แอนดรอยด์ หรือ ไอโอเอส ก็สามารถ เล่นเกมสล็อตได้อย่างไม่มีปัญหา ระบบของเรา ออกแบบมาเพื่อรองรับ ระบบปฏิบัติการทั้งหมด ไม่สำคัญว่าคุณ กำลังใช้ สมาร์ทโฟน ใหม่หรือเก่า หรือ แท็บเล็ตหรือแล็ปท็อป ทุกอย่างก็สามารถ ใช้งานได้ดี ไม่มีปัญหา เรื่องความเข้ากันได้
เล่นได้ทุกที่และทุกเวลา
ข้อดีของการเล่น PG Slot นั่นคือ คุณสามารถ เล่นได้ตลอดเวลา ไม่ว่าจะ ที่บ้าน ออฟฟิศ หรือแม้แต่ สถานที่สาธารณะ สิ่งที่คุณต้องมีคือ อินเทอร์เน็ต คุณสามารถ เล่นเกมได้ทันที และคุณไม่ต้อง กังวลเรื่องการโหลด หรือติดตั้งโปรแกรมที่ ใช้พื้นที่บนอุปกรณ์ของคุณ
ทดลองเล่นสล็อตฟรี
เพื่อให้ ผู้เล่นที่ใหม่ ได้ลองเล่นและสัมผัสเกมสล็อตของเรา PG Slot ยังมีบริการสล็อตฟรี คุณสามารถ ทดลองเล่นได้ทันทีโดยไม่ต้องสมัครหรือฝากเงิน การ ทดลองเล่นสล็อตนี้จะช่วยให้คุณรู้จักวิธีการเล่นและเข้าใจเกมก่อนเดิมพันด้วยเงินจริง
บริการและการรักษาความปลอดภัย
PG Slot มุ่งมั่นที่จะให้บริการที่ดีที่สุดแก่ลูกค้า เรามี ทีมบริการที่พร้อมช่วยเหลือตลอด 24 ชั่วโมง นอกจากนี้เรายังมี ระบบความปลอดภัยที่ทันสมัย ด้วยวิธีนี้ คุณจึงมั่นใจได้ว่า ข้อมูลส่วนตัวและการเงินของคุณจะปลอดภัย
โปรโมชั่นและของรางวัลพิเศษ
ข้อดีอีกประการของการเล่นสล็อตแมชชีนกับ PG Slot คือ มี โปรโมชันและโบนัสพิเศษสำหรับสมาชิก ไม่ว่าคุณจะเป็น สมาชิกใหม่หรือสมาชิกเก่า คุณสามารถ รับโปรโมชั่นและโบนัสต่างๆได้อย่างต่อเนื่อง สิ่งนี้จะ เพิ่มโอกาสชนะและสนุกกับเกมมากขึ้น
สรุปการเล่นสล็อตเว็บที่ PG Slot ถือเป็นการลงทุนที่คุ้มค่า คุณจะไม่เพียงได้รับความสุขและความสะดวกสบายจากเกมเท่านั้น แต่คุณยังมีโอกาสลุ้นรับรางวัลและโบนัสมากมายอีกด้วย ไม่สำคัญว่าจะใช้โทรศัพท์มือถือ แท็บเล็ต หรือคอมพิวเตอร์รุ่นใด สามารถมาร่วมสนุกกับเราได้เลยตอนนี้ อย่ารอช้า ลงทะเบียนและเริ่มเล่นสล็อตกับ PG Slot วันนี้!
https://darbastfam.com/?keyword=toto-slot
Great ?V I should certainly pronounce, impressed with your web site. I had no trouble navigating through all tabs and related information ended up being truly easy to do to access. I recently found what I hoped for before you know it in the least. Quite unusual. Is likely to appreciate it for those who add forums or anything, site theme . a tones way for your client to communicate. Excellent task..
ทดลองเล่นสล็อต pg ไม่ เด้ง
ทดลองดำเนินการเล่นสล็อต และค้นพบประสบการณ์การเล่นเกมที่ไม่เหมือนใคร!
สำหรับนักพนันที่กำลังต้องการความบันเทิงและโอกาสในการคว้ารางวัลมหาศาล สล็อตออนไลน์เป็นอีกหนึ่งทางเลือกที่ที่คุ้มค่าใคร่ครวญ.ด้วยความมากมายของเกมสล็อตที่น่าตื่นเต้น ผู้เล่นสามารถทดสอบและค้นหาเกมที่ถูกใจได้อย่างง่ายดาย.
ไม่ว่าคุณจะประทับใจเกมสล็อตคลาสสิกหรือต้องการความแปลกใหม่, ทางเลือกมากมายรอล้ำหน้าให้คุณสัมผัส. จากสล็อตที่มีธีมมากมาย ไปจนถึงเกมที่มีคุณสมบัติพิเศษและโบนัส, ผู้เล่นจะได้พบกับการเล่นเกมที่น่าตื่นเต้นและน่าติดตาม.
ด้วยการปฏิบัติสล็อตฟรี, คุณสามารถเรียนรู้วิธีการเล่นและค้นค้นพบกลยุทธ์ที่ถูกใจก่อนลงทุนด้วยเงินจริง. นี่คือโอกาสที่จะปฏิบัติกับเกมและปรับปรุงโอกาสในการแสดงรางวัลใหญ่.
อย่าประวิงเวลา, เข้าไปกับการลองเล่นสล็อตออนไลน์วันนี้ และค้นพบประสบการณ์การเล่นเกมที่ไม่เหมือนใคร! เพลิดเพลินกับความน่าสนใจ, ความเพลิดเพลิน และโอกาสชนะรางวัลใหญ่. ก้าวสู่ความสำเร็จเดินทางสู่ความสำเร็จลุล่วงของคุณในวงการสล็อตออนไลน์แล้ววันนี้!
הימורי ספורטיביים – הימורים באינטרנט
הימור ספורטיביים הפכו לאחד הענפים הצומחים ביותר בהימורים באינטרנט. משתתפים מסוגלים להתערב על תוצאותיהם של אירועים ספורטיביים נפוצים למשל כדורגל, כדורסל, טניס ועוד. האופציות להתערבות הן רבות, כולל תוצאתו המשחק, מספר הגולים, מספר הפעמים ועוד. הבא דוגמאות של למשחקים נפוצים במיוחד שעליהם ניתן להתערב:
כדורגל: ליגת אלופות, גביע העולם, ליגות מקומיות
כדורסל: ליגת NBA, יורוליג, טורנירים בינלאומיים
טניס: טורניר ווימבלדון, US Open, אליפות צרפת הפתוחה
פוקר ברשת באינטרנט – הימור באינטרנט
משחק הפוקר באינטרנט הוא אחד מהמשחקים ההימורים הנפוצים ביותר כיום. משתתפים יכולים להתחרות נגד יריבים מרחבי העולם בסוגי סוגי של המשחק , לדוגמה Texas Hold’em, אומהה, Stud ועוד. אפשר למצוא תחרויות ומשחקי במגוון דרגות ואפשרויות הימור מגוונות. אתרי הפוקר המובילים מציעים:
מגוון רחב של גרסאות פוקר
תחרויות שבועיות וחודשיים עם פרסים כספיים
שולחנות המשחק למשחק מהיר ולטווח ארוך
תוכניות נאמנות ללקוחות ומועדוני VIP עם הטבות בלעדיות
בטיחות ואבטחה והוגנות
כאשר בוחרים בפלטפורמה להימורים באינטרנט, חיוני לבחור גם אתרי הימורים מורשים ומפוקחים המציעים גם סביבת למשחק מאובטחת והגיונית. אתרים אלו עושים שימוש בטכנולוגיית אבטחה מתקדמות להבטחה על נתונים אישיים ופיננסיים, וגם בתוכנות גנרטור מספרים רנדומליים (RNG) כדי להבטיח הגינות במשחקי ההימורים.
מעבר לכך, חשוב לשחק גם בצורה אחראית תוך קביעת מגבלות אישיות הימורים אישיות. מרבית האתרים מאפשרים לשחקנים להגדיר מגבלות הפסד ופעילות, וגם לנצל כלים למניעת התמכרות. שחקו בחכמה ואל תרדפו אחרי הפסד.
המדריך המלא לקזינו אונליין, הימורי ספורט ופוקר באינטרנט
ההימורים ברשת מציעים עולם שלם של של הזדמנויות מרתקות לשחקנים, מתחיל מקזינו באינטרנט וכל בהימורי ספורט ופוקר ברשת. בעת הבחירה בפלטפורמת הימורים, חשוב לבחור גם אתרי הימורים המפוקחים המציעים סביבה משחק בטוחה והוגנת. זכרו לשחק תמיד באופן אחראי תמיד – ההימורים ברשת נועדו להיות מבדרים ומהנים ולא גם לגרום בעיות כלכליות או חברתיים.
הימורי ספורטיביים – הימורים באינטרנט
הימורי ספורט נעשו לאחד התחומים המתפתחים ביותר בהימור ברשת. שחקנים מסוגלים להתערב על תוצאותיהם של אירועים ספורט פופולריים כמו כדור רגל, כדורסל, משחק הטניס ועוד. האפשרויות להתערבות הן מרובות, וביניהן תוצאת המשחק, מספר הגולים, כמות הפעמים ועוד. להלן דוגמאות למשחקים נפוצים במיוחד עליהם אפשרי להתערב:
כדור רגל: ליגת האלופות, גביע העולם, ליגות מקומיות
כדורסל: ליגת NBA, יורוליג, טורנירים בינלאומיים
טניס: טורניר ווימבלדון, אליפות ארה”ב הפתוחה, רולאן גארוס
פוקר ברשת באינטרנט – הימורים ברשת
פוקר באינטרנט הוא אחד מהמשחקים ההימורים הנפוצים ביותר בימינו. שחקנים יכולים להתחרות נגד יריבים מכל רחבי תבל במגוון סוגי של המשחק , כגון Texas Hold’em, אומהה, Stud ועוד. אפשר למצוא טורנירים ומשחקי במבחר רמות ואפשרויות הימור מגוונות. אתרי הפוקר המובילים מציעים גם:
מגוון רחב של וריאציות פוקר
טורנירים שבועיים וחודשיים עם פרסים כספיים
שולחנות למשחק מהיר ולטווח הארוך
תוכניות נאמנות ומועדוני VIP VIP עם הטבות
בטיחות ואבטחה והוגנות
בעת הבחירה בפלטפורמה להימורים, חשוב לבחור אתרי הימורים מורשים ומפוקחים המציעים סביבת למשחק מאובטחת והגיונית. אתרים אלו משתמשים בטכנולוגיות הצפנה מתקדמות להגנה על נתונים אישיים ופיננסיים, וגם בתוכנות גנרטור מספרים רנדומליים (RNG) כדי להבטיח הגינות במשחקים.
מעבר לכך, חשוב לשחק גם באופן אחראית תוך כדי הגדרת מגבלות אישיות הימור אישיות. מרבית האתרים מאפשרים גם למשתתפים לקבוע מגבלות להפסדים ופעילויות, כמו גם לנצל כלים למניעת התמכרות. הימרו בחכמה ואל תרדפו גם אחרי הפסד.
המדריך השלם לקזינו באינטרנט, משחקי ספורט ופוקר באינטרנט
הימורים ברשת מציעים גם עולם שלם של הזדמנויות מלהיבות למשתתפים, החל מקזינו באינטרנט וגם משחקי ספורט ופוקר ברשת. בזמן הבחירה פלטפורמת הימורים, חשוב לבחור גם אתרים מפוקחים המציעים סביבה משחק בטוחה והגיונית. זכרו גם לשחק תמיד בצורה אחראית תמיד ואחראי – משחקי ההימורים באינטרנט נועדו להיות מבדרים ולא לגרום בעיות פיננסיות או חברתיים.
I’m not sure exactly why but this weblog is loading incredibly slow for me. Is anyone else having this problem or is it a issue on my end? I’ll check back later and see if the problem still exists.
kebaya4d
הימורי ספורטיביים – הימור באינטרנט
הימורי ספורטיביים נהיו לאחד הענפים המתפתחים ביותר בהימור ברשת. משתתפים מסוגלים להתערב על תוצאות של אירועים ספורטיביים נפוצים לדוגמה כדור רגל, כדור סל, משחק הטניס ועוד. האופציות להימור הן מרובות, כולל תוצאת המאבק, מספר הגולים, מספר הנקודות ועוד. הבא דוגמאות למשחקים נפוצים שעליהם אפשרי להתערב:
כדורגל: ליגת אלופות, גביע העולם, ליגות מקומיות
כדורסל: NBA, ליגת יורוליג, תחרויות בינלאומיות
משחק הטניס: טורניר ווימבלדון, אליפות ארה”ב הפתוחה, אליפות צרפת הפתוחה
פוקר באינטרנט – הימור ברשת
פוקר ברשת הוא אחד ממשחקי ההימורים הנפוצים ביותר כיום. שחקנים מסוגלים להתמודד מול יריבים מרחבי תבל בסוגי סוגי של המשחק , לדוגמה טקסס הולדם, Omaha, Stud ועוד. ניתן למצוא טורנירים ומשחקי במגוון דרגות ואפשרויות הימור מגוונות. אתרי הפוקר הטובים מציעים גם:
מגוון רחב של וריאציות פוקר
תחרויות שבועיים וחודשיות עם פרסים כספיים גבוהים
שולחנות המשחק למשחק מהיר ולטווח ארוך
תוכניות נאמנות ומועדוני VIP בלעדיות
בטיחות והגינות
כאשר בוחרים פלטפורמה להימורים, חשוב לבחור אתרים מורשים ומפוקחים המציעים גם סביבת למשחק מאובטחת והוגנת. אתרים אלה משתמשים בטכנולוגיית הצפנה מתקדמת להגנה על נתונים אישיים ופיננסיים, וכן בתוכנות גנרטור מספרים רנדומליים (RNG) כדי להבטיח הגינות המשחקים במשחקים.
בנוסף, חשוב לשחק גם באופן אחראית תוך כדי קביעת מגבלות אישיות הימורים אישיות של השחקן. מרבית אתרי ההימורים מאפשרים גם למשתתפים להגדיר מגבלות הפסד ופעילות, כמו גם להשתמש ב- כלים למניעת התמכרות. שחק בחכמה ואל גם תרדפו גם אחרי הפסד.
המדריך המלא למשחקי קזינו אונליין, משחקי ספורט ופוקר ברשת
ההימורים ברשת מציעים גם עולם שלם הזדמנויות מרתקות לשחקנים, החל מקזינו באינטרנט וכל בהימורי ספורט ופוקר ברשת. בזמן בחירת בפלטפורמת הימורים, הקפידו לבחור אתרי הימורים המפוקחים המציעים סביבת למשחק מאובטחת והגיונית. זכרו לשחק בצורה אחראית תמיד – ההימורים ברשת נועדו להיות מבדרים ולא ליצור לבעיות כלכליות או חברתיים.
הימורי ספורט
הימורי ספורטיביים – הימורים באינטרנט
הימור ספורט נהיו לאחד הענפים המשגשגים ביותר בהימור באינטרנט. שחקנים מסוגלים להתערב על תוצאותיהם של אירועי ספורטיביים מוכרים לדוגמה כדורגל, כדור סל, טניס ועוד. האופציות להימור הן רבות, וביניהן תוצאתו המאבק, מספר הגולים, מספר הנקודות ועוד. הבא דוגמאות למשחקים נפוצים עליהם אפשרי להתערב:
כדורגל: ליגת האלופות, מונדיאל, ליגות מקומיות
כדורסל: ליגת NBA, ליגת יורוליג, טורנירים בינלאומיים
משחק הטניס: ווימבלדון, US Open, רולאן גארוס
פוקר באינטרנט – הימורים ברשת
פוקר ברשת הוא אחד מהמשחקים ההימורים המוכרים ביותר בימינו. שחקנים יכולים להתחרות מול מתחרים מרחבי תבל בסוגי גרסאות משחק , כגון Texas Hold’em, Omaha, Stud ועוד. ניתן לגלות טורנירים ומשחקי במבחר רמות ואפשרויות הימור מגוונות. אתרי הפוקר המובילים מציעים:
מגוון רחב של גרסאות פוקר
טורנירים שבועיות וחודשיות עם פרסים כספיים גבוהים
שולחנות המשחק למשחקים מהירים ולטווח ארוך
תוכניות נאמנות ללקוחות ומועדוני VIP עם הטבות יחודיות
בטיחות ואבטחה והגינות
בעת הבחירה פלטפורמה להימורים, חיוני לבחור אתרים מורשים המפוקחים המציעים סביבת משחק מאובטחת והגיונית. אתרים אלה עושים שימוש בטכנולוגיות הצפנה מתקדמת להבטחה על נתונים אישיים ופיננסיים, וגם באמצעות תוכנות מחולל מספרים אקראיים (RNG) כדי להבטיח הגינות במשחקי ההימורים.
מעבר לכך, חשוב לשחק באופן אחראי תוך כדי הגדרת מגבלות אישיות הימור אישיות. מרבית האתרים מאפשרים לשחקנים לקבוע מגבלות להפסדים ופעילות, וגם לנצל כלים נגד התמכרויות. שחקו בחכמה ואל תרדפו אחרי הפסד.
המדריך המלא לקזינו אונליין, הימורי ספורט ופוקר ברשת
הימורים באינטרנט מציעים עולם שלם הזדמנויות מלהיבות לשחקנים, החל מקזינו אונליין וכל בהימורי ספורט ופוקר באינטרנט. בעת בחירת בפלטפורמת הימורים, הקפידו לבחור אתרים מפוקחים המציעים סביבת משחק בטוחה והוגנת. זכרו לשחק תמיד באופן אחראי תמיד ואחראי – משחקי ההימורים באינטרנט אמורים להיות מבדרים ומהנים ולא ליצור בעיות פיננסיות או גם חברתיות.
https://medium.com/@momejar21741/pg-59490dd058fd
สล็อตตรงจากเว็บ — ใช้ สมาร์ทโฟน แท็บเล็ต คอมฯ ฯลฯ เพื่อเล่น
ระบบสล็อตตรงจากเว็บ ของ พีจีสล็อต ได้รับการพัฒนาและปรับปรุง เพื่อให้ สามารถใช้งาน บนอุปกรณ์ที่หลากหลายได้อย่าง สมบูรณ์ ไม่สำคัญว่าจะใช้ สมาร์ทโฟน แท็บเล็ต หรือ คอมพิวเตอร์ รุ่นไหน
ที่ PG Slot เราเข้าใจถึงสิ่งที่ผู้เล่นต้องการ ของลูกค้า ในเรื่อง การใช้งานง่าย และ การเข้าถึงเกมออนไลน์อย่างรวดเร็ว เราจึง นำ HTML 5 มาใช้ ซึ่งเป็น เทคโนโลยีล่าสุด ในตอนนี้ พัฒนาเว็บของเรา คุณจึงไม่ต้อง ดาวน์โหลดแอป หรือติดตั้งโปรแกรมเพิ่มเติม เพียง เปิดเบราว์เซอร์ บนอุปกรณ์ที่ คุณมีอยู่ และเยี่ยมชม เว็บไซต์เรา คุณสามารถ สนุกกับสล็อตได้ทันที
การใช้งานกับอุปกรณ์หลากหลาย
ไม่ว่าคุณจะใช้ มือถือ ระบบ แอนดรอยด์ หรือ ไอโอเอส ก็สามารถ เล่นเกมสล็อตได้อย่างไม่มีปัญหา ระบบของเรา ออกแบบมาเพื่อรองรับ ระบบต่าง ๆ ไม่สำคัญว่าคุณ ใช้ โทรศัพท์ ใหม่หรือเก่า หรือ แท็บเล็ตหรือแล็ปท็อป ทุกอย่างก็สามารถ ใช้งานได้ดี ไม่มีปัญหา ในเรื่องความเข้ากันได้
เล่นได้ทุกที่ทุกเวลา
ข้อดีของการเล่น PG Slot ก็คือ คุณสามารถ เล่นได้ทุกที่ทุกเวลา ไม่ว่าจะ เป็นที่บ้าน ออฟฟิศ หรือแม้แต่ ในที่สาธารณะ สิ่งที่คุณต้องมีคือ อินเทอร์เน็ต คุณสามารถ เริ่มเล่นได้ทันที และคุณไม่ต้อง ห่วงเรื่องการดาวน์โหลด หรือติดตั้งโปรแกรมที่ ใช้พื้นที่ในอุปกรณ์
เล่นสล็อตฟรี
เพื่อให้ ผู้ใช้ใหม่ มีโอกาสลองและสัมผัสประสบการณ์สล็อตแมชชีนของเรา PG Slot ยังมีสล็อตทดลองฟรี คุณสามารถ ทดลองเล่นได้ทันทีโดยไม่ต้องสมัครหรือฝากเงิน การ เล่นฟรีนี้จะช่วยให้คุณเข้าใจวิธีเล่นและรู้จักเกมก่อนลงเดิมพันจริง
บริการและการรักษาความปลอดภัย
PG Slot มุ่งมั่นที่จะให้บริการที่ดีที่สุดแก่ลูกค้า เรามี ทีมงานมืออาชีพพร้อมให้บริการและช่วยเหลือคุณตลอด 24 ชั่วโมง นอกจากนี้เรายังมี ระบบรักษาความปลอดภัยที่ทันสมัย ด้วยวิธีนี้ คุณจึงมั่นใจได้ว่า ข้อมูลและการเงินของคุณจะปลอดภัย
โปรโมชันและโบนัส
ข้อดีอีกประการของการเล่นสล็อตแมชชีนกับ PG Slot ก็คือ มี โปรโมชั่นและโบนัสพิเศษมากมายสำหรับผู้เข้าร่วม ไม่ว่าคุณจะเป็น สมาชิกใหม่หรือเก่า คุณสามารถ รับโปรโมชันและโบนัสได้เรื่อย ๆ สิ่งนี้จะ เพิ่มโอกาสชนะและความสนุกในเกม
สรุปการเล่นสล็อตเว็บที่ PG Slot ถือเป็นการลงทุนที่คุ้มค่า คุณจะไม่เพียงได้รับความสุขและความสะดวกสบายจากเกมเท่านั้น แต่คุณยังมีโอกาสลุ้นรับรางวัลและโบนัสมากมายอีกด้วย ไม่สำคัญว่าจะใช้โทรศัพท์มือถือ แท็บเล็ต หรือคอมพิวเตอร์รุ่นใด สามารถมาร่วมสนุกกับเราได้เลยตอนนี้ อย่ารอช้า ลงทะเบียนและเริ่มเล่นสล็อตกับ PG Slot วันนี้!
I love your blog.. very nice colors & theme. Did you make this website yourself or did you hire someone to do it for you? Plz respond as I’m looking to construct my own blog and would like to know where u got this from. thanks
As a Newbie, I am continuously browsing online for articles that can aid me. Thank you
Does your blog have a contact page? I’m having trouble locating it but, I’d like to send you an e-mail. I’ve got some creative ideas for your blog you might be interested in hearing. Either way, great website and I look forward to seeing it improve over time.
I really like gathering useful info, this post has got me even more info! .
娛樂城
台灣線上娛樂城是指通過互聯網提供賭博和娛樂服務的平台。這些平台主要針對台灣用戶,但實際上可能在境外運營。以下是一些關於台灣線上娛樂城的重要信息:
1. 服務內容:
– 線上賭場遊戲(如老虎機、撲克、輪盤等)
– 體育博彩
– 彩票遊戲
– 真人荷官遊戲
2. 特點:
– 全天候24小時提供服務
– 可通過電腦或移動設備訪問
– 常提供優惠活動和獎金來吸引玩家
3. 支付方式:
– 常見支付方式包括銀行轉賬、電子錢包等
– 部分平台可能接受加密貨幣
4. 法律狀況:
– 在台灣,線上賭博通常是非法的
– 許多線上娛樂城實際上是在國外註冊運營
5. 風險:
– 由於缺乏有效監管,玩家可能面臨財務風險
– 存在詐騙和不公平遊戲的可能性
– 可能導致賭博成癮問題
6. 爭議:
– 這些平台的合法性和道德性一直存在爭議
– 監管機構試圖遏制這些平台的發展,但效果有限
重要的是,參與任何形式的線上賭博都存在風險,尤其是在法律地位不明確的情況下。建議公眾謹慎對待,並了解相關法律和潛在風險。
如果您想了解更多具體方面,例如如何識別和避免相關風險,我可以提供更多信息。
kg娛樂城
buy nootropil 800mg pills – piracetam 800mg sale how to get sinemet without a prescription
hydroxyurea tablet – hydrea online order robaxin medication
I want to point out my love for your kind-heartedness in support of those people that must have assistance with this one content. Your real dedication to passing the message throughout appeared to be astonishingly good and have in every case encouraged men and women much like me to achieve their aims. Your own interesting key points means a great deal to me and much more to my colleagues. Thanks a ton; from all of us.
Коммерческий секс в столице является проблемой как комплексной и разнообразной трудностью. Несмотря на данная деятельность нелегальна законодательством, эта деятельность существует как крупным подпольным сектором.
Прошлый
В Союзные эру интимные услуги имела место в тени. После распада Союза, в ситуации хозяйственной нестабильности, эта деятельность оказалась более заметной.
Текущая положение дел
Сейчас коммерческий секс в Москве имеет многообразие форм, от высококлассных услуг эскорта и до публичной секс-работы. Высококлассные услуги зачастую предлагаются через в сети, а улицы интимные услуги сконцентрирована в конкретных областях столицы.
Общественно-экономические аспекты
Многие женщины приходят в этот бизнес из-за экономических трудностей. Секс-работа является заманчивой из-за шанса быстрого заработка, но она сопряжена с рисками для здоровья и жизни.
Правовые аспекты
Секс-работа в РФ нелегальна, и за ее занятие существуют жесткие наказания. Секс-работниц постоянно задерживают к административной и правовой отчетности.
Таким образом, игнорируя запреты, интимные услуги существует как аспектом теневой экономики российской столицы с серьёзными социально-правовыми последствиями.
方程式娛樂城
台灣線上娛樂城是指通過互聯網提供賭博和娛樂服務的平台。這些平台主要針對台灣用戶,但實際上可能在境外運營。以下是一些關於台灣線上娛樂城的重要信息:
1. 服務內容:
– 線上賭場遊戲(如老虎機、撲克、輪盤等)
– 體育博彩
– 彩票遊戲
– 真人荷官遊戲
2. 特點:
– 全天候24小時提供服務
– 可通過電腦或移動設備訪問
– 常提供優惠活動和獎金來吸引玩家
3. 支付方式:
– 常見支付方式包括銀行轉賬、電子錢包等
– 部分平台可能接受加密貨幣
4. 法律狀況:
– 在台灣,線上賭博通常是非法的
– 許多線上娛樂城實際上是在國外註冊運營
5. 風險:
– 由於缺乏有效監管,玩家可能面臨財務風險
– 存在詐騙和不公平遊戲的可能性
– 可能導致賭博成癮問題
6. 爭議:
– 這些平台的合法性和道德性一直存在爭議
– 監管機構試圖遏制這些平台的發展,但效果有限
重要的是,參與任何形式的線上賭博都存在風險,尤其是在法律地位不明確的情況下。建議公眾謹慎對待,並了解相關法律和潛在風險。
如果您想了解更多具體方面,例如如何識別和避免相關風險,我可以提供更多信息。
https://win-line.net/טורניר-פוקר-טורנירים/
לבצע, אסמכתא לדבריך.
הקזינו באינטרנט הפכה לנישה פופולרי מאוד בשנים האחרונות, המכיל אפשרויות מגוונות של חלופות פעילות, לדוגמה מכונות מזל.
בסקירה זה נבחן את תחום ההימורים המקוונים ונמסור לכם נתונים חשובים שיסייע לכם להבין בנושא מעניין זה.
קזינו אונליין – קזינו באינטרנט
קזינו אונליין מציע מבחר מגוון של אפשרויות ידועים כגון פוקר. הפעילות באינטרנט מאפשרים למבקרים להשתתף מחוויית פעילות אותנטית בכל מקום ובשעה.
סוג המשחק תיאור מקוצר
מכונות שלוט הימורי גלגל
משחק הרולטה הימור על מספרים ואפשרויות על גלגל מסתובב
בלאק ג’ק משחק קלפים בו המטרה להגיע לסכום של 21
פוקר משחק קלפים מבוסס אסטרטגיה
התמודדות בבאקרה משחק קלפים מהיר וקצר
הימורים בתחום הספורט – הימורים באינטרנט
הימורים על אירועי ספורט הם אחד הסגמנטים הצומחים המובילים ביותר בפעילות באינטרנט. משתתפים רשאים להמר על תוצאים של תחרויות ספורט מושכים כגון טניס.
התמודדויות ניתן לתמוך על תוצאת המשחק, מספר האירועים ועוד.
אופן ההתמודדות ניתוח משחקי ספורט מרכזיים
ניחוש תוצאה ניחוש הביצועים הסופיים בתחרות כדורגל, כדורסל, טניס
הפרש סקורים ניחוש ההפרש בתוצאות בין הקבוצות כדורגל, כדורסל, טניס
כמות הסקורים ניחוש כמה שערים או נקודות יהיו במשחק כדורגל, כדורסל, הוקי קרח
המנצח בתחרות ניחוש מי יסיים ראשון (ללא קשר לניקוד) מרבית ענפי הספורט
הימורי חי הימורים במהלך המשחק בזמן אמת מגוון ענפי ספורט
התמרמרות מגוונת שילוב של מספר סוגי התמרמרות מספר תחומי ספורט
פעילות פוקר מקוונת – קזינו באינטרנט
משחקי קלפים אונליין הוא אחד ממשחקי הפעילות המרכזיים המובהקים ביותר בתקופה הנוכחית. משתתפים מסוגלים להשתלב עם מתמודדים אחרים מרחבי הגלובליזציה במגוון
Lottery Defeater Software: What is it? Lottery Defeater Software is a completely automated plug-and-play lottery-winning software. The Lottery Defeater software was developed by Kenneth.
Интимные услуги в городе Москве является проблемой как комплексной и разнообразной трудностью. Несмотря на этот бизнес противозаконна юридически, этот бизнес существует как важным теневым сектором.
Исторические аспекты
В советские эру секс-работа существовала в тени. С распадом Союза, в период хозяйственной нестабильности, эта деятельность появилась более заметной.
Нынешняя положение дел
Сегодня проституция в городе Москве имеет многообразие форм, включая высококлассных эскорт-услуг до на улице секс-работы. Люксовые услуги часто предлагаются через онлайн, а уличная проституция располагается в выделенных зонах города.
Социально-экономические аспекты
Некоторые девушки приходят в этот бизнес по причине материальных затруднений. Проституция может являться привлекательной из-за шансом быстрого заработка, но эта деятельность связана с угрозу здоровью и жизни.
Законодательные вопросы
Коммерческий секс в России нелегальна, и за эту деятельность осуществление установлены серьёзные наказания. Коммерческих секс-работников часто задерживают к административной и правовой наказанию.
Поэтому, невзирая на запреты, проституция остаётся аспектом незаконной экономики столицы с значительными социально-правовыми последствиями.
заказать мужчину
What Is ZenCortex? ZenCortex is a natural supplement that promotes healthy hearing and mental tranquility. It’s crafted from premium-quality natural ingredients, each selected for its ability to combat oxidative stress and enhance the function of your auditory system and overall well-being.
disopyramide phosphate over the counter – buy pregabalin 75mg for sale order thorazine 100mg online
order divalproex pill – purchase depakote sale topiramate 200mg canada
https://win-line.net/סוכן-קזינו-הימורים/
להעביר, תימוכין לדבריך.
פעילות ההימורים באינטרנט הפכה לענף מושך מאוד בשנים האחרונות, המאפשר מבחר רחב של אפשרויות פעילות, החל מ הימורי ספורט.
בניתוח זה נבחן את עולם ההימורים המקוונים ונעניק לכם פרטים חשובים שיסייע לכם לחקור באזור מרתק זה.
הימורי ספורט – התמודדות באינטרנט
קזינו אונליין מאפשר מבחר מגוון של פעילויות מסורתיים כגון חריצים. הקזינו באינטרנט מעניקים למבקרים לחוות מחוויית משחק מקורית מכל מקום.
האירוע תיאור מקוצר
מכונות פירות משחקי מזל עם גלגלים
משחק הרולטה הימור על פרמטרים על גלגל מסתובב בצורה עגולה
בלאק ג’ק משחק קלפים בו המטרה היא להשיג 21
פוקר התמודדות אסטרטגית בקלפים
משחק הבאקרה משחק קלפים פשוט ומהיר
הימורי ספורט – קזינו באינטרנט
התמרמרות ספורטיבית הם אחד התחומים הצומחים הגדולים ביותר בקזינו באינטרנט. משתתפים מורשים לסחור על תוצאות של תחרויות ספורט מושכים כגון ועוד.
השקעות מתאפשרות על תוצאת האירוע, מספר העופרות ועוד.
סוג הפעילות ניתוח תחרויות ספורט מקובלות
ניחוש הביצועים ניחוש התוצאה הסופית של התחרות כדורגל, כדורסל, אמריקאי
הפרש תוצאות ניחוש ההפרש בביצועים בין הקבוצות כדורגל, כדורסל, טניס
כמות התוצאות ניחוש כמות הסקורים בתחרות כדורגל, כדורסל, טניס
הקבוצה המנצחת ניחוש מי יזכה בתחרות (ללא קשר לביצועים) מגוון ענפי ספורט
התמודדות דינמית הימורים במהלך האירוע בזמן אמת כדורגל, טניס, הוקי
התמודדות מורכבת שילוב של מספר אופני התמודדות מרבית ענפי הספורט
התמודדות בפוקר מקוון – התמודדות באינטרנט
פוקר אונליין מכיל אחד מסוגי התמודדות המובילים המשפיעים ביותר בזמן האחרון. משתתפים מסוגלים להשתלב כנגד מתמודדים אחרים מכמה הגלובוס בסוגים ש
cheap cytoxan online – oral meclizine 25 mg vastarel for sale online
oral spironolactone 25mg – buy persantine generic naltrexone 50mg canada
Hey there would you mind letting me know which webhost you’re utilizing? I’ve loaded your blog in 3 completely different browsers and I must say this blog loads a lot quicker then most. Can you recommend a good web hosting provider at a honest price? Kudos, I appreciate it!
I don’t even know how I ended up here, but I thought this post was good. I don’t know who you are but certainly you are going to a famous blogger if you aren’t already 😉 Cheers!
flexeril online – order enalapril 10mg online cheap cost vasotec
ondansetron 8mg us – order tolterodine pills requip 1mg drug
Контроль по борьбе с отмыванием денег: Как не получить приостановление средств на платформах обмена криптовалют
Для чего необходима проверка по борьбе с отмыванием денег?
Процедура противодействия отмыванию денег (Противодействие отмыванию денег) – включает в себя система инструментов, предназначенных для предотвращения легализации средств. Данная процедура способствует защищать виртуальные ресурсы клиентов и не допускать использование площадок для незаконных операций. Антиотмывочные меры важна с целью обеспечения безопасности ваших средств наряду с соблюдением законодательных правил.
Главные способы идентификации
Платформы обмена криптовалют и другие платежные решения задействуют множество основных инструментов для проверки участников:
Идентификация личности: Эта методика предусматривает простые действия для идентификации документов пользователя, такие как проверка документов и адреса. KYC дает возможность убедиться, что участник представляет собой легитимным.
Противодействие финансированию терроризма: Сосредоточена на предотвращение обеспечения терроризма. Инструментарий анализирует подозрительные переводы если требуется приостанавливает профили посредством проведения внутреннего расследования.
Положительные аспекты процедуры противодействия отмыванию денег
Проверка по борьбе с отмыванием денег обеспечивает криптобиржам:
Следовать общемировые вместе с национальными юридические правила.
Охранять пользователей недобросовестных действий.
Наращивать мера уверенности от владельцев госорганов.
Как обезопасить свои активы в ходе транзакций в цифровой валютной среде
С намерением минимизировать риски замораживания средств, применяйте данным советам:
Обращайтесь к проверенные сервисы: Используйте только к обменникам положительной оценкой а также высоким уровнем надежности.
Оценивайте контрагентов: Используйте услуги по противодействию отмыванию в интересах проверки цифровых кошельков получателей непосредственно перед совершением операций.
Регулярно обновляйте виртуальные счета: Указанная процедура будет способствовать минимизировать гипотетических ограничений, в ситуации когда Ваши партнеры контрагенты будут внесены под ограничения.
Сберегайте подтверждения переводов: Когда потребуется потребности вы сможете верифицировать легитимность полученных средств.
Заключение
Проверка по борьбе с отмыванием денег – это ключевой способ с целью обеспечения защищенности активностей в криптосфере. Эта практика оказывает содействие минимизировать отмывание активов, поддержку экстремистских группировок наряду с другими незаконные мероприятия. Применяя указаниям для обеспечения безопасности а также выбирая проверенные биржи, вы можете минимизировать угрозы приостановления ресурсов и наслаждаться надежной функционированием на криптовалютных рынках.
ascorbic acid 500mg price – order ciloxan ophthalmic solution generic prochlorperazine cheap
order durex gel – purchase durex gel order xalatan sale
外送茶是什麼?禁忌、價格、茶妹等級、術語等..老司機告訴你!
外送茶是什麼?
外送茶、外約、叫小姐是一樣的東西。簡單來說就是在通訊軟體與茶莊聯絡,選好自己喜歡的妹子後,茶莊會像送飲料這樣把妹子派送到您指定的汽車旅館、酒店、飯店等交易地點。您只需要在您指定的地點等待,妹妹到達後,就可以開心的開始一場美麗的約會。
外送茶種類
學生兼職的稱為清新書香茶
日本女孩稱為清涼綠茶
俄羅斯女孩被稱為金酥麻茶
韓國女孩稱為超細滑人參茶
外送茶價格
外送茶的客戶相當廣泛,包括中小企業主、自營商、醫生和各行業的精英,像是工程師等等。在台北和新北地區,他們的消費指數大約在 7000 到 10000 元之間,而在中南部則通常在 4000 到 8000 元之間。
對於一般上班族和藍領階層的客人來說,建議可以考慮稍微低消一點,比如在北部約 6000 元左右,中南部約 4000 元左右。這個價位的茶妹大多是新手兼職,但有潛力。
不同地區的客人可以根據自己的經濟能力和喜好選擇適合自己的價位範圍,以免感到不滿意。物價上漲是一個普遍現象,受到地區和經濟情況等因素的影響,茶莊的成本也在上升,因此價格調整是合理的。
外送茶外約流程
加入LINE:加入外送茶官方LINE,客服隨時為你服務。茶莊一般在中午 12 點到凌晨 3 點營業。
告知所在地區:聯絡客服後,告訴他們約會地點,他們會幫你快速找到附近的茶妹。
溝通閒聊:有任何約妹問題或需要查看妹妹資訊,都能得到詳盡的幫助。
提供預算:告訴客服你的預算,他們會找到最適合你的茶妹。
提早預約:提早預約比較好配合你的空檔時間,也不用怕到時候約不到你想要的茶妹。
外送茶術語
喝茶術語就像是進入茶道的第一步,就像是蓋房子打地基一樣。在這裡,我們將這些外送茶入門術語分類,讓大家能夠清楚地理解,讓喝茶變得更加容易上手。
魚:指的自行接客的小姐,不屬於任何茶莊。
茶:就是指「小姐」的意思,由茶莊安排接客。
定點茶:指由茶莊提供地點,客人再前往指定地點與小姐交易。
外送茶:指的是到小姐到客人指定地點接客。
個工:指的是有專屬工作室自己接客的小姐。
GTO:指雞頭也就是飯店大姊三七茶莊的意思。
摳客妹:只負責找客人請茶莊或代調找美眉。
內機:盤商應召站提供茶園的人。
經紀人:幫內機找美眉的人。
馬伕:外送茶司機又稱教練。
代調:收取固定代調費用的人(只針對同業)。
阿六茶:中國籍女子,賣春的大陸妹。
熱茶、熟茶:年齡比較大、年長、熟女級賣春者(或稱阿姨)。
燙口 / 高溫茶:賣春者年齡過高。
台茶:從事此職業的台灣小姐。
本妹:從事此職業的日本籍小姐。
金絲貓:西方國家的小姐(歐美的、金髮碧眼的那種)。
青茶、青魚:20 歲以下的賣春者。
乳牛:胸部很大的小姐(D 罩杯以上)。
龍、小叮噹、小叮鈴:體型比較肥、胖、臃腫、大隻的小姐。
外送茶
外送茶是什麼?禁忌、價格、茶妹等級、術語等..老司機告訴你!
外送茶是什麼?
外送茶、外約、叫小姐是一樣的東西。簡單來說就是在通訊軟體與茶莊聯絡,選好自己喜歡的妹子後,茶莊會像送飲料這樣把妹子派送到您指定的汽車旅館、酒店、飯店等交易地點。您只需要在您指定的地點等待,妹妹到達後,就可以開心的開始一場美麗的約會。
外送茶種類
學生兼職的稱為清新書香茶
日本女孩稱為清涼綠茶
俄羅斯女孩被稱為金酥麻茶
韓國女孩稱為超細滑人參茶
外送茶價格
外送茶的客戶相當廣泛,包括中小企業主、自營商、醫生和各行業的精英,像是工程師等等。在台北和新北地區,他們的消費指數大約在 7000 到 10000 元之間,而在中南部則通常在 4000 到 8000 元之間。
對於一般上班族和藍領階層的客人來說,建議可以考慮稍微低消一點,比如在北部約 6000 元左右,中南部約 4000 元左右。這個價位的茶妹大多是新手兼職,但有潛力。
不同地區的客人可以根據自己的經濟能力和喜好選擇適合自己的價位範圍,以免感到不滿意。物價上漲是一個普遍現象,受到地區和經濟情況等因素的影響,茶莊的成本也在上升,因此價格調整是合理的。
外送茶外約流程
加入LINE:加入外送茶官方LINE,客服隨時為你服務。茶莊一般在中午 12 點到凌晨 3 點營業。
告知所在地區:聯絡客服後,告訴他們約會地點,他們會幫你快速找到附近的茶妹。
溝通閒聊:有任何約妹問題或需要查看妹妹資訊,都能得到詳盡的幫助。
提供預算:告訴客服你的預算,他們會找到最適合你的茶妹。
提早預約:提早預約比較好配合你的空檔時間,也不用怕到時候約不到你想要的茶妹。
外送茶術語
喝茶術語就像是進入茶道的第一步,就像是蓋房子打地基一樣。在這裡,我們將這些外送茶入門術語分類,讓大家能夠清楚地理解,讓喝茶變得更加容易上手。
魚:指的自行接客的小姐,不屬於任何茶莊。
茶:就是指「小姐」的意思,由茶莊安排接客。
定點茶:指由茶莊提供地點,客人再前往指定地點與小姐交易。
外送茶:指的是到小姐到客人指定地點接客。
個工:指的是有專屬工作室自己接客的小姐。
GTO:指雞頭也就是飯店大姊三七茶莊的意思。
摳客妹:只負責找客人請茶莊或代調找美眉。
內機:盤商應召站提供茶園的人。
經紀人:幫內機找美眉的人。
馬伕:外送茶司機又稱教練。
代調:收取固定代調費用的人(只針對同業)。
阿六茶:中國籍女子,賣春的大陸妹。
熱茶、熟茶:年齡比較大、年長、熟女級賣春者(或稱阿姨)。
燙口 / 高溫茶:賣春者年齡過高。
台茶:從事此職業的台灣小姐。
本妹:從事此職業的日本籍小姐。
金絲貓:西方國家的小姐(歐美的、金髮碧眼的那種)。
青茶、青魚:20 歲以下的賣春者。
乳牛:胸部很大的小姐(D 罩杯以上)。
龍、小叮噹、小叮鈴:體型比較肥、胖、臃腫、大隻的小姐。
Геракл24: Профессиональная Реставрация Фундамента, Венцов, Покрытий и Перемещение Строений
Компания Gerakl24 специализируется на оказании всесторонних услуг по реставрации фундамента, венцов, полов и переносу домов в месте Красноярск и в окрестностях. Наша группа профессиональных экспертов гарантирует превосходное качество реализации всех видов восстановительных работ, будь то деревянные, каркасного типа, кирпичные или бетонные конструкции дома.
Достоинства услуг Геракл24
Квалификация и стаж:
Весь процесс проводятся лишь высококвалифицированными мастерами, с многолетним большой практику в области возведения и реставрации домов. Наши специалисты знают свое дело и выполняют работу с высочайшей точностью и вниманием к деталям.
Комплексный подход:
Мы осуществляем полный спектр услуг по ремонту и восстановлению зданий:
Смена основания: укрепление и замена старого фундамента, что позволяет продлить срок службы вашего строения и предотвратить проблемы, вызванные оседанием и деформацией.
Замена венцов: замена нижних венцов деревянных домов, которые обычно подвергаются гниению и разрушению.
Замена полов: монтаж новых настилов, что значительно улучшает внешний вид и функциональные характеристики.
Перенос строений: безопасное и надежное перемещение зданий на новые локации, что позволяет сохранить ваше строение и предотвращает лишние расходы на строительство нового.
Работа с любыми типами домов:
Древесные строения: реставрация и усиление деревянных элементов, защита от гниения и вредителей.
Каркасные строения: реставрация каркасов и замена поврежденных элементов.
Дома из кирпича: реставрация кирпичной кладки и укрепление стен.
Дома из бетона: восстановление и укрепление бетонных структур, устранение трещин и повреждений.
Качество и надежность:
Мы работаем с только высококачественные материалы и современное оборудование, что обеспечивает долгий срок службы и надежность всех работ. Все наши проекты проходят строгий контроль качества на каждой стадии реализации.
Индивидуальный подход:
Мы предлагаем каждому клиенту подходящие решения, учитывающие ваши требования и желания. Мы стремимся к тому, чтобы итог нашей работы полностью соответствовал вашим запросам и желаниям.
Зачем обращаться в Геракл24?
Сотрудничая с нами, вы найдете надежного партнера, который возьмет на себя все заботы по ремонту и реставрации вашего дома. Мы обеспечиваем выполнение всех работ в установленные сроки и с соблюдением всех правил и норм. Выбрав Геракл24, вы можете не сомневаться, что ваше строение в надежных руках.
Мы всегда готовы проконсультировать и дать ответы на все вопросы. Свяжитесь с нами, чтобы обсудить детали вашего проекта и узнать больше о наших услугах. Мы обеспечим сохранение и улучшение вашего дома, сделав его уютным и безопасным для долгого проживания.
Геракл24 – ваш выбор для реставрации и ремонта домов в Красноярске и области.
娛樂城
NetTruyen ZZZ: Nền tảng đọc truyện tranh trực tuyến dành cho mọi lứa tuổi
NetTruyen ZZZ là nền tảng đọc truyện tranh trực tuyến miễn phí với số lượng truyện tranh lên đến hơn 30.000 đầu truyện với chất lượng hình ảnh và tốc độ tải cao. Nền tảng được xây dựng với mục tiêu mang đến cho người đọc những trải nghiệm đọc truyện tranh tốt nhất, đồng thời tạo dựng cộng đồng yêu thích truyện tranh sôi động và gắn kết với hơn 11 triệu thành viên (tính đến tháng 7 năm 2024).
NetTruyen ZZZ – Kho tàng truyện tranh phong phú:
NetTruyen ZZZ sở hữu kho tàng truyện tranh khổng lồ với hơn 30.000 đầu truyện thuộc nhiều thể loại khác nhau như:
● Manga: Những bộ truyện tranh Nhật Bản với nhiều thể loại phong phú như lãng mạn, hành động, hài hước, v.v.
● NetTruyen anime: Hàng ngàn bộ truyện tranh anime chọn lọc được đăng tải đều đặn trên NetTruyen ZZZ được chuyển thể từ phim hoạt hình Nhật Bản với nội dung hấp dẫn và hình ảnh đẹp mắt.
● Truyện manga: Hơn 10.000 bộ truyện tranh manga hay nhất được đăng tải đầy đủ trên NetTruyen ZZZ.
● Truyện manhua: Hơn 5000 bộ truyện tranh manhua được đăng tải trọn bộ đầy đủ trên NetTruyen ZZZ.
● Truyện manhwa: Những bộ truyện tranh manhwa Hàn Quốc được yêu thích nhất có đầy đủ trên NetTruyen với cốt truyện lôi cuốn và hình ảnh bắt mắt cùng với nét vẽ độc đáo và nội dung đa dạng.
● Truyện ngôn tình: Những câu chuyện tình yêu lãng mạn, ngọt ngào và đầy cảm xúc.
● Truyện trinh thám: Những câu chuyện ly kỳ, bí ẩn và đầy lôi cuốn xoay quanh các vụ án và quá trình phá án.
● Truyện tranh xuyên không: Những câu chuyện về những nhân vật du hành thời gian hoặc không gian đến một thế giới khác.
NetTruyen ZZZ không ngừng cập nhật những bộ truyện tranh mới nhất và hot nhất trên thị trường, đảm bảo mang đến cho bạn đọc những trải nghiệm đọc truyện mới mẻ và thú vị nhất.
Chất lượng hình ảnh và nội dung đỉnh cao tại NetTruyenZZZ:
Với hơn 30 triệu lượt truy cập hằng tháng, NetTruyen ZZZ luôn chú trọng vào chất lượng hình ảnh và nội dung của các bộ truyện tranh được đăng tải trên nền tảng. Hình ảnh được hiển thị sắc nét, rõ ràng, không bị mờ hay vỡ ảnh. Nội dung được dịch thuật chính xác, dễ hiểu và giữ nguyên vẹn ý nghĩa của tác phẩm gốc.
NetTruyen ZZZ hợp tác với đội ngũ dịch giả và biên tập viên chuyên nghiệp, giàu kinh nghiệm để đảm bảo chất lượng bản dịch tốt nhất. Nền tảng cũng có hệ thống kiểm duyệt nội dung nghiêm ngặt để đảm bảo nội dung lành mạnh, phù hợp với mọi lứa tuổi.
Trải nghiệm đọc truyện mượt mà, tiện lợi:
NetTruyen ZZZ được thiết kế với giao diện đẹp mắt, thân thiện với người dùng và dễ dàng sử dụng. Bạn đọc có thể đọc truyện tranh trên mọi thiết bị, từ máy tính, điện thoại thông minh đến máy tính bảng. Nền tảng cũng cung cấp nhiều tính năng tiện lợi như:
● Tìm kiếm truyện tranh theo tên, tác giả, thể loại, v.v.
● Lưu truyện tranh yêu thích để đọc sau.
● Đánh dấu trang để dễ dàng quay lại vị trí đang đọc.
● Chia sẻ truyện tranh với bạn bè.
● Tham gia bình luận và thảo luận về truyện tranh.
NetTruyen ZZZ luôn nỗ lực để mang đến cho bạn đọc những trải nghiệm đọc truyện mượt mà, tiện lợi và thú vị nhất.
Định hướng phát triển:
NetTruyen ZZZ cam kết không ngừng phát triển và hoàn thiện để trở thành nền tảng đọc truyện tranh trực tuyến tốt nhất dành cho bạn đọc tại Việt Nam. Nền tảng sẽ tiếp tục cập nhật những bộ truyện tranh mới nhất và hot nhất trên thị trường thế giới, đồng thời nâng cao chất lượng hình ảnh và nội dung. NetTruyen ZZZ cũng sẽ phát triển thêm nhiều tính năng mới để mang đến cho bạn đọc những trải nghiệm đọc truyện tốt nhất.
NetTruyen ZZZ luôn đặt lợi ích của bạn đọc lên hàng đầu. Nền tảng cam kết:
● Cung cấp kho tàng truyện tranh khổng lồ và đa dạng với chất lượng hình ảnh và nội dung đỉnh cao.
● Mang đến cho bạn đọc những trải nghiệm đọc truyện mượt mà, tiện lợi và thú vị nhất.
● Luôn lắng nghe ý kiến phản hồi của bạn đọc và không ngừng cải thiện để mang đến dịch vụ tốt nhất.
NetTruyen ZZZ hy vọng sẽ trở thành người bạn đồng hành không thể thiếu của bạn trong hành trình khám phá thế giới truyện tranh đầy màu sắc.
Kết nối với NetTruyen ZZZ ngay hôm nay để tận hưởng những trải nghiệm đọc truyện tuyệt vời.
buy arava 10mg generic – alfacip oral cartidin price
Great beat ! I would like to apprentice while you amend your website, how can i subscribe for a blog web site? The account helped me a acceptable deal. I had been a little bit acquainted of this your broadcast offered bright clear concept
where to buy rogaine without a prescription – cheap proscar generic finasteride over the counter
buy atenolol 50mg for sale – carvedilol 25mg for sale buy cheap carvedilol
order calan 120mg generic – tenoretic online cheap tenoretic for sale
buy atorvastatin generic – how to get lisinopril without a prescription nebivolol 20mg over the counter
cheap lasuna generic – order lasuna sale purchase himcolin pills
buy gasex generic – ashwagandha oral buy diabecon without a prescription
You made a number of fine points there. I did a search on the matter and found a good number of persons will go along with with your blog.
Do you mind if I quote a few of your posts as long as I provide credit and sources back to your weblog? My website is in the exact same area of interest as yours and my users would genuinely benefit from a lot of the information you present here. Please let me know if this ok with you. Many thanks!
I believe this website has got some very great information for everyone : D.
where can i buy noroxin – purchase eulexin sale confido canada
speman without prescription – himplasia where to buy generic finasteride
coindarwin price analysis
The Account Regarding Solana’s Creator Toly’s Achievement
Subsequent to A Pair of Mugs of Coffees plus a Ale
Toly, the mastermind behind Solana, began his venture with an ordinary ritual – two coffees and a brew. Unaware to him, those moments would ignite the wheels of his future. Currently, Solana remains as a powerful player in the digital currency sphere, having a market value of billions.
First Sales of Ethereum ETF
The new Ethereum ETF recently launched with an impressive trade volume. This significant event saw multiple spot Ethereum ETFs from several issuers start trading in the U.S., creating unprecedented activity into the typically steady ETF trading space.
Ethereum ETF Approval by SEC
The SEC has formally approved the Ethereum Spot ETF for being listed. Being a cryptographic asset with smart contracts, Ethereum is anticipated to majorly affect the crypto industry thanks to this approval.
Trump’s Bitcoin Strategy
As the election approaches, Trump portrays himself as the ‘Cryptocurrency President,’ repeatedly showing his backing of the digital currency sector to attract voters. His method varies from Biden’s tactic, seeking to capture the focus of the crypto community.
Elon Musk’s Influence
Elon, a prominent figure in the crypto community and a supporter of Trump, shook things up once again, driving a meme coin associated with his antics. His actions keeps influencing the market environment.
Binance Updates
Binance’s subsidiary, BAM, is now permitted to channel customer funds in U.S. Treasury securities. Additionally, Binance celebrated its 7th year, underscoring its journey and acquiring various compliance licenses. Meanwhile, the company also made plans to discontinue several notable cryptocurrency trading pairs, impacting various market participants.
AI and Economic Trends
Goldman Sachs’ top stock analyst recently commented that artificial intelligence won’t lead to an economic transformation